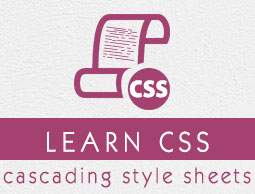
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Variables
CSS variables are custom properties that allows you to store and reuse values throughout your CSS program. CSS variables are similar to variables in other programming languages.
They are set using the -- prefix, and accessed using the var() function.
To declare a CSS variable, use the following syntax:
--variable-name: value;
To use a CSS variable with var() function, use the following syntax:
var(--variable-name, fallback-value);
Custom properties are affected by the CSS cascade and inherit their value from their parent element.
CSS variables cannot be used in media queries or container queries, but you can use the var() function to replace values within CSS properties on an element. You cannot use them to set the name of a CSS property or selector, or to define a media query or container query.
CSS Variables - Standard Approach
The following example demonstrates CSS that applies the same color to elements of different classes −
<html> <head> <style> .box { width: 400px; height: 200px; text-align: center; line-height: 35px; margin: 10px; background-color: pink; color: blue; } .box1 { display: inline-block; background-color: yellow; width: 100px; height: 50px; color: blue; } .box2 { display: inline-block; background-color: yellow; width: 100px; height: 50px; color: blue; } </style> </head> <body> <div class="box"> <h2>Tutorialspoint</h2> <p>How to code a website using HTML and CSS</p> <div class="box1">HTML</div> <div class="box2">CSS</div> </div> </body> </html>
CSS Variables - :root Pseudo-class
CSS variables are declared at the top of the stylesheet using the :root selector, which matches the root element of the document. This means that CSS variables declared using the :root selector can be used by any element in the document.
CSS variable names are case-sensitive, which means that --pink-color and --Pink-color are two different variables.
Step-1: Define the custom properties
To declare variables using the :root pseudo-class, you simply add the -- prefix to the variable name and then set its value.
:root { --pink-color: pink; --blue-color: blue; }
Step-2: Use the custom properties in the CSS
These variables can then be used throughout your CSS code using the var() function.
.box { width: 400px; height: 200px; background-color: var(--pink-color); color: var(--blue-color); } .box1, .box2 { display: inline-block; background-color: var(--pink-color); width: 100px; height: 50px; color: var(--blue-color); }
The following example demonstrates that the CSS variables --pink-color and --blue-color are declared in the :root element, which means they are global custom properties that can be used anywhere in the CSS code −
<html> <head> <style> :root { --pink-color: pink; --blue-color: blue; } .box { width: 400px; height: 200px; text-align: center; line-height: 35px; margin: 10px; background-color: var(--pink-color); color: var(--blue-color); } .box1, .box2 { display: inline-block; border: 3px solid yellow; background-color: var(--pink-color); width: 100px; height: 50px; color: var(--blue-color); } </style> </head> <body> <div class="box"> <h2>Tutorialspoint</h2> <p>How to code a website using HTML and CSS</p> <div class="box1">HTML</div> <div class="box2">CSS</div> </div> </body> </html>
CSS Variables - Inheritance of Custom Properties
You can use CSS variables to define reusable CSS values that can be inherited by child elements.
Step-1: Declare the custom property in the :root selector.
This makes the variable global and accessible to all elements in the document.
:root { --pink-color: pink; }
Step-2: Use the var() function to access the custom property in the CSS.
This allows you to reuse the custom property throughout your CSS code.
.box { --box-background: var(--pink-color); background-color: var(--box-background); }
Step-3: Override the custom property value for specific elements using the -- prefix.
This allows you to customize the value of the custom property for specific elements.
.box1, .box2 { background-color: var(--box-background); }
The following example demonstrates that the use of CSS custom properties with inheritance −
<html> <head> <style> :root { --pink-color: pink; } .box { --box-background: var(--pink-color); color: black; width: 400px; height: 200px; text-align: center; line-height: 35px; margin: 10px; background-color: var(--box-background); } .box1, .box2 { color: blue; display: inline-block; border: 3px solid yellow; background-color: var(--box-background); width: 100px; height: 50px; } </style> </head> <body> <div class="box"> <h2>Tutorialspoint</h2> <p>How to code a website using HTML and CSS</p> <div class="box1">HTML</div> <div class="box2">CSS</div> </div> </body> </html>
CSS Variables - Custom Property Fallback Value
You can use CSS variables with fallback values to ensure that your CSS code is still valid and works even if the variable is not defined.
CSS fallback values are not used to make CSS custom properties work in older browsers. They are only used as a backup in browsers that support CSS custom properties, in case the variable is not defined or has an invalid value.
CSS variable fallback values can be specified using commas. For example, the var(--pink-color, blue, pink) function defines a fallback value of blue, pink. The fallback value is the text that appears between the first comma and the end of the function.
In below example, If the --pink-color or --blue-color custom properties are not defined, the fallback values of red and green will be used, respectively.
Here is an example −
<html> <head> <style> :root { --pink-color: pink; --blue-color: blue; } .box { width: 400px; height: 200px; text-align: center; line-height: 35px; margin: 10px; background-color: var(--pink-color, red); color: var(--blue-color, green ); } .box1, .box2 { display: inline-block; border: 3px solid yellow; background-color: var(--pink-color, red); width: 100px; height: 50px; color: var(--grey-color, brown); } </style> </head> <body> <div class="box"> <h2>Tutorialspoint</h2> <p>How to code a website using HTML and CSS</p> <div class="box1">HTML</div> <div class="box2">CSS</div> </div> </body> </html>
CSS Variables - Handling Invalid Custom Properties
In below example, the --red-color custom property is defined with a value of 15px. This is an invalid value because the red color property only accepts color values.
However, the browser will not be able to resolve the value of the custom property because it is invalid. As a result, it will simply ignore the CSS rule and the text in the second h2 element will remain the same color.
Here is an example −
<html> <head> <style> :root { --red-color: 15px; } h2 { color: red; } h2 { color: var(--red-color); } </style> </head> <body> <h2>Tutorialspoint CSS Variables.</h2> </body> </html>
CSS Variables - Values in Javascript
The following example demonstartes that how to use JavaScript to set CSS custom properties −
<html> <head> <style> .box { width: 400px; height: 200px; text-align: center; line-height: 35px; margin: 10px; background-color: var(--pink-color); color: var(--blue-color); } .box1, .box2 { display: inline-block; border: 3px solid yellow; width: 100px; height: 50px; color: var(--blue-color); } </style> </head> <body> <div class="box"> <h2>Tutorialspoint</h2> <p>How to code a website using HTML and CSS</p> <div class="box1">HTML</div> <div class="box2">CSS</div> </div> <script> const boxElement = document.querySelector('.box'); const jsPinkColor = 'pink'; const jsBlueColor = 'blue'; boxElement.style.setProperty('--pink-color', jsPinkColor); boxElement.style.setProperty('--blue-color', jsBlueColor); </script> </body> </html>