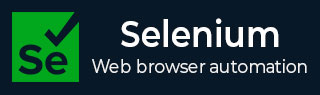
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium WebDriver - Browsing Context
Selenium Webdriver can be used for Browsing Context. There are multiple APIs which are used for the browser context commands. Some of the operations which can be performed using the Browsing Context are opening a new window, tab, utilizing an already opened window, opening a URL, and so on.
Create Browsing Context
In order to create a Browsing Context, we would use the ChromeOptions class, then set the capability webSocketUrl to the true value and pass its reference to the Webdriver. Please Note, We would also need to import the below statement in order to use the methods of the Browsing Context.
import org.openqa.selenium.bidi.browsingcontext.BrowsingContext.
While tests are running using the APIs on browsing context commands, there would be one tab opened with the title BiDi-CDP Mapper having the message - BiDi-CDP Mapper is controlling this tab along with the debug information. This would prove that the automation tests are using the Browsing Context.
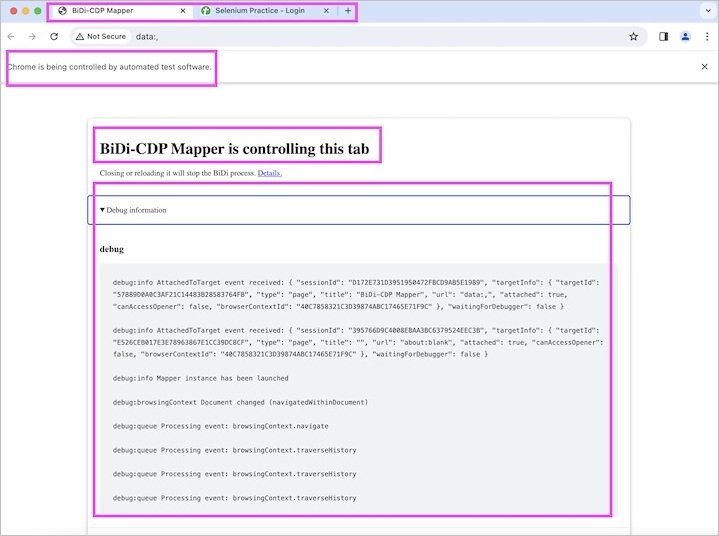
The next tab would open the application triggered from the automation test.
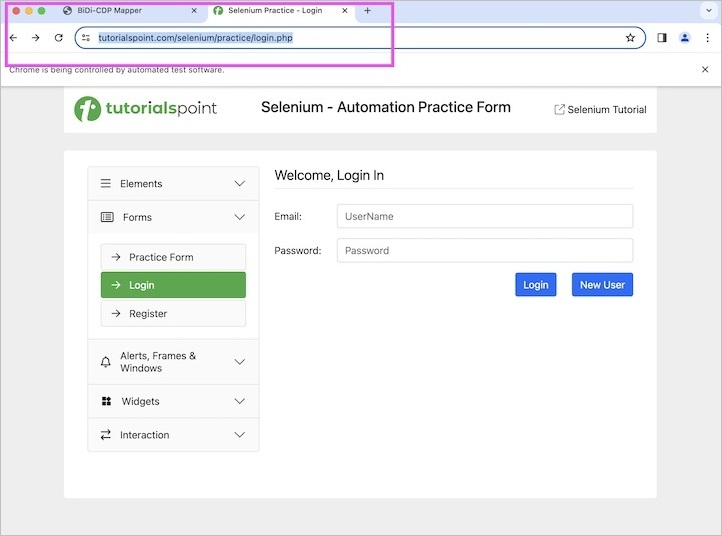
The Browsing Context is Selenium is a feature available from the Selenium 4.x version. To utilize all the APIs on the browser context commands, it is recommended to use the Selenium Maven dependency from Selenium 4.16.x versions.
Dependencies To Be Added To pom.xml For Browsing Context
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.16.1</version> </dependency> <!-- https://mvnrepository.com/artifact/org.testng/testng --> <dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.9.0</version> <scope>test</scope> </dependency> </dependencies> </project>
Create Browsing Context in New Window and Navigate to URL
Let us take an example where we would open a browser, then open another new window and launch an application having the below URL to create the Browsing Context −
https://www.tutorialspoint.com/selenium/.
Code Implementation in BrowsingContextNewWindows.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.NavigationResult; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextNewWindows { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // open browsing context in new window BrowsingContext bc = new BrowsingContext(driver, WindowType.WINDOW); // obtain id of browsing context in new window String text = bc.getId(); System.out.println("Id of browsing context in new window: " + text); // navigate to new url in the new window NavigationResult i = bc.navigate("https://www.tutorialspoint.com/selenium/practice/buttons.php"); // get new URL opened in the new window System.out.println("Get URL: " + i.getUrl()); // Quitting browser driver.quit(); } }
It will show the following output −
Id of browsing context in new window: 14CC57B70B48D044482821778B560618 Get URL: https://www.tutorialspoint.com/selenium/practice/buttons.php Process finished with exit code 0
In the above example, we had opened a browser, then opened another new window and launched an application having the below URL to create the Browsing Context. We had obtained the browsing context id of the new window and the URL launched in the console with messages - Id of browsing context in new window: 14CC57B70B48D044482821778B560618 and Get URL: https://www.tutorialspoint.com/selenium/.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Create Browsing Context in New Tab and Navigate to URL
Let us take an example where we would open a browser, then open another new tab and launch an application having the below URL there to create the Browsing Context − https://www.tutorialspoint.com/selenium/.
Code Implementation in BrowsingContextNewTabs.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.NavigationResult; import org.openqa.selenium.bidi.browsingcontext.ReadinessState; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextNewTabs { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // open browsing context in new tab BrowsingContext bc = new BrowsingContext(driver, WindowType.TAB); // obtain id of browsing context in new tab String text = bc.getId(); System.out.println("Id of browsing context in new tab: " + text); // navigate to new url in the new tab in readiness state NavigationResult i = bc.navigate("https://www.tutorialspoint.com/selenium/practice/buttons.php", ReadinessState.COMPLETE); // get new URL opened in the new tab System.out.println("Get URL: " + i.getUrl()); // Quitting browser driver.quit(); } }
It will show the following output −
Id of browsing context in new tab: E7D9C6C1EA830EE2E968EFFDAE800EE2 Get URL: https://www.tutorialspoint.com/selenium/practice/buttons.php Process finished with exit code 0
In the above example, we had opened a browser, then opened another new tab and launched an application having the below URL to create the Browsing Context. We had obtained the browsing context id of the new tab and the URL launched in the console with messages - Id of browsing context in new tab: E7D9C6C1EA830EE2E968EFFDAE800EE2 and Get URL: https://www.tutorialspoint.com/selenium/.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Create Browsing Context Using Existing Window/Tab Handle
Let us take an example where we would create a Browsing Context using an existing window/tab.
Code Implementation in BrowsingContextNewTab.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextNewTab { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); // open browsing context in new tab/window BrowsingContext bc = new BrowsingContext(driver, windowID); // obtain id of browsing context in new tab/window String text = bc.getId(); System.out.println("Id of browsing context in new tab/window: " + text); // Quitting browser driver.quit(); } }
It will show the following output −
Id of browsing context in new tab/window: E5EB8BA6EBAFBA14561CC8EB23360034 Process finished with exit code 0
In the above example, we had created the Browsing Context using an existing window/tab handle. We had obtained the browsing context id in the console with message - Id of browsing context in new/window: E5EB8BA6EBAFBA14561CC8EB23360034.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Create Browsing Context After Opening New Window Having Reference Browsing Context
Let us take an example where we would create a Browsing Context by opening a new window having a reference browsing context.
Code Implementation in BrowsingContextNewWindow.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextNewWindow { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); // open browsing context in new window using browsing context BrowsingContext bc = new BrowsingContext(driver, WindowType.WINDOW, windowID); // obtain id of browsing context String text = bc.getId(); System.out.println("Id of browsing context in new window: " + text); // Quitting browser driver.quit(); } }
It will show the following output −
Id of browsing context in new window: F82CF86144C26AEA4168F672C11B8C92 Process finished with exit code 0
In the above example, we had created the Browsing Context after opening a new window having a reference browsing context. We had obtained the browsing context id in the console with message - Id of browsing context in window: F82CF86144C26AEA4168F672C11B8C92.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Create Browsing Context After Opening New Tab Having Reference Browsing Context
Let us take an example where we would create a Browsing Context by opening a new tab having a reference browsing context.
Code Implementation in BrowsingContextNewTab.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextNewTab { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); // open browsing context in new tab using browsing context BrowsingContext bc = new BrowsingContext(driver, WindowType.TAB, windowID); // obtain id of browsing context String text = bc.getId(); System.out.println("Id of browsing context in new tab: " + text); // Quitting browser driver.quit(); } }
It will show the following output −
Id of browsing context in new tab: C88F54A24AF3C6DE71C5B5E37913BA77 Process finished with exit code 0
In the above example, we had created the Browsing Context after opening a new tab having a reference browsing context. We had obtained the browsing context id in the console with message - Id of browsing context in tab: C88F54A24AF3C6DE71C5B5E37913BA77.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Get the Browsing Context Tree
Let us take an example where we would obtain every browsing context starting from the parent browsing context.
Code Implementation in BrowsingContextTree.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.BrowsingContextInfo; import org.openqa.selenium.bidi.browsingcontext.ReadinessState; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.List; import java.util.concurrent.TimeUnit; public class BrowsingContextTree { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); // open browsing context in new tab/window BrowsingContext bc = new BrowsingContext(driver, windowID); // open another URL bc.navigate("https://www.tutorialspoint.com/selenium/practice/buttons.php", ReadinessState.COMPLETE); // list of browsing context tree List<BrowsingContextInfo> tree = bc.getTree(); // obtain total browsing context tree int size = tree.size(); System.out.println("Total browsing contexts: " + size); // obtain total children BrowsingContextInfo c = tree.get(0); int size1 = c.getChildren().size(); System.out.println("Total children: " + size1); // Quitting browser driver.quit(); } }
It will show the following output −
Total browsing contexts: 1 Total children: 0 Process finished with exit code 0
Get the Browsing Context Tree with Depth Value
Let us take an example where we would obtain every browsing context starting from the parent browsing context along with depth value.
Code Implementation in BrowsingContextTreeDepth.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.BrowsingContextInfo; import org.openqa.selenium.bidi.browsingcontext.ReadinessState; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.List; import java.util.concurrent.TimeUnit; public class BrowsingContextTreeDepth { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); System.out.println("Window Handle Id: " + windowID); // open browsing context in new tab/window BrowsingContext bc = new BrowsingContext(driver, windowID); // open another URL bc.navigate("https://www.tutorialspoint.com/selenium/practice/buttons.php", ReadinessState.COMPLETE); // list of browsing context tree with depth value List<BrowsingContextInfo> tree = bc.getTree(0); // obtain total browsing context tree int size = tree.size(); System.out.println("Total browsing contexts: " + size); // obtain total children BrowsingContextInfo c = tree.get(0); List children = c.getChildren(); System.out.println("Total children: " + children); System.out.println("Browsing Context id: " + c.getId()); // Quitting browser driver.quit(); } }
It will show the following output −
Window Handle Id: DE5E963CFFCC9A42A5F7A4903BF0C12C Total browsing contexts: 1 Total children: null Browsing Context id: DE5E963CFFCC9A42A5F7A4903BF0C12C Process finished with exit code 0
Get All the Top Level Browsing Contexts
Let us take an example where we would obtain every top level browsing contexts.
Code Implementation in BrowsingContextTopLevel.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.BrowsingContextInfo; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.List; import java.util.concurrent.TimeUnit; public class BrowsingContextTopLevel { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); // open browsing context in new tab/window BrowsingContext bc1 = new BrowsingContext(driver, windowID); // open another browsing context in new window BrowsingContext bc2 = new BrowsingContext(driver, WindowType.WINDOW); // list of every top level browsing contexts List<BrowsingContextInfo> tree = bc1.getTopLevelContexts(); // obtain total browsing context tree int size = tree.size(); System.out.println("Total top level browsing context: " + size); // Quitting browser driver.quit(); } }
It will show the following output −
Total top level browsing context: 2 Process finished with exit code 0
In the above example, we obtained all the top level browsing contexts in the console with message - Total top level browsing context: 2.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Close a Window Browsing Context
Let us take an example where we would close a window browsing context using the close() method.
Code Implementation in BrowsingContextWindowClose.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.BrowsingContextInfo; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.List; import java.util.concurrent.TimeUnit; public class BrowsingContextWindowClose { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // open browsing context in new window BrowsingContext bc1 = new BrowsingContext(driver, WindowType.WINDOW); // open another browsing context in new window BrowsingContext bc2 = new BrowsingContext(driver, WindowType.WINDOW); // list of every top level browsing contexts List<BrowsingContextInfo> tree = bc1.getTopLevelContexts(); // obtain total browsing context tree int size = tree.size(); System.out.println("Total top level browsing context before closing: " + size); // close one browsing context bc2.close(); // obtain total browsing context tree after closing one List<BrowsingContextInfo> tree1 = bc1.getTopLevelContexts(); int size1 = tree1.size(); System.out.println("Total top level browsing context after closing: " + size1); // Quitting browser driver.quit(); } }
It will show the following output −
Total top level browsing context before closing: 3 Total top level browsing context after closing: 2 Process finished with exit code 0
Close a Tab Browsing Context
Let us take an example where we would close a tab browsing context using the close() method.
Code Implementation in BrowsingContextTabClose.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.BrowsingContextInfo; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.List; import java.util.concurrent.TimeUnit; public class BrowsingContextTabClose { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // open browsing context in new tab BrowsingContext bc1 = new BrowsingContext(driver, WindowType.TAB); // open another browsing context in new tab BrowsingContext bc2 = new BrowsingContext(driver, WindowType.TAB); // list of every top level browsing contexts List<BrowsingContextInfo> tree = bc1.getTopLevelContexts(); // obtain total browsing context tree int size = tree.size(); System.out.println("Total top level browsing context before closing: " + size); // close one browsing context bc2.close(); // obtain total browsing context tree after closing one List<BrowsingContextInfo> tree1 = bc1.getTopLevelContexts(); int size1 = tree1.size(); System.out.println("Total top level browsing context after closing: " + size1); // Quitting browser driver.quit(); } }
It will show the following output −
Total top level browsing context before closing: 3 Total top level browsing context after closing: 2 Process finished with exit code 0
Activate a Browsing Context
Let us take an example where we would activate a browsing context using the activate() method.
Code Implementation in BrowsingContextTabClose.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextTabClose { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc1 = new BrowsingContext(driver, windowID); // open another browsing context in new window BrowsingContext bc2 = new BrowsingContext(driver, WindowType.WINDOW); // activate browsing context bc1.activate(); // Quitting browser driver.quit(); } }
Reload a Browsing Context
Let us take an example where we would reload a browsing context and obtain the navigation id, and url.
Code Implementation in BrowsingContextReload.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.NavigationResult; import org.openqa.selenium.bidi.browsingcontext.ReadinessState; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextReload { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php"); // open browsing context in new tab BrowsingContext bc = new BrowsingContext(driver, WindowType.TAB); // navigate to URL in a browsing context bc.navigate("https://www.tutorialspoint.com/selenium/practice/buttons.php", ReadinessState.COMPLETE); // reload browsing context NavigationResult load = bc.reload(ReadinessState.INTERACTIVE); // get navigation id String text = load.getNavigationId(); System.out.println("Navigation ID: " + text); // get URL opened in browsing context String url = load.getUrl(); System.out.println("Navigation URL: " + url); // Quitting browser driver.quit(); } }
It will show the following output −
Navigation ID: 0C12BD5C26E08392DB18F6C7928F8020 Navigation URL: https://www.tutorialspoint.com/selenium/practice/buttons.php Process finished with exit code 0
In the above example, we had reloaded the browsing context and had obtained navigation id and url with messages in the console - Navigation ID: 0C12BD5C26E08392DB18F6C7928F8020, and Navigation URL: https://www.tutorialspoint.com/selenium/.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Handle User Prompts with Browsing Context
Let us take an example where we would handle user prompts with browsing context using the handleUserPrompt() method.
Code Implementation in BrowsingContextHandlePrompt.java
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextHandlePrompt { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/alerts.php"); // get window handle String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc = new BrowsingContext(driver, driver.getWindowHandle()); // identify button WebElement btn = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/div[1]/button")); btn.click(); // accept and input text in prompt bc.handleUserPrompt(true, "Tutorialspoint"); // Quitting browser driver.quit(); } }
It will show the following output −
Process finished with exit code 0
In the above example, we had handled the user prompt with the Browsing Context.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Capture Screenshots with Browsing Context
Let us take an example where we would capture screenshots with browsing context using the captureScreenshot() method.
Code Implementation in BrowsingContextCaptureScreenshot.java
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextCaptureScreenshot { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/alerts.php"); // get window handle String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc = new BrowsingContext(driver, driver.getWindowHandle()); // identify button then click WebElement btn = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/div[1]/button")); btn.click(); // capture screenshot String sc = bc.captureScreenshot(); // get number of screenshot int size = sc.length(); System.out.println("Size of Screenshot: " + size); // Quitting browser driver.quit(); } }
It will show the following output −
Size of Screenshots: 209152 Process finished with exit code 0
Capture a Screenshot of an Element with Browsing Context
Let us take an example where we would capture a screenshot of an element with browsing context using the captureElementScreenshot() method.
Code Implementation in BrowsingContextElemntCaptureScreenshot.java
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import org.openqa.selenium.remote.RemoteWebElement; import java.util.concurrent.TimeUnit; public class BrowsingContextElemntCaptureScreenshot { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // get window handle String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc = new BrowsingContext(driver, windowID); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/alerts.php"); // identify button WebElement btn = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/div[1]/button")); String sc = bc.captureElementScreenshot(((RemoteWebElement) btn).getId()); // get number of screenshot int size = sc.length(); System.out.println("Size of Screenshot of an element: " + size); // Quitting browser driver.quit(); } }
It will show the following output −
Size of Screenshot of an element: 3080 Process finished with exit code 0
Set the Viewport Pixel with Browsing Context
Let us take an example where we would set a viewport with a pixel with browsing context using the setViewport() method.
Code Implementation in SetViewPort.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class SetViewPort { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // get window handle String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc = new BrowsingContext(driver, windowID); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/alerts.php"); // set view port bc.setViewport(200, 600, 6); // Quitting browser driver.quit(); } }
It will show the following output −
Process finished with exit code 0
Print a Page with Browsing Context
Let us take an example where we would print a page with browsing context using the print() method.
Code Implementation in PrintPage.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import org.openqa.selenium.print.PrintOptions; import java.util.concurrent.TimeUnit; public class PrintPage { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // get window handle String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc = new BrowsingContext(driver, windowID); // launching a browser and open a URL driver.get("https://www.tutorialspoint.com/selenium/practice/alerts.php"); // object of PrintOptions PrintOptions p = new PrintOptions(); // get print String print = bc.print(p); // Quitting browser driver.quit(); } }
Navigate, Move Forward, Backward, and Traverse in Browser History with Browsing Context
Let us take an example where we move forward, backward, and traverse in browser history with browsing context. These are performed using the forward(), back(), and traverse(-1) methods of the BrowsingContext class.
Code Implementation in BrowsingContextHistory.java
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.ReadinessState; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextHistory { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // get window handle String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc = new BrowsingContext(driver, windowID); // navigate to URL bc.navigate("https://www.tutorialspoint.com/selenium/practice/login.php", ReadinessState.COMPLETE); // identify element then click WebElement btn = driver.findElement(By.xpath("//*[@id='signInForm']/div[3]/a")); btn.click(); // get page title after click String title = driver.getTitle(); System.out.println("Page title after click: " + title); // navigate back in browser history bc.back(); // get page title after navigating back in browser history String title1 = driver.getTitle(); System.out.println("Page title after navigating back in browser history: " + title1); // navigate forward in browser history bc.forward(); // get page title after navigating forward in browser history String title2 = driver.getTitle(); System.out.println("Page title after navigating forward in browser history: " + title2); // traverse history bc.traverseHistory(-1); // Quitting browser driver.quit(); } }
It will show the following output −
Page title after click: Selenium Practice - Register Page title after navigating back in browser history: Selenium Practice - Login Page title after navigating forward in browser history: Selenium Practice - Register Process finished with exit code 0
In the above example, we had launched an application and navigated back, and, forward in the browser history and obtained the browser title after navigations in the console with the messages - Page title after click: Selenium Practice - Register, Page title after navigating back in browser history: Selenium Practice - Login, and Page title after navigating forward in browser history: Selenium Practice - Register.
This concludes our comprehensive take on the tutorial on Selenium WebDriver - Browser Context. We’ve started with describing what is Browser Context, and the Selenium dependencies required for the Browser Context, walked through the examples to create Browser Context in a new window and tab, in a new window, and tab using an existing window handle, navigate to a URL in normal and readiness state, obtain browsing context tree, its depth, and all top level browsing contexts, close a tab or window, activate and reload a browsing context, handle user prompt, capture screenshot of a web page, and specific element, set viewport with pixel, print page, and navigate forward, backward, and traversing in browser history with Selenium.
This equips you with in-depth knowledge of the Selenium WebDriver - Browser Context. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.