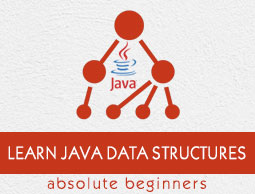
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Remove elements from a BitSet
You can clear the all the bits i.e. set all bits to false using the clear() method of the BitSet class. Similarly you can also clear the values at the required index by passing the index as a parameter to this method.
Example
Following is an example to remove the elements of a BitSet class. Here, we are trying to sets the elements at the indices with even values (up to 25) to true. Later we will clear the elements at the indices with values divisible by 5.
import java.util.BitSet; public class RemovingelementsOfBitSet { public static void main(String args[]) { BitSet bitSet = new BitSet(10); for (int i = 1; i<25; i++) { if(i%2==0) { bitSet.set(i); } } System.out.println(bitSet); System.out.println("After clearing the contents ::"); for (int i = 0; i<25; i++) { if(i%5==0) { bitSet.clear(i); } } System.out.println(bitSet); } }
Output
{2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 22, 24} After clearing the contents :: {2, 4, 6, 8, 12, 14, 16, 18, 22, 24}
Advertisements
To Continue Learning Please Login