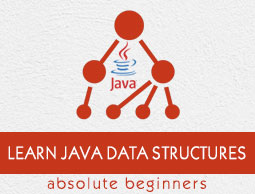
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Creating a Binary Tree
A tree is a data structure with elements/nodes connected to each other similar to linked list. But, unlike linked list a tree is a nonlinear data structure where each element/node in a tree is connected to multiple nodes (in hierarchical manner).
In a tree the node without any preceding elements i.e. node at the top of the tree, is known as the root node. Each tree contains only one root node.
Any node except the root node has one edge upward to a node called parent.
The node below a given node connected by its edge downward is called its child node.
The node which does not have any child node is called the leaf node.
A tree where each node have 0 or, 1 or 2 children maximum 2 is known as a binary tree. A binary tree has the benefits of both an ordered array and a linked list as search is as quick as in a sorted array and insertion or deletion operation are as fast as in linked list.
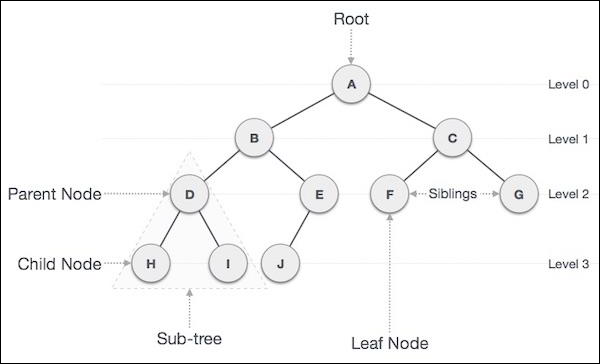
Creating the Binary Tree in Java
To create/implement a binary tree create a Node class that will store int values and keep a reference to each child create three variables.
Two variables of Node type to store left and right nodes and one is of integer type to store data. Then from another class try creating nodes such that no node should have more than 2 child nodes in a hierarchical manner.
Example
Following is an example of creating a binary tree here we have created a Node class with variables for data, left and, right nodes including setter and getter methods to set and retrieve values of them.
import java.util.LinkedList; import java.util.Queue; class Node{ int data; Node leftNode, rightNode; Node() { leftNode = null; rightNode = null; this.data = data; } Node(int data) { leftNode = null; rightNode = null; this.data = data; } int getData() { return this.data; } Node getleftNode() { return this.leftNode; } Node getRightNode() { return this.leftNode; } void setData(int data) { this.data = data; } void setleftNode(Node leftNode) { this.leftNode = leftNode; } void setRightNode(Node rightNode) { this.leftNode = rightNode; } } public class CreatingBinaryTree { public static void main(String[] args){ Node node = new Node(50); node.leftNode = new Node(60); node.leftNode.leftNode = new Node(45); node.leftNode.rightNode = new Node(64); node.rightNode = new Node(60); node.rightNode.leftNode = new Node(45); node.rightNode.rightNode = new Node(64); System.out.println("Binary Tree Created Pre-order of its elements is: "); preOrder(node); } public static void preOrder(Node root){ if(root !=null){ System.out.println(root.data); preOrder(root.leftNode); preOrder(root.rightNode); } } }
Output
Binary Tree Created Pre-order of its elements is: 50 60 45 64 60 45 64
To Continue Learning Please Login