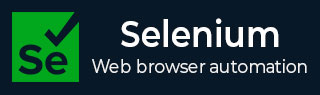
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium with C# Tutorial
Selenium can be used with multiple languages like Java, Python, JavaScript, Ruby, C#, and so on. Selenium is used extensively for web automation testing. Selenium is an open-source and a portable automated software testing tool for testing web applications.
It has capabilities to operate across different browsers and operating systems. Selenium is not just a single tool but a set of tools that helps testers to automate web-based applications more efficiently. C# is another language binding for Selenium.
There are no major differences between the Java and C# language bindings in Selenium. The main difference lies in the language only. Also, there is a difference in the Change log of both of these languages.
In Java, we say use the Webdriver, WebElement but in C# we call it IWebdriver, IWebElement. So the convention which is followed in C# is that, while declaring an interface, the letter ‘I’ is added prior to the name of the Interface.
In Page Factory using Java, the element is identified using the @FindBy annotations, however, while using the Page Factory in C#, it is known as an attribute and denoted by [FindBy] is used to locate elements.
Setup Selenium with C# and Launch a Browser
Step 1 − Install the C# code editor called Visual Studio from the link − https://visualstudio.microsoft.com/downloads/.
Using this editor, we can start working on a C# project to start our test automation.
To get a more detailed view on how to set up the Visual Studio, refer to the link − https://www.tutorialspoint.com/ebook/.
Step 2 − Create a new project, by either right clicking on an existing project, and click on the Add option then click on the New Project, or if no project is opened, click on the File menu, then select the New Project option.
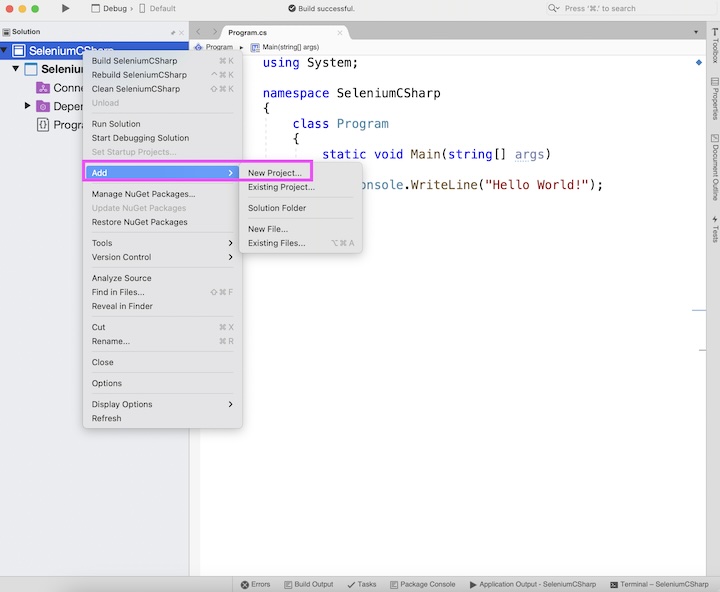
Step 3 − Select the option Console Application, then click on the Next button.
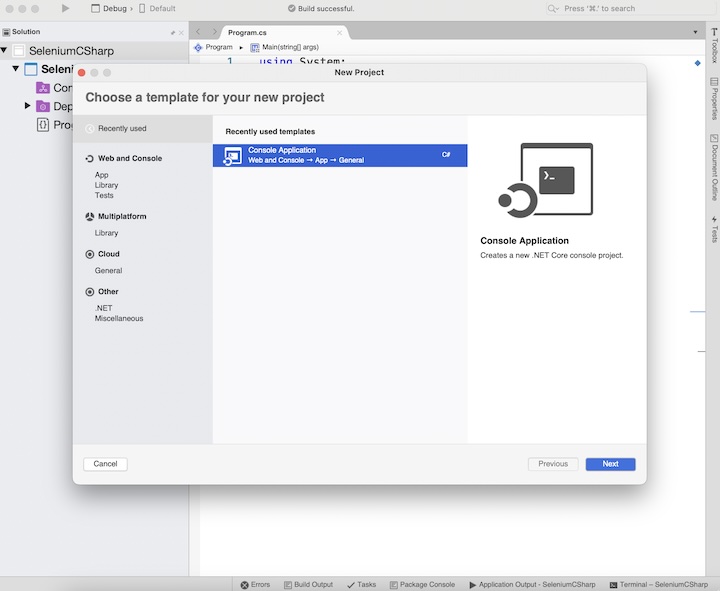
Step 4 − Enter a project name, say SeleniumTest, then click on the Create button.
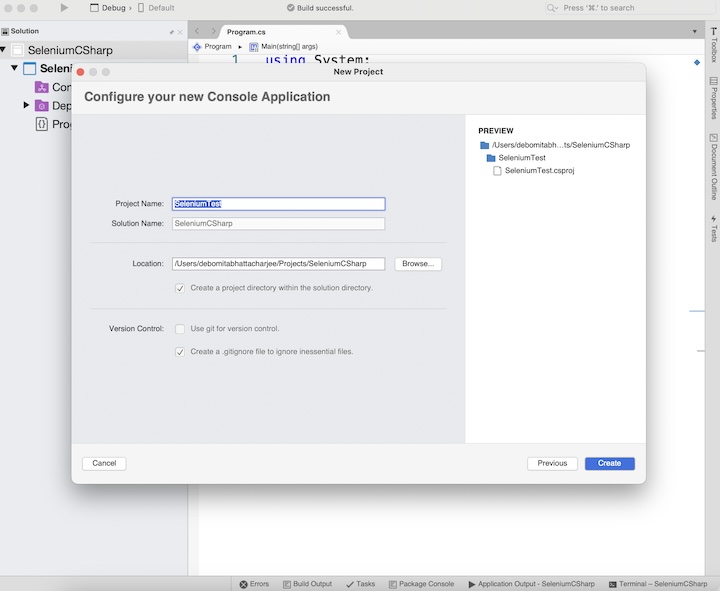
Step 5 − The newly created project - SeleniumTest should be visible.
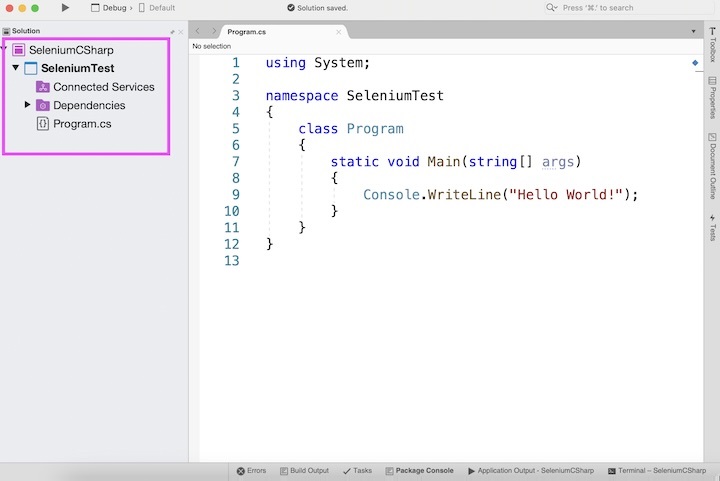
Step 6 − Right click on the newly created project and select the option Manage NuGet Packages.
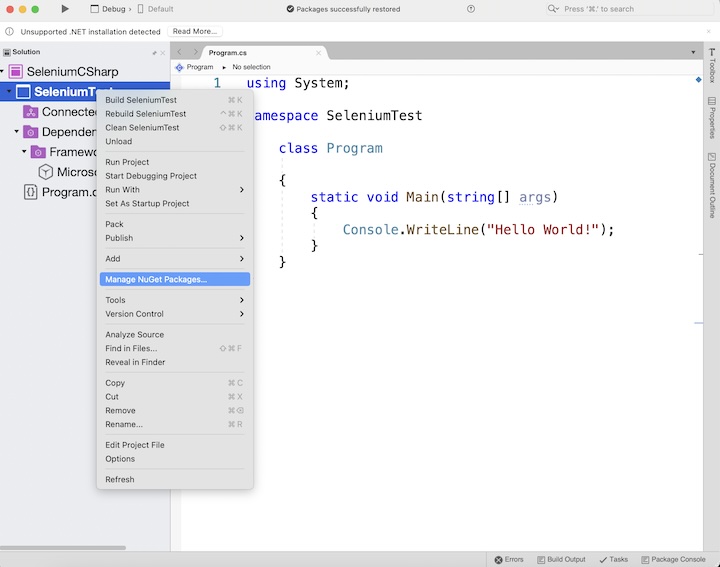
Step 7 − Enter selenium in the search box at the top right, all the packages based on selenium search should display. Click on the Selenium.WebDriver and click on the Add Packages button. Install all the Selenium related packages.
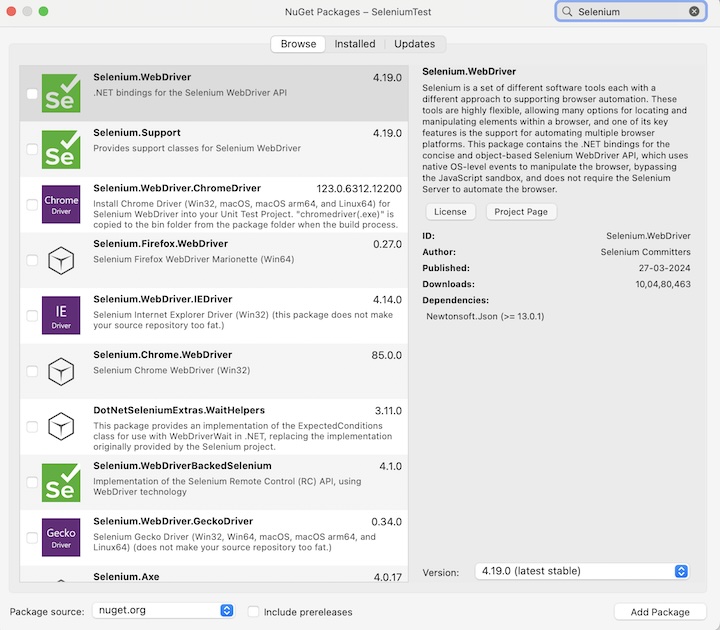
Step 8 − Once done, Selenium.Webdriver successfully added a message to display at the top of the Visual Studio.
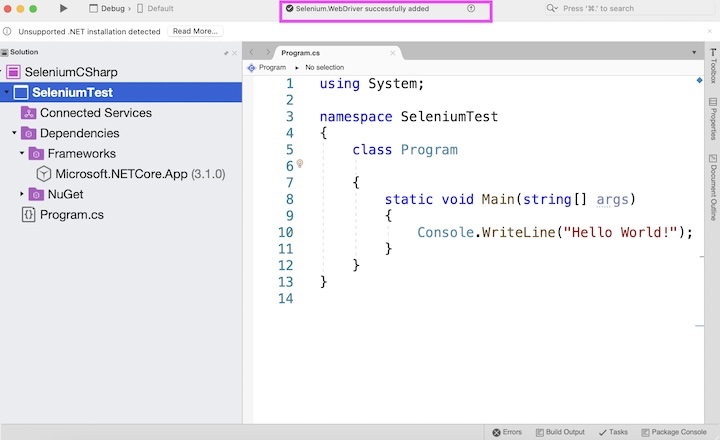
Step 9 − Restart the Visual Studio. After it is launched again, all the installed packages should be displayed under the NuGet folder in the Solution Explorer.
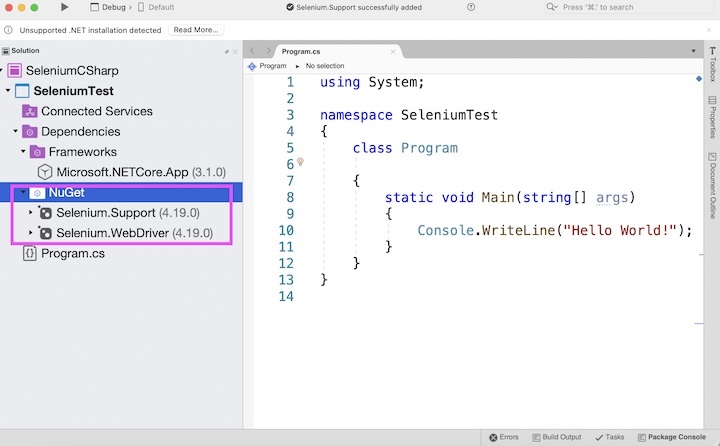
Step 10 − Write a code to obtain the page title - Selenium Practice - Modal Dialogs of the below page.
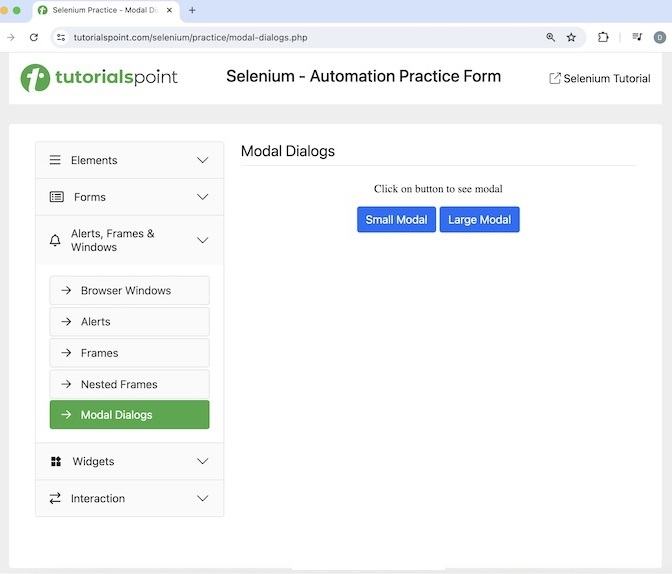
Example
using System; using OpenQA.Selenium; using OpenQA.Selenium.Chrome; namespace SeleniumTest { class Program { static void Main(string[] args) { // Initiate Webdriver IWebDriver driver = new ChromeDriver(); // adding an implicit wait of 20 secs driver.Manage().Timeouts().ImplicitWait = TimeSpan.FromSeconds(20); // launch the application driver.Navigate().GoToUrl("https://www.tutorialspoint.com/selenium/practice/modal-dialogs.php"); // get the page title String pageTitle = driver.Title; Console.WriteLine("Page title is: " + pageTitle); } } }
Output
Page title is: Selenium Practice - Modal Dialogs
In the above example, we had launched an application and obtained its page title in the console with the message - Page title is: Selenium Practice - Modal Dialogs.
Launch Browser and Quit Driver with Selenium C#
We can launch the browser and open an application using the driver.Navigate().GoToUrl method, and finally quit the browser with the Quit() method.
using System; using OpenQA.Selenium; using OpenQA.Selenium.Chrome; namespace SeleniumTest { class Program { static void Main(string[] args){ // Initiate Webdriver IWebDriver driver = new ChromeDriver(); // adding an implicit wait of 20 secs driver.Manage().Timeouts().ImplicitWait = TimeSpan.FromSeconds(20); // launch the application driver.Navigate().GoToUrl("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // quitting browser driver.Quit(); } } }
In the above example, we had first launched an application in the Chrome browser then quit the browser.
Identify Element and Check Its Functionality Using Selenium C#
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the element. There are some locators available in Selenium for this purpose, they are id, class, class name, name, link text, partial link text, tagname, css, and xpath. These locators need to be used with the FindElement() method.
For example, driver.FindElement(By.Id("id")) will locate the first web element with the given id attribute value. In case there is no element with the matching value of the id attribute, NoSuchElementException should be thrown.
Let us see the html code of the radio button beside the Impressive label highlighted in the below image −
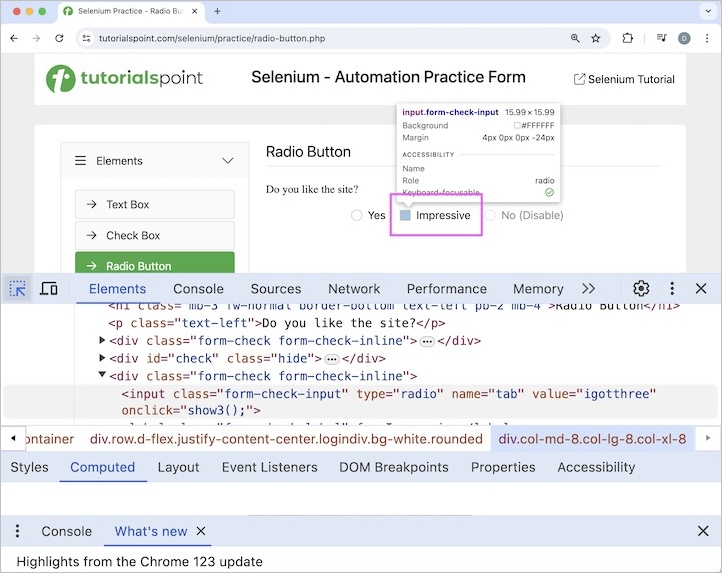
<input class="form-check-input" type="radio" name="tab" value="igotthree" onclick="show3();">
Let us click on that radio button after which we would get the text You have checked Impressive on the web page.
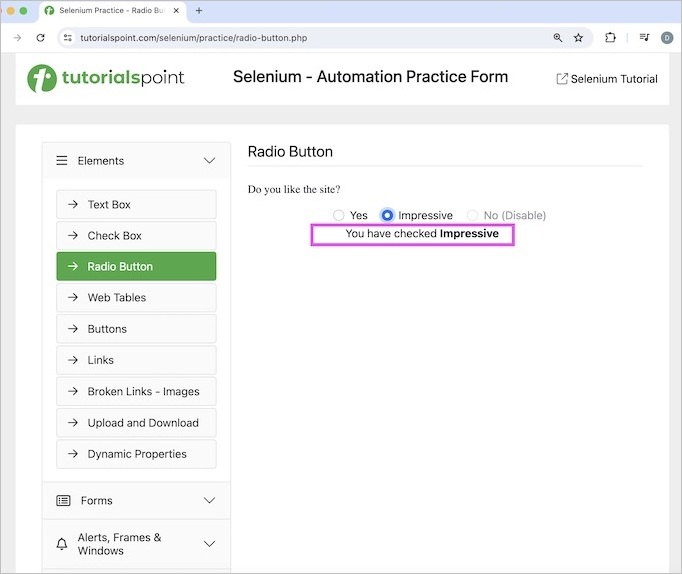
Example
using System; using OpenQA.Selenium; using OpenQA.Selenium.Chrome; namespace SeleniumTest { class Program { static void Main(string[] args){ // Initiate the Webdriver IWebDriver driver = new ChromeDriver(); // adding an implicit wait of 20 secs driver.Manage().Timeouts().ImplicitWait = TimeSpan.FromSeconds(20); // launch an application driver.Navigate().GoToUrl("https://www.tutorialspoint.com/selenium/practice/radio-button.php"); // identify a radio button then click on radio button IWebElement r = driver.FindElement (By.XPath("/html/body/main/div/div/div[2]/form/div[3]/input")); r.Click(); // identify text after clicking radio button IWebElement txt = driver.FindElement(By.Id("check1")); // obtain text String text = txt.Text; Console.WriteLine("Text is: " + text); // quitting browser driver.Quit(); } } }
Output
Text is: You have checked Impressive
In the above example, we had obtained the text after clicking the radio button beside the label Impressive with the message in the console - You have checked Impressive.
This concludes our comprehensive take on the tutorial on Selenium - C# Tutorial. We’ve started with describing how to set up Selenium with C# and launch a browser, and how to identify an element and check its functionality using Selenium C#.
This equips you with in-depth knowledge of the Selenium - C# Tutorial. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.