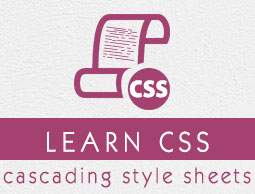
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Forms
HTML forms are required, when you want to collect some data from the site visitor. They have input fields for users to enter information, labels to identify the input fields, and buttons to submit the form or perform an action.
CSS can be used to make forms look good. You can style forms using CSS to change the appearance of form elements, such as text fields, checkboxes, radio buttons, select menus, and submit buttons.
Following sections discuss some ways to style forms using CSS:
A simple HTML form looks as in the following code:
<form> <input type="text" id="username" name="username" placeholder="Username"> <input type="password" id="password" name="password" placeholder="password"> <textarea id="message" name="message" rows="4" placeholder="Message"></textarea> <input type="submit" value="Submit"> </form>
Here are some common CSS techniques and properties you can use to style HTML forms:
CSS Form - Font and Text Styling
You can style the text within form elements using CSS properties like font-family, font-size, color and text-align as demonstrated below −
input[type="text"], input[type="password"], textarea { font-family: Arial, sans-serif; font-size: 16px; color: blue; }
Following example demonstrates this:
<html> <head> <style> input[type="text"], input[type="password"], textarea { font-family: Arial, sans-serif; font-size: 16px; color: blue; } label{ color:brown; } form{ display: grid; } </style> </head> <body> <h2>Font and Text Styling</h2> <form> <input type="text" id="username" name="username" placeholder="Username"> <input type="password" id="password" name="password" placeholder="password"> <textarea id="message" name="message" rows="4" placeholder="Message"></textarea> <input type="submit" value="Submit"> </form> </body> </html>
CSS Form - Styling Element Borders and Backgrounds
You can control the borders and backgrounds of form elements using properties like border, background-color, and border-radius.
input[type="text"], input[type="password"], textarea { border: 1px solid red; background-color: antiquewhite; border-radius: 5px; }
Following example demonstrates this:
<html> <head> <style> input[type="text"], input[type="password"], textarea { border: 1px solid red; background-color: antiquewhite; border-radius: 5px; } label{ color:brown; } form{ display: grid; } </style> </head> <body> <h2>Styling Form Element Borders and Backgrounds</h2> <form> <input type="text" id="username" name="username" placeholder="Username"> <input type="password" id="password" name="password" placeholder="password"> <textarea id="message" name="message" rows="4" placeholder="Message"></textarea> <input type="submit" value="Submit"> </form> </body> </html>
CSS Form - Using Padding and Margins
Adjust the spacing within and around form elements using padding and margin .
input[type="text"], input[type="password"], textarea { padding: 10px; margin: 5px 0; }
Following example demonstrates this:
<html> <head> <style> input[type="text"], input[type="password"], textarea { border: 1px solid red; background-color: antiquewhite; border-radius: 5px; padding: 10px; margin: 5px 0; } label{ color:brown; } form{ display: grid; } </style> </head> <body> <h2>Using Padding and Margins In Forms</h2> <form> <input type="text" id="username" name="username" placeholder="Username"> <input type="password" id="password" name="password" placeholder="password"> <textarea id="message" name="message" rows="4" placeholder="Message"></textarea> <input type="submit" value="Submit"> </form> </body> </html>
CSS Form - Focus Styles
You can define styles for when form elements are in focus using the :focus pseudo-class.
input[type="text"]:focus, input[type="password"]:focus, textarea:focus { border: 2px solid #007bff; }
Following example demonstrates this:
<html> <head> <style> input[type="text"], input[type="password"], textarea { border: 1px solid red; background-color: antiquewhite; border-radius: 5px; padding: 10px; margin: 5px 0; } input[type="text"]:focus, input[type="password"]:focus, textarea:focus { border: 10px solid yellow; } label{ color:brown; } form{ display: grid; } </style> </head> <body> <h2>Focus Styling In Forms</h2> <p>Place the mouse in each of the input fields to see effect of focus styling</p> <form> <input type="text" id="username" name="username" placeholder="Username"> <input type="password" id="password" name="password" placeholder="password"> <textarea id="message" name="message" rows="4" placeholder="Message"></textarea> <input type="submit" value="Submit"> </form> </body> </html>
CSS Form - Button Styling
Style form buttons using CSS. You can change the button's background color, text color, and add hover effects.
input[type="submit"] { background-color: #007bff; color: #fff; padding: 10px 20px; border: none; cursor: pointer; } input[type="submit"]:hover { background-color: #0056b3; }
Following example demonstrates this:
<html> <head> <style> input[type="text"], input[type="password"], textarea { border: 1px solid red; background-color: antiquewhite; border-radius: 5px; padding: 10px; margin: 5px 0; } input[type="submit"] { background-color: #007bff; color: #fff; padding: 10px 20px; border: none; cursor: pointer; } input[type="submit"]:hover { background-color: #0056b3; } label{ color:brown; } form{ display: grid; } </style> </head> <body> <h2>Form Button Styling</h2> <form> <input type="text" id="username" name="username" placeholder="Username"> <input type="password" id="password" name="password" placeholder="password"> <textarea id="message" name="message" rows="4" placeholder="Message"></textarea> <input type="submit" value="Submit"> </form> </body> </html>
CSS Form - Checkbox and Radio Button Styling
Checkboxes and radio buttons are hard to style, but you can make them visually appealing.
input[type="checkbox"], input[type="radio"] { margin-right: 5px; vertical-align: middle; }
Following example demonstrates this:
<html> <head> <style> form { max-width: 300px; margin: 0 auto; } label { display: block; margin-bottom: 5px; font-size: 20px; color: red; } input[type="radio"], input[type="checkbox"] { margin-right: 5px; vertical-align: middle; } </style> </head> <body> <form> <label>Radio Buttons: </label> <input type="radio" name="gender" value="male"> Male <input type="radio" name="gender" value="female"> Female <label>Checkboxes:</label> <input type="checkbox" name="lang" value="html"> HTML <input type="checkbox" name="lang" value="css"> CSS <input type="checkbox" name="lang" value="bootstrap"> Bootstrap </form> </body> </html>
CSS Form - Form Layout
Use CSS to control the layout of your form elements. You can use techniques like CSS Grid or Flexbox for more complex form layouts.
.form-container { display: grid; grid-template-columns: 1fr 1fr; gap: 20px; }
Example
The following example demonstrates a simple login form where users can enter their login information and click the login button to submit the form −
<html> <head> <style> .form-container { max-width: 300px; margin: 0 auto; padding: 10px; border: 1px solid #ccc; border-radius: 5px; background-color: #f2c3ee; } .form-container input[type="text"], .form-container input[type="password"] { width: 100%; padding: 5px; margin: 10px 0; border: 1px solid #ccc; border-radius: 3px; } .form-container button[type="submit"] { width: 100%; padding: 10px; background-color: #15a415; color: #fff; border: none; border-radius: 3px; } .form-container button[type="submit"]:hover { background-color: #e62b1e; } h1 { color: #15a415; text-align: center; } h2 { color: #e62b1e; text-align: center; } </style> </head> <body> <div class="form-container"> <h1>Tutorialspoint</h1> <h2>Login</h2> <form> <label for="username">Username:</label> <input type="text" id="username" name="username" placeholder="username" required> <label for="password">Password:</label> <input type="password" id="password" name="password" placeholder="password" required> <button type="submit">Login</button> </form> </div> </body> </html>
CSS Form - Box-sizing
To make all form elements of the same size, you can set the box-sizing property to border-box. This property includes the border and padding in the width and height of an element, making it easier to size elements consistently.
Here is an example −
<!DOCTYPE html> <head> <style> .box-sizing-element input,textarea,button { width: 100%; padding: 10px; margin: 0; box-sizing: border-box; } .normal-element input,textarea,button { width: 100%; padding: 10px; margin: 0; } button{ background-color: #04AA6D; border: none; color: white; padding: 16px 32px; text-decoration: none; margin: 4px 2px; cursor: pointer; } </style> </head> <body> <div class="normal-element"> <h3>Without box-sizing</h3> <form> <input type="text" id="name" name="name" placeholder="Enter your name"> <br> <input type="email" id="email" name="email" placeholder="Email id"> <br> <textarea id="message" name="message" rows="4" placeholder="Message"></textarea> <br> <button type="submit" value="Submit">Submit</button> </form> </div> <div class="box-sizing-element"> <h3>With box-sizing</h3> <form> <input type="text" id="name" name="name" placeholder="Enter your name"> <br> <input type="text" id="email" name="email" placeholder="Email id"> <br> <textarea id="message" name="message" rows="4" placeholder="Message"></textarea> <br> <button type="submit" value="Submit">Submit</button> </form> </div> </body> </html>
CSS Form - Fieldset and Legend
The following example demonstrates how to style <fieldset> and <legend> elements. −
<html> <head> <style> form { width : 300px; margin: 0 auto; } fieldset { border: 1px solid #ccc; border-radius: 5px; padding: 10px; } legend { font-weight: bold; text-align: center; color: rgb(15, 141, 15); } </style> </head> <body> <form> <fieldset> <legend>Login</legend> <label for="username">Username:</label> <input type="text" id="username" name="username"> <br> <label for="password">Password:</label> <input type="password" id="password" name="password"> <input type="submit" value="Submit"> </fieldset> </form> </body> </html>
CSS Form - Full Width Input
To make an input field take up the full width of its parent container, you can set its width property of the input field to 100% as shown below −
<html> <head> <style> input.full-width-input { width: 100%; font-size: 16px; color: #545c53; } label { font-weight: bold; font-size: 20px; color: #268219; } </style> </head> <body> <form> <label for="email">Address: </label> <input type="text" id="email" name="email" class="full-width-input" placeholder="Enter address..." required> </form> </body> </html>
CSS Form - Colored Input
The following example shows how to add a background-color to an input field using the background-color property −
<html> <head> <style> input.colored-input { width: 100%; font-size: 16px; color: #545c53; padding: 15px; border: 3px solid #22d90e; background-color: #93ea88; } label { font-weight: bold; font-size: 20px; color: #268219; } </style> </head> <body> <form> <label for="add">Address: </label> <input type="text" id="add" name="add" class="colored-input" placeholder="Enter address..." required> </form> </body> </html>
CSS Form - Images In The Inputs
The following example demonstrates a password input field with a lock icon on the left side. This icon is used to symbolize password input fields in user interfaces −
<html> <head> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css"> <style> .input-container { position: relative; width: 100%; margin-bottom: 20px; } input.password-input { width: 100%; font-size: 16px; color: #545c53; padding: 15px 15px 15px 40px; border: 2px solid #22d90e;; } .password-icon { position: absolute; top: 50%; left: 15px; transform: translateY(-50%); font-size: 20px; color: #22d90e; } </style> </head> <body> <form class="input-container"> <i class="password-icon fas fa-lock"></i> <input type="password" id="password" name="password" class="password-input" placeholder="password" required> </form> </body> </html>
CSS Form - Input With Animation
The following example demonstrates how an input field expands to occupy the full width of its container when it is clicked or focused −
<html> <head> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css"> <style> .input-container { position: relative; width: 100%; margin-bottom: 20px; } input.password-input { width: 50%; font-size: 16px; color: #545c53; padding: 15px 15px 15px 40px; border: 2px solid #22d90e;; } input.password-input:focus { width: 100%; } .password-icon { position: absolute; top: 50%; left: 15px; transform: translateY(-50%); font-size: 20px; color: #22d90e; } </style> </head> <body> <form class="input-container"> <i class="password-icon fas fa-lock"></i> <input type="password" id="password" name="password" class="password-input" placeholder="password" required> </form> </body> </html>
CSS Form - Styling Textareas
Here is an example of a text input area that allows users to enter longer text, such as comments or messages −
<html> <head> <style> textarea { width: 100%; height: 100px; font-size: 16px; color: #938b8b; padding: 15px; border: 3px solid #22d90e; } textarea:focus { box-shadow: 0 0 10px #db59ab; } </style> </head> <body> <form> <textarea>Enter text here....</textarea> </form> </body> </html>
CSS Form - Styling Dropdown Menus
The following example demonstrates how to style a dropdown menu that is hidden by default. When the user clicks on the trigger element, the dropdown menu will open and display the list of items −
<html> <head> <style> select { width: 100%; font-size: 16px; color: #000000; padding: 15px; border: 3px solid #147309; background-color: #93ea88; } </style> </head> <body> <form> <select aria-placeholder="Select Language"> <option value="lang" disabled selected>Select Language</option> <option value="html">HTML</option> <option value="css">CSS</option> <option value="bootstrap">Bootstrap</option> </select> </form> </body> </html>
Responsive Form Layout
Here is an example of a simple responsive form layout. Check this form on different devices to see the responsiveness.−
<html> <head> <style> .container { max-width: 400px; margin: 0 auto; padding: 20px; background-color: #c5e08e; border-radius: 5px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); } h1 { text-align: center; margin-bottom: 20px; color: #34892b; } label { display: block; font-weight: bold; font-size: 15px; margin-bottom: 5px; color: #f25820; } input[type="text"], input[type="email"], select { width: 100%; padding: 15px; margin-bottom: 15px; border: 2px solid #ccc; border-radius: 10px; background-color: #eff5f5; } input[type="submit"] { width: 100%; padding: 10px; background-color: #216e2a; color: #fff; border: none; font-size: 20px; border-radius: 4px; cursor: pointer; } textarea { width: 100%; height: 100px; padding: 15px; margin-bottom: 15px; border: 2px solid #ccc; border-radius: 10px; background-color: #eff5f5; } input[type="radio"] { margin-right: 5px; vertical-align: middle; margin-bottom: 10px; } input[type="submit"]:hover { background-color: #44d115; color: #000000; } </style> </head> <body> <div class="container"> <h1>Registration Form</h1> <form> <label for="name">Name:</label> <input type="text" id="name" name="name" placeholder="Enter Name..." required> <label for="email">Email:</label> <input type="email" id="email" name="email" placeholder="Enter Email Id..." required> <label for="email">Address:</label> <textarea>Address...</textarea> <label>Gender: </label> <input type="radio" name="gender" value="male"> Male <input type="radio" name="gender" value="female"> Female <label for="gender">Langauges:</label> <select id="gender" name="gender"> <option value="lang" disabled selected>Select Gender</option> <option value="male">Hindi</option> <option value="female">Marathi</option> <option value="other">English</option> </select> <input type="submit" value="Submit"> </form> </div> </body> </html>