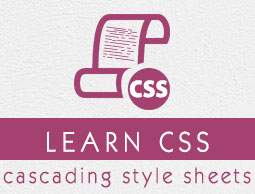
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Navigation Bar
A navigation bar is a section of a graphical user interface (GUI) that helps users navigate through a website, app, or other software. It is essential for users to quickly and easily navigate to the content they are looking for.
The navigation bar can be horizontal or vertical, that contains links to important pages or features.
Navbars can also contain other elements, such as the logo of the website or app, search bar, or social media icons. Navbars can be styled using CSS to change their appearance.
CSS Horizontal Navbar
Following example shows a horizontal navigation bar, which is the most common type of navigation bar displayed across the top of a web page as shown below −
<html> <head> <style> ul { background-color: #28bf17; overflow: hidden; list-style-type: none; margin: 0; padding: 0; } li { float: left; } li a { display: block; color: #f2f2f2; text-align: center; padding: 10px; text-decoration: none; font-size: 17px; } li a:hover { background-color: #dd9ede; color: black; } .active-link { background-color: #f53319; color: white; } </style> </head> <body> <ul> <li><a href="#" class="active-link">Tutorialspoint</a></li> <li><a href="#">Home</a></li> <li><a href="#">Articles</a></li> <li><a href="#">Courses</a></li> <li><a href="#">eBook</a></li> </ul> <h1>Welcome to TutorialsPoint</h1> <h3>Simple Easy Learning at your fingertips</h3> </body> </html>
CSS Vertical Navbar
A vertical navigation bar is also known as a sidebar menu. It is typically positioned on the left or right side of the screen.
Here is an example −
<html> <head> <style> ul { background-color: #28bf17; list-style-type: none; margin: 0; padding: 0; width: 200px; } li { text-align: center; } li a { display: block; color: #f2f2f2; text-align: center; padding: 10px; text-decoration: none; font-size: 17px; } li a:hover { background-color: #dd9ede; color: black; } .active-link { background-color: #f53319; color: white; } </style> </head> <body> <ul> <li><a href="#" class="active-link">Tutorialspoint</a></li> <li><a href="#">Home</a></li> <li><a href="#">Articles</a></li> <li><a href="#">Courses</a></li> <li><a href="#">eBook</a></li> </ul> </body> </html>
CSS Dropdown Navbar
A dropdown navbar is a navigation bar with a drop-down menu that appears when a user hovers over a link. Dropdown menus are a way to show a list of related items in a small space.
Here is an example −
<html> <head> <style> .navbar { background-color: #28bf17; overflow: hidden; } .navbar a { display: block; float: left; color: #f2f2f2; text-align: center; padding: 10px; text-decoration: none; font-size: 15px; } .navbar a:hover { background-color: #dd9ede; color: black; } .active-link { background-color: #f53319; color: white; } .dropdown { float: left; } .dropdown .dropbtn { border: none; color: #f2f2f2; padding: 10px; background-color: #28bf17; } .dropdown-content { display: none; position: absolute; background-color: #c7385a; min-width: 120px; } .dropdown-content a { float: none; color: #f2f2f2; padding: 10px; display: block; text-align: left; } .dropdown-content a:hover { background-color: #dd9ede; color: black; } .dropdown:hover .dropdown-content { display: block; } </style> </head> <body> <nav class="navbar"> <a href="#" class="active-link">Tutorialspoint</a> <a href="#">Home</a> <div class="dropdown"> <button class="dropbtn">Articles</button> <div class="dropdown-content"> <a href="#">HTML</a> <a href="#">CSS</a> <a href="#">Bootstrap</a> </div> </div> <a href="#">Courses</a> <a href="#">eBook</a> </nav> <h1>Welcome to TutorialsPoint</h1> <h3>Simple Easy Learning at your fingertips</h3> </body> </html>
CSS Fixed Navbar
A fixed navbar is a navigation bar that sticks to the top of the screen when the user scrolls down the page. To make a navbar fixed, you can use the position: fixed property.
Here is an example −
<html> <head> <style> body { margin: 0; } .navbar { position: fixed; top: 0; width: 100%; margin: 0; padding: 0px; overflow: hidden; background-color: #28bf17; } .navbar a { display: block; float: left; color: #f2f2f2; text-align: center; padding: 10px; text-decoration: none; font-size: 17px; } .navbar a:hover { background-color: #dd9ede; color: black; } .active-link { background-color: #f53319; color: white; } .heading { padding-top: 170px; text-align: center; background-color: #e6e451; padding-bottom: 300px } </style> </head> <body> <nav class="navbar"> <a href="#" class="active-link">Tutorialspoint</a> <a href="#">Home</a> <a href="#">Articles</a> <a href="#">Courses</a> <a href="#">eBook</a> </nav> <div class="heading"> <h1>Welcome to TutorialsPoint</h1> <h2>Tutorialspoint CSS Fixed Navbar</h2> <h3>Simple Easy Learning at your fingertips</h3> </div> </body> </html>
CSS Sticky Navbar
You can use the position: sticky property to create a sticky navbar, which will stay at the top of the screen even when the user scrolls down the page.
Here is an example −
<html> <head> <style> body { margin: 0; } .navbar { position:sticky; top: 0; width: 100%; margin: 0; padding: 0px; overflow: hidden; background-color: #28bf17; } .navbar a { display: block; float: left; color: #f2f2f2; text-align: center; padding: 10px; text-decoration: none; font-size: 17px; } .navbar a:hover { background-color: #dd9ede; color: black; } .active-link { background-color: #f53319; color: white; } .heading { padding-top: 170px; text-align: center; background-color: #e6e451; padding-bottom: 300px } h2 { text-align: center; } </style> </head> <body> <h2>Scroll down to see the effect</h1> <nav class="navbar"> <a href="#" class="active-link">Tutorialspoint</a> <a href="#">Home</a> <a href="#">Articles</a> <a href="#">Courses</a> <a href="#">eBook</a> </nav> <div class="heading"> <h1>Welcome to TutorialsPoint</h1> <h2>Tutorialspoint CSS Sticky Navbar</h2> <h3>Simple Easy Learning at your fingertips</h3> </div> </body> </html>
Divider Lines for Navbar
You can also add a divider line between the links in the navbar using the border-right property as shown below −
<html> <head> <style> .navbar { background-color: #28bf17; overflow: hidden; } .navbar a { display: block; float: left; color: #f2f2f2; text-align: center; padding: 10px; text-decoration: none; font-size: 17px; border-right: 2px solid #f013c8; } .navbar a:hover { background-color: #dd9ede; color: black; } .active-link { background-color: #f53319; color: white; } </style> </head> <body> <nav class="navbar"> <a href="#" class="active-link">Tutorialspoint</a> <a href="#">Home</a> <a href="#">Articles</a> <a href="#">Courses</a> <a href="#">eBook</a> </nav> <h1>Welcome to TutorialsPoint</h1> <h2>Tutorialspoint CSS Navbar with borders</h2> </body> </html>
Fixed Vertical Navbar
The following example demonstrates how the position: fixed property can be used to create a fixed vertical navbar, which ensures that the navbar stays on the left side of the screen, even when the user scrolls down the page.
<html> <head> <style> ul { position: fixed; background-color: #28bf17; list-style-type: none; margin: 0; padding: 0; width: 200px; height: 100%; } li { text-align: center; border-bottom: 2px solid #f013c8; } li a { display: block; color: #f2f2f2; text-align: center; padding: 10px; text-decoration: none; font-size: 17px; } li a:hover { background-color: #dd9ede; color: black; } .active-link { background-color: #f53319; color: white; } .heading { padding-top: 170px; text-align: center; background-color: #e6e451; padding-bottom: 300px } </style> </head> <body> <ul> <li><a href="#" class="active-link">Tutorialspoint</a></li> <li><a href="#">Home</a></li> <li><a href="#">Articles</a></li> <li><a href="#">Courses</a></li> <li><a href="#">eBook</a></li> </ul> <div class="heading"> <h1>Welcome to TutorialsPoint</h1> <h2>Tutorialspoint CSS Fixed Vertical Navbar</h2> <h3>Simple Easy Learning at your fingertips</h3> </div> </body> </html>