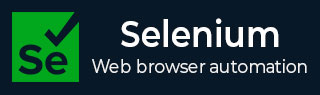
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium with Java Tutorial
Selenium can be used with multiple languages like Java, Python, JavaScript, Ruby, and so on. Selenium is used extensively for web automation testing. Selenium is an open-source and a portable automated software testing tool for testing web applications. It has capabilities to operate across different browsers and operating systems. Selenium is not just a single tool but a set of tools that helps testers to automate web-based applications more efficiently.
How to Setup Selenium with Java?
Step 1 − Download and install Java from the link − https://www.oracle.com/java/technologies/downloads/.
To get a more detailed view on how set up Java, refer to the link − https://www.youtube.com/watch?v=bxIZ1GVWYkQ.
Once we have successfully installed Java, we can confirm its installation by running the command: java, from the command prompt.
C:\java
It will display the following information on the screen −
Usage: java [options] <mainclass> [args...] (to execute a class) or java [options] -jar <jarfile> [args...] (to execute a jar file) or java [options] -m <module>[/<mainclass>] [args...] java [options] --module <module>[/<mainclass>] [args...] (to execute the main class in a module) or java [options] <sourcefile> [args] (to execute a single source-file program) Arguments following the main class, source file, -jar <jarfile>, -m or --module <module>/<mainclass> are passed as the arguments to main class. where options include: -cp <class search path of directories and zip/jar files> -classpath <class search path of directories and zip/jar files> --class-path <class search path of directories and zip/jar files> A ; separated list of directories, JAR archives, and ZIP archives to search for class files. -p <module path> --module-path <module path>... A ; separated list of directories, each directory is a directory of modules. --upgrade-module-path <module path>... A ; separated list of directories, each directory is a directory of modules that replace upgradeable modules in the runtime image --add-modules <module name>[,<module name>...] root modules to resolve in addition to the initial module. <module name> can also be ALL-DEFAULT, ALL-SYSTEM, ALL-MODULE-PATH. --enable-native-access <module name>[,<module name>...] modules that are permitted to perform restricted native operations. <module name> can also be ALL-UNNAMED. --list-modules list observable modules and exit -d <module name> --describe-module <module name> describe a module and exit --dry-run create VM and load main class but do not execute main method. The --dry-run option may be useful for validating the command-line options such as the module system configuration. --validate-modules validate all modules and exit The --validate-modules option may be useful for finding conflicts and other errors with modules on the module path. -D<name>=<value> set a system property -verbose:[class|module|gc|jni] enable verbose output for the given subsystem -version print product version to the error stream and exit --version print product version to the output stream and exit -showversion print product version to the error stream and continue --show-version print product version to the output stream and continue --show-module-resolution show module resolution output during startup -? -h -help print this help message to the error stream --help print this help message to the output stream -X print help on extra options to the error stream --help-extra print help on extra options to the output stream -ea[:<packagename>...|:<classname>] -enableassertions[:<packagename>...|:<classname>] enable assertions with specified granularity -da[:<packagename>...|:<classname>] -disableassertions[:<packagename>...|:<classname>] disable assertions with specified granularity -esa | -enablesystemassertions enable system assertions -dsa | -disablesystemassertions disable system assertions -agentlib:<libname>[=<options>] load native agent library <libname>, e.g. -agentlib:jdwp see also -agentlib:jdwp=help -agentpath:<pathname>[=<options>] load native agent library by full pathname -javaagent:<jarpath>[=<options>] load Java programming language agent, see java.lang.instrument -splash:<imagepath> show splash screen with specified image HiDPI scaled images are automatically supported and used if available. The unscaled image filename, e.g. image.ext, should always be passed as the argument to the -splash option. The most appropriate scaled image provided will be picked up automatically. See the SplashScreen API documentation for more information @argument files one or more argument files containing options --disable-@files prevent further argument file expansion --enable-preview allow classes to depend on preview features of this release To specify an argument for a long option, you can use --<name>=<value> or --<name> <value>.
Step 2 − Next, we would Confirm the version of the Java installed by running the command −
java –version
It will show the following output −
openjdk version "17.0.9" 2023-10-17 OpenJDK Runtime Environment Homebrew (build 17.0.9+0) OpenJDK 64-Bit Server VM Homebrew (build 17.0.9+0, mixed mode, sharing)
The output of the command executed signified that the java version installed in the system is 17.0.9.
Step 3 − Install Maven in our system using the below link −
https://maven.apache.org/download.cgi.
To get a more detailed view on how set up Maven, refer to the link − Maven Environment.
Next, we would confirm the version of the Maven installed by running the following command −
mvn –version.
It will show the following output −
Apache Maven 3.9.6 (bc0240f3c744dd6b6ec2920b3cd08dcc295161ae) Maven home: /opt/homebrew/Cellar/maven/3.9.6/libexec Java version: 21.0.1, vendor: Homebrew, runtime: /opt/homebrew/Cellar/openjdk/21.0.1/libexec/openjdk.jdk/Contents/Home Default locale: en_IN, platform encoding: UTF-8 OS name: "mac os x", version: "14.0", arch: "aarch64", family: "mac"
The output of the command executed signified that the Maven version installed in the system is Apache Maven 3.9.6.
Step 4 − Install any code editor like IntelliJ, Eclipse, and so on to write and run the Selenium test. There are several editors available in the market for example: Eclipse, IntelliJ, Atom, and so on. Using these editors, we can start working on a Java project to start our test automation.
To get a more detailed view on how set up IntelliJ, refer to the link − Selenium IntelliJ.
Step 5 − Add the Selenium Maven dependencies from the below link −
https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java.
Step 6 − Select and click on a version link under the Central tab. Then navigate to the Selenium Java>><version> page. Copy the dependency under the Maven tab.
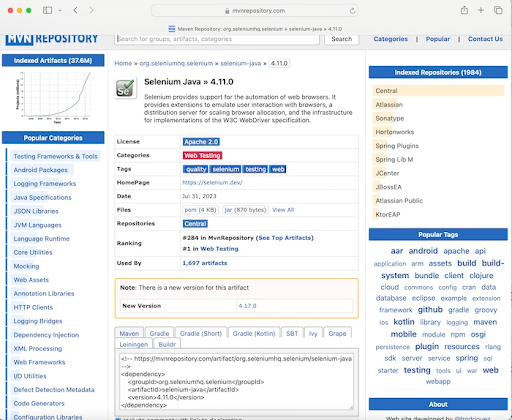
Dependency example −
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency>
Step 7 − Paste the dependency copied in Step6 in the pom.xml file.
Step 8 − Add the below code in the Main.java file.
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class Main { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // URL launch driver.get("https://www.google.com"); // get browser title after browser launch System.out.println("Browser title: " + driver.getTitle()); } }
Overall dependencies added in the pom.xml file −
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> </dependencies> </project>
Step 9 − Right click and select Run ‘Main.main()’ option. Wait till the run is completed.
Step 10 − Chrome browser should be launched, the output in console with the message should be - Browser Title: Google.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
It will show the following output −
Browser title: Google Process finished with exit code 0
Along with that Chrome browser got launched with the message Chrome is being controlled by automated test software at the top.
Launch Browser and Quit Driver with Selenium Java
We can launch the browser and open an application using the driver.get() method, and finally quit the browser with the quit() method.
Code Implementation in MainBrowserQuit.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class MainBrowserQuit { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // URL launch and get the browser title driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); System.out.println( "Browser title after launch: " + driver.getTitle()); // close browser driver.quit(); } }
It will show the following output −
Browser title after launch: Selenium Practice - Student Registration Form Process finished with exit code 0
In the above example, we had first launched the Chrome browser then retrieved the browser title and then quitted the browser, and in the console received the message - Browser title after launch: Selenium Practice - Student Registration Form.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Identify Element and Check Its Functionality Using Selenium Java
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the element. There are some locators available in Selenium for this purpose, they are id, class, class name, name, link text, partial link text, tagname, css, and xpath. These locators need to be used with the findElement() method.
For example, findElement(By.name("<value of name attribute>")) will locate the first web element with the given name attribute value. In case there is no element with the matching value of the name attribute, NoSuchElementException should be thrown.
Let us see the html code of the same input box as discussed before in the below image −
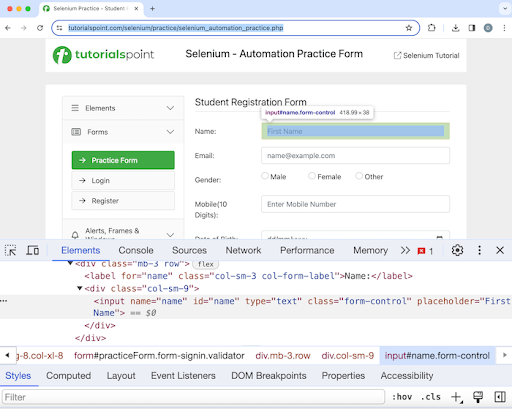
<input name="name" id="name" type="text" class="form-control" placeholder="First Name">
The edit box highlighted in the above image has a name attribute with a value as name. Let us input the text Selenium into this edit box after identifying it.
Code Implementation in LocatorsName.java
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorsName { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // Opening the webpage where we will identify edit box enter text driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the search box with name locator to enter text WebElement i = driver.findElement(By.name("name")); i.sendKeys("Selenium"); // Get the value entered String text = i.getAttribute("value"); System.out.println("Entered text is: " + text); // Closing browser driver.quit(); } }
It will show the following output −
Entered text is: Selenium Process finished with exit code 0
The output shows the message - Process with exit code 0 meaning that the above code executed successfully. Also, the value entered within the edit box (obtained from the getAttribute method) - Selenium got printed in the console.
This concludes our comprehensive take on the tutorial on Selenium - Java Tutorial. We’ve started with describing how to set up Selenium with Java, how to launch a browser and quit a session using the Selenium Java, and how to identify an element and check its functionality using Selenium Java.
This equips you with in-depth knowledge of the Selenium - Java Tutorial. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.