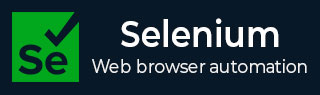
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium - Data Driven Framework
Selenium Webdriver can be used to develop test scripts which are based on the data driven framework. A data driven framework is mostly used to create test cases which are dependent on data available from an external source. This external source can be any file having extensions with .txt, .properties, .xlsx, .xls, .csv, and also with the help of data providers.
A data driven framework is the one in which the test cases are segregated from the data set. Also, it gives the provision to run the same test case against multiple sets of data. Since the test code implementation and test data are independent of each other, the data set can be modified without impacting the implementation logic and vice-versa.
Why Data Driven Testing Framework is Used?
In a data driven testing framework, more than one set of data can be run against the same test case, thus the same application can be tested against a wide data range without requiring additional code. Thus code is developed once but reused multiple times.
A data driven testing framework allows multiple execution of test cases without increasing the number of test cases. Sometimes, test data is generated automatically and this allows the application to be tested against a wide range of random data sets. In such a scenario, a data driven testing framework ensures a more robust and quality product.
Advantages of Data Driven Testing Framework
A data driven testing framework allows optimal use of test cases by ensuring a more structured execution. This also helps to shorten the test cycle while ensuring a quality product. The test implementation code comprising various functions, methods, and actions are tested with a wide range of data sets in a data driven testing framework.
Differences Between Data Driven and Keyword Driven Testing Framework
There exists some differences between the data driven and keyword driven frameworks. A keyword driven framework is easier to maintain since more abstractions remain among the test data, keywords, and implementation logic layers, while a data driven framework is more difficult to maintain than a keyword driven framework as abstraction exists only between the test data and test scripts.
More systemic planning is required to be in place for a keyword driven framework rather than in a data driven framework since in the data driven framework we are only segregating the test data from the test scripts.
Example 1 - Data Driven with Excel
Let us take an example where we would perform data driven testing with excel using the Apache POI library. Let us take an example of the below excel named the DetailsStudent.xlsx file, where we would read the value from that excel file and input those data to the below registration page and once successfully done, we would write the text - Test Case: Pass in the cell(at same row and Column E). If not successfully done, we would write the text - Test Case: Fail in that same cell.
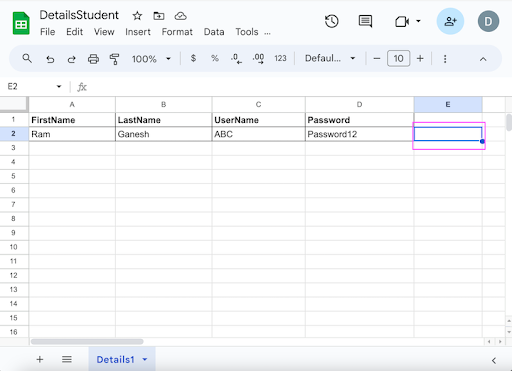
The below image shows the registration page where we would enter data in the fields Full Name:, Last Name:, Username:, and Password from the DetailsStudent.xlsx file.
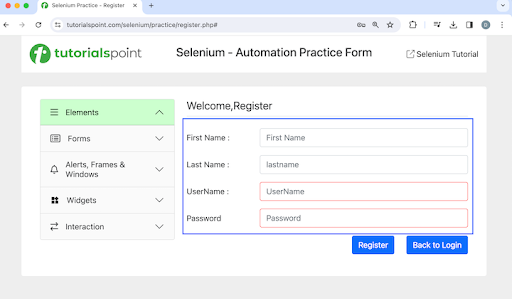
Please Note − The DetailsStudent.xlsx excel file was placed within the project under the Resources folder as shown in the below image.
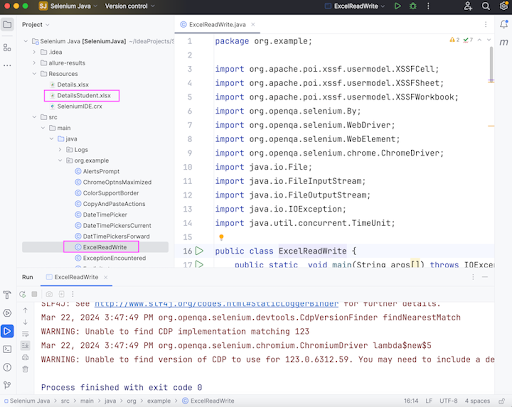
How to Install the Apache POI?
Step 1 − Add Apache POI Common dependencies from the below link −
https://mvnrepository.com/artifact/org.apache.poi/poi.
Step 2 − Add Apache POI API Based On OPC and OOXML Schemas dependencies from the below link −
https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml.
Step 3 − Save the pom.xml with all the dependencies and update the maven project.
Code Implementation in ExcelReadWrite.java
package org.example; import org.apache.poi.xssf.usermodel.XSSFCell; import org.apache.poi.xssf.usermodel.XSSFSheet; import org.apache.poi.xssf.usermodel.XSSFWorkbook; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.util.concurrent.TimeUnit; public class ExcelReadWrite { public static void main(String args[]) throws IOException { // identify location of .xlsx file File f = new File("./Resources/DetailsStudent.xlsx"); FileInputStream i = new FileInputStream(f); // instance of XSSFWorkbook XSSFWorkbook w = new XSSFWorkbook(i); // create sheet in XSSFWorkbook with name Details1 XSSFSheet s = w .getSheet("Details1"); // handle total rows in XSSFSheet int r = s.getLastRowNum() - s.getFirstRowNum(); // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS); // Opening the webpage where we will identify elements driver.get("https://www.tutorialspoint.com/selenium/practice/register.php#"); //Identify elements for registration WebElement fname = driver.findElement(By.xpath("//*[@id='firstname']")); WebElement lname = driver.findElement(By.xpath("//*[@id='lastname']")); WebElement uname = driver.findElement(By.xpath("//*[@id='username']")); WebElement pass = driver.findElement(By.xpath("//*[@id='password']")); WebElement btn = driver.findElement(By.xpath("//*[@id='signupForm']/div[5]/input")); // loop through rows, read and enter values in form for(int j = 1; j <= r; j++) { fname.sendKeys(s.getRow(j).getCell(0).getStringCellValue()); lname.sendKeys(s.getRow(j).getCell(1).getStringCellValue()); uname.sendKeys(s.getRow(j).getCell(2).getStringCellValue()); pass.sendKeys(s.getRow(j).getCell(3).getStringCellValue()); // submit registration form btn.click(); // verify form submitted WebElement fname1 = driver.findElement(By.xpath("//*[@id='firstname']")); String value = fname1.getAttribute("value"); // create cell at Column 4 to write values in excel XSSFCell c = s.getRow(j).createCell(4); // write results in excel if (value.isEmpty()) { c.setCellValue("Test Case: PASS"); } else { c.setCellValue("Test Case: FAIL"); } // complete writing value in excel FileOutputStream o = new FileOutputStream("./Resources/DetailsStudent.xlsx"); w.write(o); } // closing workbook object w.close(); // Quitting browser driver.quit(); } }
Dependencies added to pom.xml.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.poi/poi --> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi</artifactId> <version>5.2.5</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml --> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi-ooxml</artifactId> <version>5.2.5</version> </dependency> </dependencies> </project>
Output
Process finished with exit code 0
In the above example, we had read the whole excel file and had written the value Test Case: Pass in the cell at the fifth Column.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
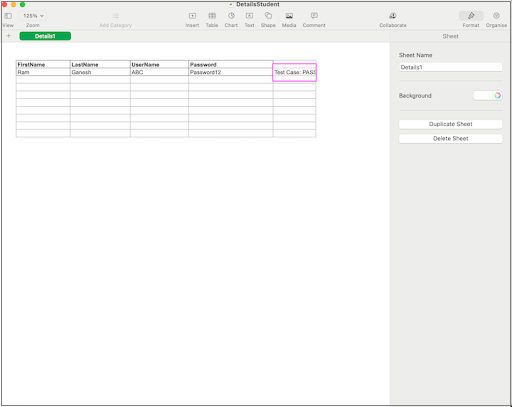
As seen in the image above, Test Case: Pass was written into DetailsStudent.xlsx excel file in the Column 5 post the test run with respect to the registration data available in the same excel.
Example 2 - Data Driven with TestNG DataProvider
Let us take an example where we would perform data driven testing with the help of the default functionality of TestNG which is known as the DataProvider. The DataProvider is used to feed data to any test method.
We would input values in the same registration page as mentioned in the previous example where we would enter data in the fields Full Name:, Last Name:, Username:, and Password using the TestNG DataProvider.
How to Install the TestNG?
Install Java(version above 8) in the system and check if it is present with the command: java -version. The java version installed will be visible if installation has been completed successfully.
Install maven in the system and check if it is present with the command: mvn -version. The maven version installed will be visible if installation has been completed successfully.
Install any IDE like Eclipse, IntelliJ, and so on.
Add the TestNG dependencies from the link below: https://mvnrepository.com/artifact/org.testng/testng.
Code Implementation in DataTest.java
package CrossBrw; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.annotations.*; import java.util.concurrent.TimeUnit; public class DataTest { WebDriver driver; @BeforeTest public void setup() throws Exception { // Initiate the Webdriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/register.php#"); } @Test(dataProvider = "registration-data") public void userRegistration (String fname1, String lname1, String uname1, String pass1) { //Identify elements for registration WebElement fname = driver.findElement(By.xpath("//*[@id='firstname']")); WebElement lname = driver.findElement(By.xpath("//*[@id='lastname']")); WebElement uname = driver.findElement(By.xpath("//*[@id='username']")); WebElement pass = driver.findElement(By.xpath("//*[@id='password']")); // enter details from data provider fname.sendKeys(fname1); lname.sendKeys(lname1); uname.sendKeys(uname1); pass.sendKeys(pass1); // get values entered String text = fname.getAttribute("value"); String text1 = lname.getAttribute("value"); String text2 = uname.getAttribute("value"); String text3 = pass.getAttribute("value"); System.out.println("Entered first name: " + text); System.out.println("Entered last name: " + text1); System.out.println("Entered username: " + text2); System.out.println("Entered password: " + text3); } @DataProvider (name = "registration-data") public Object[][] registrationData(){ return new Object[][] { {"Ram", "Ganesh", "ABC", "Password12"} }; } @AfterTest public void teardown() { // quitting browser driver.quit(); } }
Dependencies added to pom.xml.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <!-- https://mvnrepository.com/artifact/org.testng/testng --> <dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.9.0</version> <scope>test</scope> </dependency> </dependencies> </project>
Output
Entered first name: Ram Entered last name: Ganesh Entered username: ABC Entered password: Password12 =============================================== Default Suite Total tests run: 1, Passes: 1, Failures: 0, Skips: 0 =============================================== Process finished with exit code 0
In the above example, we had input the data in the registration page from the data available in the DataProvider and obtained the values entered with the message in the console - Entered first name: Ram, Entered last name: Ganesh, Entered username: ABC, and Entered password: Password12.
The result in the console shows Total tests run: 1, as there is one method= with @Test annotation - userRegisterration().
Finally, the message Passes: 1, and Process finished with exit code 0 was received, signifying successful execution of the code.
This concludes our comprehensive take on the tutorial on Selenium Webdriver - Data Driven Framework. We’ve started with describing what is a data driven framework, why is a data driven framework used, what are the advantages of a data driven framework, what are the differences between data driven and keyword driven frameworks, and walked through examples of how to implement a data driven framework using excel and dataProvider in TestNG along with Selenium Webdriver.
This equips you with in-depth knowledge of the Data Driven Framework in Selenium Webdriver. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.