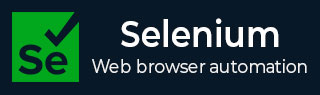
- Selenium Tutorial
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium IDE Tutorial
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - Errors & Logging
- Selenium - Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
- Selenium Useful Resources
- Selenium - Quick Guide
- Selenium - Useful Resources
- Selenium - Automation Practice
- Selenium - Discussion
Selenium Webdriver - Driver Sessions
The webdriver sessions mostly refer to the beginning and closing of the browser. A session is created by default by initializing the driver class object. There are two types of driver class - Local Driver and Remote Driver.
For quitting the driver, the quit() method is used. It is always recommended to use the quit() instead of close(), because close() method can only close the browser in focus and the session id will be returned as invalid or expired, while the quit() method terminates the driver session, and the session id will be returned as null.
Session Handling with Selenium Webdriver
We can perform session handling with the help of Selenium webdriver using the TestNG framework. To trigger different sessions, we shall use the attribute parallel in the TestNG XML file.
A TestNG execution configuration is done in the TestNG XML. To create multiple sessions, we shall add the attributes – parallel and thread-count in the XML file.
The attribute thread-count controls the number of sessions to be created while executing the tests in a parallel mode. The value of the parallel attribute is set to methods.
Get Session IDS In Selenium Webdriver
To get multiple session ids, we would create three methods which will in parallel. We would get the different session ids in this test using the RemoteWebdriver class.
A RemoteWebdriver is a class which implements the webdriver interface. The RemoteWebdriver is used widely to trigger execution in the remote machines. A session id is a distinct number assigned to each session. It has a format in UUID.
Example - Get Session IDS
Code Implementation in SessionHandling.java
package Report; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.remote.SessionId; import org.testng.annotations.*; import org.openqa.selenium.remote.RemoteWebDriver; public class SessionHandling { WebDriver driver; @Test public void method1() { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); //launch URL driver.get("https://www.tutorialspoint.com/selenium/practice/browser-windows.php"); //get session ID SessionId s = ((RemoteWebDriver)driver).getSessionId(); System.out.println("Session Id is for method1: " + s); } @Test public void method2() { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); //launch URL driver.get("https://www.tutorialspoint.com/selenium/practice/login.php"); //get session ID SessionId s = ((RemoteWebDriver)driver).getSessionId(); System.out.println("Session Id is for method2: " + s); } @Test public void method3() { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); //launch URL driver.get("https://www.tutorialspoint.com/selenium/practice/accordion.php"); //get session ID SessionId s = ((RemoteWebDriver)driver).getSessionId(); System.out.println("Session Id is for method3: " + s); } }
Configurations in testng.xml file.
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd"> <suite name="All Test Suite" parallel="methods" thread-count="3"> <test verbose="2" preserve-order="true" name="TestNGTest.java"> <classes> <class name="Report.SessionHandling"></class> </classes> </test> </suite>
Dependencies in pom.xml file.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <!-- https://mvnrepository.com/artifact/com.googlecode.json-simple/json-simple --> <dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.9.0</version> <scope>test</scope> </dependency> </dependencies> </project>
Project structure of the above implementation is shown in the below image −
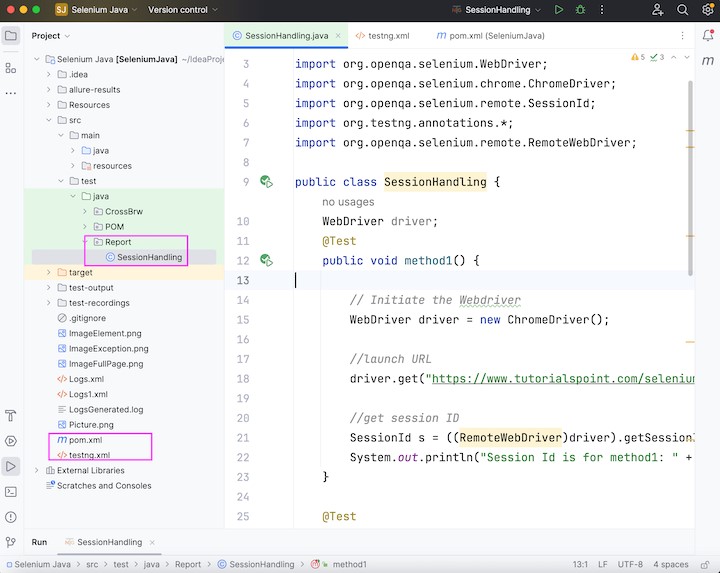
Output
Session Id is for method1: 63eae5bba42f97e30e7d01ef211f51e9 Session Id is for method2: 0b23f13aee68ca7c92de1cd93ee78c93 Session Id is for method3: e06fdaa8de91f4706e81566c854d1cf1 =============================================== Default Suite Total tests run: 3, Passes: 3, Failures: 0, Skips: 0 =============================================== Process finished with exit code 0
The result in the console shows Total tests run: 3, as there were three methods with @Test annotations - method1(), method2(), and method3(). Also, we had obtained the session ids of the three methods running in the parallel with the messages in console - Session Id is for method1: 63eae5bba42f97e30e7d01ef211f51e9, Session Id is for method2: 0b23f13aee68ca7c92de1cd93ee78c93, and Session Id is for method3: e06fdaa8de91f4706e81566c854d1cf1.
Finally, the message Passes: 3, and Process finished with exit code 0 was received, signifying successful execution of the code.
The below link provides a detailed description of TestNG −
https://www.tutorialspoint.com/testng/index.htm.
Get Single Session ID in Selenium WebDriver
To get a single session id, we would open a session using the RemoteWebdriver class. A session id is unique to every session. A RemoteWebdriver is a class which implements the webdriver interface. The RemoteWebdriver is used widely to trigger execution in the remote machines.
Example - Get Single ID
Code Implementation in HandlingSessionID.java
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class HandlingSessionID { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will get the session id driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); //get webdriver session id SessionId s = ((RemoteWebDriver) driver).getSessionId(); System.out.println("Session Id is: " + s); // Closing browser driver.quit(); } }
Dependencies in pom.xml file.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> </dependencies> </project>
Output
Session Id is: 78eae5bba42f97e30e7d01ef234f51e9 Process finished with exit code 0
In the above example, we had obtained the session id of webdriver with the message in the console - Session Id is: 78eae5bba42f97e30e7d01ef234f51e9.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
This concludes our comprehensive take on the tutorial on Selenium Webdriver - Driver Sessions. We’ve started with describing a webdriver session, differences between close() and quit() methods, and walked through an example illustrating how to get the session ids with Selenium.
This equips you with in-depth knowledge of the Selenium webdriver - driver sessions. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.