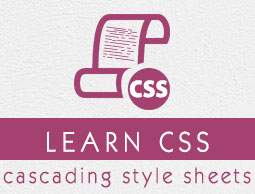
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Image Sprites
Image sprites are a technique used in web development to combine multiple images into a single image file. This approach can help reduce the number of server requests and improve website performance.
Image sprites are commonly used for icons, buttons, and other small graphics on a website. CSS is then used to display specific parts of the sprite image as needed.
Here's a step-by-step guide on how to create and use image sprites in CSS:
Step 1: Create The Sprite Image
Create a single image file that contains all the individual images (icons, buttons, etc.) you want to use on your website. You can use software like Photoshop or a similar tool to arrange these images into a single image file.
Save the sprite image in a suitable format like PNG or JPEG. Make sure the individual images within the sprite are well-organized with consistent spacing between them.
Step 2: Add HTML Markup
In your HTML document, you'll need to add elements that will display the individual images from the sprite. Typically, you'll use HTML elements like <div> or <span> for this purpose. Here's an example:
<html> <head> <!-- CSS styling here --> </head> <body> <div class="sprite-icon"></div> </body> </html>
Step 3: Define CSS Classes
In your CSS file / style tag (inline styling), define classes for each element that uses the sprite image. You'll set the background-image to your sprite image and specify the background-position to display the desired part of the sprite. Here's an example:
.sprite-icon { width: 32px; /* Set the width of the displayed image */ height: 32px; /* Set the height of the displayed image */ background-image: url('sprites.png'); /* Path to your sprite image */ background-position: -16px -16px; /* Position of the individual image within the sprite */ }
In the above example, the background-position property is used to specify which part of the sprite image to display. The values (-16px, -16px) represent the position of the desired image within the sprite. By adjusting these values, you can display different images from the sprite.
Step 4: Use The Sprites In HTML
You can now use the CSS classes you defined in your HTML elements to display the individual images from the sprite:
<div class="sprite-icon"></div>
Repeat this process for each element that needs to display an image from the sprite.
CSS Image Sprites - Basic Example
The following example demonstrates how to use CSS image sprites to display multiple images from a single image file.
<html> <head> <style> .orignal-img { width: 300px; height: 100px; } ul { list-style: none; padding: 0; } li { height: 150px; display: block; } li a { display: block; height: 100%; } .bootstrap, .html, .css { width: 150px; height: 150px; background-image: url('images/devices.png'); background-repeat: no-repeat; } .bootstrap { background-position: -5px -5px; } .html { background-position: -155px -5px; } .css { background-position: -277px -5px; } </style> </head> <body> <h3>Orignal Image</h3> <img class="orignal-img" src="images/devices.png"/> <h3>After implementing CSS image sprites</h3> <ul class="navbar"> <li><a href="#" class="bootstrap"></a></li> <li><a href="#" class="html"></a></li> <li><a href="#" class="css"></a></li> </ul> </body> </html>
CSS Image Sprites - Hover Effect
The following example demostartes how to use image sprites to create a hover effect where the images become slightly transparent when you hover over them −
<html> <head> <style> .orignal-img { width: 300px; height: 100px; } ul { list-style: none; padding: 0; } li { height: 150px; display: block; } li a { display: block; height: 100%; } .bootstrap, .html, .css { width: 150px; height: 150px; background-image: url('images/devices.png'); background-repeat: no-repeat; } .bootstrap { background-position: -5px -5px; } .html { background-position: -155px -5px; } .css { background-position: -277px -5px; } .bootstrap:hover, .html:hover, .css:hover { opacity: 0.7; } </style> </head> <body> <h3>Orignal Image</h3> <img class="orignal-img" src="images/devices.png"/> <h3>After implementing CSS image sprites</h3> <ul class="navbar"> <li><a href="#" class="bootstrap"></a></li> <li><a href="#" class="html"></a></li> <li><a href="#" class="css"></a></li> </ul> </body> </html>