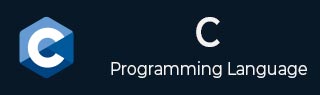
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - raise() function
The C library raise(sig) function causes signal sig to be generated. The sig argument is compatible with the SIG macros.
Two types of Signal Handling −
- Testing Signal Handling: In this signal handling, we use raise() to check whether a signal handler responds correctly to a specific signal.
- Custom Signal Handling: If user want to set the custom signal handling using signal() function then we can use raise() function to call the handler explicitly.
Syntax
Following is the syntax of the C library raise() function −
int raise(int sig)
Parameters
This function accepts only a singal parameter −
sig − This is the signal number to send. Following are few important standard signal constants −
Sr.No. | Macro & Signal |
---|---|
1 |
SIGABRT (Signal Abort) The Abnormal termination which is initiated by the abort function. |
2 |
SIGFPE (Signal Floating-Point Exception) Erroneous arithmetic operation, such as zero divide or an operation resulting in overflow(not necessarily with a floating-point operation). |
3 |
SIGILL (Signal Illegal Instruction) Invalid function image, such as an illegal instruction. This is generally due to a corruption in the code or to an attempt to execute data. |
4 |
SIGINT (Signal Interrupt) Interactive attention signal. Generally generated by the application user. |
5 |
SIGSEGV (Signal Segmentation Violation) Invalid access to storage − When a program tries to read or write outside the memory it is allocated for it. |
6 |
SIGTERM (Signal Terminate) Termination request sent to program. |
Return Value
This function returns zero if it is successful otherwise non-zero.
Example 1
In this example, we use the C library raise() function to see its demonstration.
#include <signal.h> #include <stdio.h> void signal_catchfunc(int); int main () { int ret; ret = signal(SIGINT, signal_catchfunc); if( ret == SIG_ERR) { printf("Error: unable to set signal handler.\n"); exit(0); } printf("Going to raise a signal\n"); ret = raise(SIGINT); if( ret !=0 ) { printf("Error: unable to raise SIGINT signal.\n"); exit(0); } printf("Exiting...\n"); return(0); } void signal_catchfunc(int signal) { printf("!! signal caught !!\n"); }
Output
The above code produces the following result −
Going to raise a signal !! signal caught !! Exiting...
Example 2
In this example, we illustrate the signal reciever and sender using the functions − signal() and raise().
#include <signal.h> #include <stdio.h> void handler(int sig) { // Signal receiver printf("Signal received : %d\n", sig); } int main() { signal(SIGILL, handler); // Signal sender printf("Signal Sended : %d\n", SIGILL); raise(SIGILL); return 0; }
Output
On execution of above code, we get the following result −
Signal Sended : 4 Signal received : 4