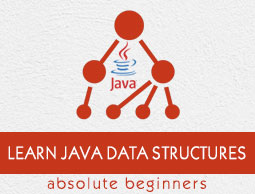
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Pushing elements to a Stack
Push operation in the a stack involves inserting elements to it. If you push a particular element to a stack it will be added to the top of the stack. i.e. the first inserted element in the stack is the last one to be popped out. (First in last out)
You can push an element into Java stack using the push() method.
Example
import java.util.Stack; public class PushingElements { public static void main(String args[]) { Stack stack = new Stack(); stack.push(455); stack.push(555); stack.push(655); stack.push(755); stack.push(855); stack.push(955); System.out.println(stack); } }
Output
[455, 555, 655, 755, 855, 955]
Advertisements
To Continue Learning Please Login