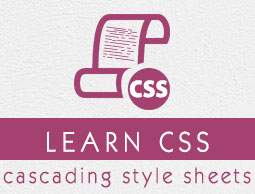
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Custom Properties
Custom properties use names that start with --, such as --color-name. These properties can store values, which can then be used in other elements using the var() function.
Custom properties are specific to elements, and their values follow the cascading rules, the value of a custom property is determined by the cascading algorithm.
Possible Values
<declaration-value> − This value can be any combination of tokens, but it must not include certain disallowed tokens. It specifies what a valid declaration can have as its value.
Applies To
All the HTML elements.
Syntax
--keywordValue: right; --colorValue: #e74c3c; --complexValue: 5px 10px rgb(238, 50, 17);
When defining custom properties with "--", remember they are case sensitive. "--my-color" and "--My-color" will not have the same value, they are separate variables.
CSS Custom Properties - Commas in Values
The following syntax shows how to use multiple values using commas. The transform: scale(var(--scale, 1.1, 1.5)) property defines the first comma, which separates the fallback, and the second comma, which is a value −
button { transform: scale(var(--scale, 1.1, 1.5)); }
CSS Custom Properties
The following example demonstrates the use of custom properties −
<html> <head> <style> :root { --red-color: red; --green-color: rgb(125, 226, 31); } div { width: 200px; height: 100px; margin: 10px; } .box1 { background-color: var(--green-color); color: var(--red-color); } .box2 { background-color: var(--red-color); color: var(--green-color); } .box3 { --pink-color: rgb(247, 30, 193); } .box3 p { color: var(--pink-color); } </style> </head> <body> <div class="box1"> Green Background, Red Text </div> <div class="box2"> Red Background, Green Text </div> <div class="box3"> <p>Pink Text</p> </div> </body> </html>
CSS Custom Properties - Setting Value
The following example demonstrates that custom property's value can be set using another custom property −
<html> <head> <style> html { --red-color: #e92424; --green-color: #2c1fdd; --yellow-color: #f6f867; --back: var(--yellow-color); --para: var(--green-color); --border: var(--red-color); } div { width: 200px; height: 150px; padding: 10px; background-color: var(--back); border: 3px solid var(--border); } h3 { color: var(--green-color); text-align: center; } p { color: var(--para); } </style> </head> <body> <div> <h3>Tutorialspoint</h3> <p>Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text.</p> </div> </body> </html>
CSS Custom Properties - Splitting Colors
The following example demonstrates that when you hover over the box, the background color is changed by modifying the values of --h, --s, --l, and --a −
<html> <head> <style> .box { width: 150px; width: 150px; padding: 10px; --h: 0; /* hue */ --s: 70%; /* saturation */ --l: 50%; /* lightness */ --a: 1; /* alpha */ background-color: hsl(var(--h) var(--s) var(--l) / var(--a)); color: white; } .box:hover { --l: 75%; } .box:focus { --s: 75%; } .box[disabled] { --s: 0%; --a: 0.5; } </style> </head> <body> <div class="box"> Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text. </div> </body> </html>
CSS Custom Properties - Shadow
The following example demonstrates box shadow effect with --shadow value. The box shadow is initially 2px, but when you hover over it, the shadow increases to 10px −
<html> <head> <style> .box { width: 150px; width: 150px; padding: 10px; margin: 30px; --shadow: 2px; background-color: aqua; box-shadow: 0 0 20px var(--shadow) red; } .box:hover { --shadow: 10px; } </style> </head> <body> <div class="box"> Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text. </div> </body> </html>
CSS Custom Properties - Gradients
The following example demonstrates that the box with gradient background transitioning from green to yellow to red. The gradient angle is initially 90deg, but when you hover over it, gradient angle changes to 180deg −
<html> <head> <style> .box { width: 150px; width: 150px; padding: 10px; margin: 30px; --gradient-angle: 90deg; background: linear-gradient(var(--gradient-angle), green, yellow, red); } .box:hover { --gradient-angle: 180deg; } </style> </head> <body> <div class="box"> Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text. </div> </body> </html>
CSS Custom Properties - Grid
The following example demonstrates the grid layout where the width of column changes based on the the screen width. The value of the --boundary is initially 30px, but when you resize the browser window, the --boundary value changes to the 40% of the container width −
<html> <head> <style> .box { background-color: lightcoral; display: grid; --boundary: 30px; grid-template-columns: var(--boundary) 1fr var(--boundary); } @media (max-width: 800px) { .box { --boundary: 40%; } } </style> </head> <body> <div class="box"> Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text. </div> </body> </html>
CSS Custom Properties - Transforms
The following example demonstrates the transform effect using custom properties. When you hover over a button, it scales down to 80% of its original size, and when you click it, it moves down 3px −
<html> <head> <style> button { transform: var(--scale-button, scale(1)) var(--translate-button, translate(0)); padding: 10px; background-color: yellow; } button:active { --translate-button: translate(0, 3px); } button:hover { --scale-button: scale(0.8); } </style> </head> <body> <button> Tutorialspoint </button> </body> </html>
CSS Custom Properties - Concatenation of Unit Types
The following example demonstrates how to use CSS custom properties to dynamically set the font size. The calc() function calculates the size by multiplying the custom properties --size-val and --pixel-values −
<html> <head> <style> html { --size-val: 24; --unit-val: px; font-size: var(--size-val) + var(--unit-val); font-size: calc(var(--size-val) * 1px); --pixel-values: 1px; font-size: calc(var(--size-val) * var(--pixel-values)); } .box { width: 200px; height: 150px; background-color: yellowgreen; } </style> </head> <body> <div class="box"> Lorem Ipsum is simply dummy text of the printing and typesetting industry. </div> </body> </html>
CSS Custom Properties - Using the Cascade
The following example demonstrates that how to use CSS custom properties with cascade. The first box uses default global --background-color value (yellow), and second box uses the local --background-color value (lightblue) −
<html> <head> <style> html { --background-color: yellow; --color: red; } .container { --background-color: lightblue; } .box { background: var(--background-color); } div { height: 100px; width: 100px; margin: 10px; } </style> </head> <body> <div class="box"> Yellow background color box. </div> <div class="container"> <div class="box"> Blue background color box. </div> </div> </body> </html>
CSS Custom Properties - :root
The following example demonstrates how to set custom properties with :root selector. The :root selector matches as high up as they can go −
<html> <head> <style> :root { --yellow: yellow; } .box { background-color: var(--yellow); } </style> </head> <body> <div class="box"> Lorem Ipsum is simply dummy text of the printing and typesetting industry. </div> </body> </html>
CSS Custom Properties - Combining With !important
The following example demonstrates applying !important to the --background-color variable, it is difficult to override the value of the --background-color variable −
<html> <head> <style> .box { --background-color: yellow !important; background-color: var(--background-color); --text: red; color: var(--text); } </style> </head> <body> <div class="box"> Lorem Ipsum is simply dummy text of the printing and typesetting industry. </div> </body> </html>
CSS Custom Properties - Fallbacks
The following example demonstrates scale transformation effect on the button. The scaling factor is specified using the --scale custom property. If --scale is not defined, the scale set to 1.2 −
<html> <head> <style> button { transform: scale(var(--scale, 1.2)); padding: 10px; background-color: yellow; margin: 5px; } button:hover { --scale: scale(0.8); } </style> </head> <body> <button> Hover Me </button> </body> </html>
CSS Custom Properties - @property
The following example demonstrates the use of @property rule to define custom property --gradient-color with initial value pink and transition effect. When you hover over the box, gradient color changes to green color −
<html> <head> <style> @property --gradient-color { initial-value: pink; inherits: false; } .box { width: 150px; height: 150px; --gradient-color: pink; background: linear-gradient(var(--gradient-color), yellow); transition: --gradient-color 1s; } .box:hover { --gradient-color: green; } </style> </head> <body> <div class="box"> Lorem Ipsum is simply dummy text of the printing and typesetting industry. </div> </body> </html>