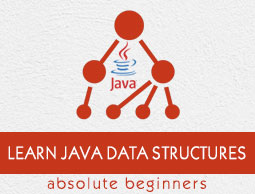
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Printing the elements of the Vector
You can print all the elements of a vector using the println statement directly.
System.out.println(vect);
Or, you can prints its elements one by one using the methods hasMoreElements() and nextElement().
Example
import java.util.*; public class VectorPrintingElements { public static void main(String args[]) { // initial size is 3, increment is 2 Vector v = new Vector(); v.addElement(new Integer(1)); v.addElement(new Integer(2)); v.addElement(new Integer(3)); v.addElement(new Integer(4)); System.out.println("Capacity after four additions: " + v.capacity()); // enumerate the elements in the vector. Enumeration vEnum = v.elements(); System.out.println("\nElements in vector:"); while(vEnum.hasMoreElements()) System.out.print(vEnum.nextElement() + " "); System.out.println(); } }
Output
Capacity after four additions: 10 Elements in vector: 1 2 3 4
Advertisements
To Continue Learning Please Login