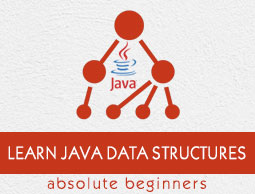
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Verifying if the Vector is empty
The Vector class of the java.util package provides a isEmpty() method. This method verifies whether the current vector is empty or not. If the given vector is empty this method returns true else it returns false.
Example
import java.util.Vector; public class Vector_IsEmpty { public static void main(String args[]) { Vector vect = new Vector(); vect.addElement("Java"); vect.addElement("JavaFX"); vect.addElement("HBase"); vect.addElement("Neo4j"); vect.addElement("Apache Flume"); System.out.println("Elements of the vector :"+vect); boolean bool1 = vect.isEmpty(); if(bool1==true) { System.out.println("Given vector is empty"); } else { System.out.println("Given vector is not empty"); } vect.clear(); boolean bool2 = vect.isEmpty(); System.out.println("cleared the contents of the vector"); if(bool2==true) { System.out.println("Given vector is empty"); } else { System.out.println("Given vector is not empty"); } } }
Output
Elements of the vector :[Java, JavaFX, HBase, Neo4j, Apache Flume] Given vector is not empty cleared the contents of the vector Given vector is empty
Advertisements
To Continue Learning Please Login