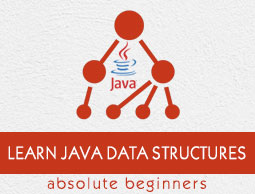
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Popping elements from a Stack
The pop operation in a Stack refers to the removal of the elements from the stack. On performing this operation on the stack the element at the top of the stack will be removed i.e. the element inserted at last into the stack will be popped at first. (Last in first out)
Example
import java.util.Stack; public class PoppingElements { public static void main(String args[]) { Stack stack = new Stack(); stack.push(455); stack.push(555); stack.push(655); stack.push(755); stack.push(855); stack.push(955); System.out.println("Elements of the stack are :"+stack.pop()); System.out.println("Contents of the stack after popping the element :"+stack); } }
Output
Elements of the stack are :955 Contents of the stack after popping the element :[455, 555, 655, 755, 855]
Advertisements
To Continue Learning Please Login