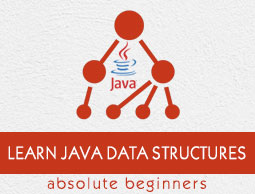
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Searching for maximum value in a tree
To find the maximum value of a tree (without child nodes) compare the left node and right node and get the larger value (store it in max) then, compare it with the value of the root
If the result (max) is greater then, it is the maximum value of the tree else the root is the maximum value of the tree.
To get the maximum value of a whole binary tree get the maximum value of the left subtree, the maximum value of the right subtree and, the root. Now compare three of them larger value among these three is the maximum value of the tree.
Example
class Node{ int data; Node leftNode, rightNode; Node() { leftNode = null; rightNode = null; this.data = data; } Node(int data) { leftNode = null; rightNode = null; this.data = data; } int getData() { return this.data; } Node getleftNode() { return this.leftNode; } Node getRightNode() { return this.leftNode; } void setData(int data) { this.data = data; } void setleftNode(Node leftNode) { this.leftNode = leftNode; } void setRightNode(Node rightNode) { this.leftNode = rightNode; } } public class MaxValueInBinaryTree { public static void main(String[] args){ Node node = new Node(50); node.leftNode = new Node(60); node.leftNode.leftNode = new Node(45); node.leftNode.rightNode = new Node(64); node.rightNode = new Node(60); node.rightNode.leftNode = new Node(45); node.rightNode.rightNode = new Node(64); System.out.println("Maximum value is "+maximumValue(node)); } public static int maximumValue(Node root) { int max = 0; if(root!=null) { int lMax = maximumValue(root.leftNode); int rMax = maximumValue(root.rightNode);; if(lMax>rMax){ max = lMax; } else { max = rMax; } if(root.data>max) { max = root.data; } } return max; } }
Output
Maximum value is 64
Advertisements
To Continue Learning Please Login