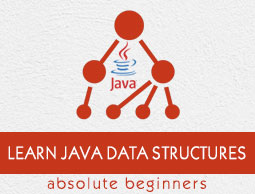
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Remove elements from a Dictionary
You can remove elements of the dictionary using the remove() method of the dictionary class. This method accepts key or key-value pair and deletes the respective element.
dic.remove("Ram"); or dic.remove("Ram", 94.6);
Example
import java.util.Hashtable; import java.util.Dictionary; public class RemovingElements { public static void main(String args[]) { Dictionary dic = new Hashtable(); dic.put("Ram", 94.6); dic.put("Rahim", 92); dic.put("Robert", 85); dic.put("Roja", 93); dic.put("Raja", 75); System.out.println("Contents of the hash table :"+dic); dic.remove("Ram"); System.out.println("Contents of the hash table after deleting specified elements :"+dic); } }
Output
Contents of the hash table :{Rahim = 92, Roja = 93, Raja = 75, Ram = 94.6, Robert = 85} Contents of the hash table after deleting specified elements :{Rahim = 92, Roja = 93, Raja = 75, Robert = 85}
Advertisements
To Continue Learning Please Login