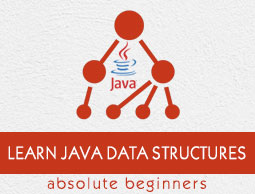
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Searching for values in a tree
To search whether the given tree contains a particular element. Compare it with every element down the tree if found display a message saying element found.
Example
class Node{ int data; Node leftNode, rightNode; Node() { leftNode = null; rightNode = null; this.data = data; } Node(int data) { leftNode = null; rightNode = null; this.data = data; } int getData() { return this.data; } Node getleftNode() { return this.leftNode; } Node getRightNode() { return this.leftNode; } void setData(int data) { this.data = data; } void setleftNode(Node leftNode) { this.leftNode = leftNode; } void setRightNode(Node rightNode) { this.leftNode = rightNode; } } public class SeachingValue { public static void main(String[] args) { Node node = new Node(50); node.leftNode = new Node(60); node.leftNode.leftNode = new Node(45); node.leftNode.rightNode = new Node(64); node.rightNode = new Node(60); node.rightNode.leftNode = new Node(45); node.rightNode.rightNode = new Node(64); System.out.println("Pre order of the above created tree :"); preOrder(node); System.out.println(); int data = 60; boolean b = find(node, data); if(b) { System.out.println("Element found"); } else { System.out.println("Element not found"); } } public static void preOrder(Node root) { if(root !=null) { System.out.print(root.data+" "); preOrder(root.leftNode); preOrder(root.rightNode); } } public static boolean find(Node root, int data) { if(root == null) { return false; } if(root.getData() == data) { return true; } return find(root.getleftNode(), data)||find(root.getRightNode(), data); } }
Output
Pre order of the above created tree : 50 60 45 64 60 45 64 Element found
Advertisements
To Continue Learning Please Login