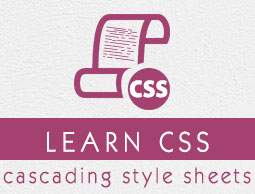
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - place-content
CSS place-content is a shorthand property that aligns content in both the block (column) and inline (row) axes simultaneously. It is used to set both the align-content and justify-content properties in a single declaration.
This property is a shorthand for the following CSS properties:
Possible Values
start − Items are aligned to each other against the start edge of the container in the corresponding axis.
end − Items are aligned to each other against the end edge of the container in the corresponding axis.
flex-start − Aligns the items at the start of the flex container.
flex-end − Aligns the items at the end of the flex container.
center − Aligns the items at the center of the flex container.
left − Aligns the items to the left side of the alignment container. This value acts like start if the property's axis is not parallel to the inline axis.
right − Aligns the items to the right edge of the alignment container in the appropriate axis. This value acts like start if the property's axis is not parallel to the inline axis.
space-between − Items within the alignment container are evenly distributed, with equal spacing between adjacent items, with thefirst and last items are aligned with the main-start and main-end edge.
baseline, first baseline, last baseline −
These values specify the involvment of first or last baseline alignment in the alignment of the content.
First and last baseline are synonym to baseline. First and last refer to the line boxes within the flex items.
The start is the fallback alignment for first-baseline and end for last-baseline.
space-around − Items within the alignment container are evenly distributed. Each pair of adjacent elements has the same spacing before the first and last items is half the distance between adjacent items.
space-evenly − Items within the alignment container are evenly distributed, with equal spacing between adjacent items and at the main-start and main-end edges.
stretch − If the total size of items along the main axis is smaller than the alignment container, auto-sized items increase their size equally to fill the container, respecting max-height/max-width constraints.
safe − Used with an alignment keyword and when the item overflows the container, causing any loss of data, the alignment is set as per the start value.
unsafe − Used with an alignment keyword and even if the item overflows the container, causing any loss of data, the alignment value passed is honored.
Applies To
Multi-line flex containers.
Syntax
Positional Alignment
place-content: center start; place-content: start center; place-content: end left; place-content: flex-start center; place-content: flex-end center;
Baseline Alignment
place-content: baseline center; place-content: first baseline space-evenly; place-content: last baseline right;
Distributed Alignment
place-content: space-between space-evenly; place-content: space-around space-evenly; place-content: space-evenly stretch; place-content: stretch space-evenly;
The first property value is align-content and the second is justify-content.
If the second value is missing, the first value is used for both, as long as it is valid. If it is invalid for both, the entire value is invalid.
CSS place-content - center start Value
The following example demonstrates property place-content: center start aligns flex items horizontally towards the center and vertically towards the top of the container −
<html> <head> <style> .flex-container { background-color: red; height: 300px; border: 2px solid black; display: flex; flex-wrap: wrap; place-content: center start; } .flex-container > div { flex-basis: 100px; height: 50px; margin: 5px; background-color: greenyellow; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> </div> </body> </html>
CSS place-content - start center Value
The following example demonstrates property place-content: start center aligns flex items along the left edge of the container horizontally and vertically in the center of the container −
<html> <head> <style> .flex-container { background-color: red; height: 300px; border: 2px solid black; display: flex; flex-wrap: wrap; place-content: start center; } .flex-container > div { flex-basis: 100px; height: 50px; margin: 5px; background-color: greenyellow; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> </div> </body> </html>
CSS place-content - end right Value
The following example demonstrates property place-content: end right aligns the flex items to the right edge horizontally and the bottom edge vertically −
<html> <head> <style> .flex-container { background-color: red; height: 300px; border: 2px solid black; display: flex; flex-wrap: wrap; place-content: end right; } .flex-container > div { flex-basis: 100px; height: 50px; margin: 5px; background-color: greenyellow; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> </div> </body> </html>
CSS place-content - flex-start center Value
The following example demonstrates property place-content: flex-start center aligns the flex items horizontally towards the left edge and vertically towards the center of the container −
<html> <head> <style> .flex-container { background-color: red; height: 300px; border: 2px solid black; display: flex; flex-wrap: wrap; place-content: flex-start center; } .flex-container > div { flex-basis: 100px; height: 50px; margin: 5px; background-color: greenyellow; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> </div> </body> </html>
CSS place-content - flex-end center Value
The following example demonstrates property place-content: flex-end center aligns the flex items horizontally towards the right end and vertically towards the center of the container −
<html> <head> <style> .flex-container { background-color: red; height: 300px; border: 2px solid black; display: flex; flex-wrap: wrap; place-content: flex-end center; } .flex-container > div { flex-basis: 100px; height: 50px; margin: 5px; background-color: greenyellow; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> </div> </body> </html>
CSS place-content - last baseline Value
The following example demonstrates property place-content: last baseline property the last line of items aligns to the baseline of the highest item on that line −
<html> <head> <style> .flex-container { background-color: red; height: 300px; border: 2px solid black; display: flex; flex-wrap: wrap; place-content: last baseline; } .flex-container > div { flex-basis: 100px; height: 50px; margin: 5px; background-color: greenyellow; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> </div> </body> </html>
CSS place-content - space-between Value
The following example demonstrates property place-content: space-between property evenly distributes space between the flex items inside the flex container −
<html> <head> <style> .flex-container { background-color: red; height: 300px; border: 2px solid black; display: flex; flex-wrap: wrap; place-content: space-between; } .flex-container > div { flex-basis: 100px; height: 50px; margin: 5px; background-color: greenyellow; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> </div> </body> </html>
CSS place-content - space-around Value
The following example demonstrates property place-content: space-around property evenly distributes space around the flex items inside the flex container −
<html> <head> <style> .flex-container { background-color: red; height: 300px; border: 2px solid black; display: flex; flex-wrap: wrap; place-content: space-around; } .flex-container > div { flex-basis: 100px; height: 50px; margin: 5px; background-color: greenyellow; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> </div> </body> </html>
CSS place-content - space-evenly Value
The following example demonstrates property place-content: space-evenly property evenly distributes space evenly (between and around) the flex items inside the flex container −
<html> <head> <style> .flex-container { background-color: red; height: 300px; border: 2px solid black; display: flex; flex-wrap: wrap; place-content: space-around; } .flex-container > div { flex-basis: 100px; height: 50px; margin: 5px; background-color: greenyellow; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> </div> </body> </html>