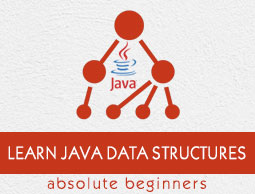
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Java Data Structures - Stack Class
A stack is an Abstract Data Type (ADT), commonly used in most programming languages. It is named stack as it behaves like a real-world stack, for example – a deck of cards or a pile of plates, etc.
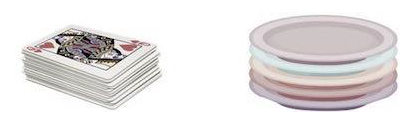
A real-world stack allows operations at one end only. For example, we can place or remove a card or plate from the top of the stack only. Likewise, Stack ADT allows all data operations at one end only. At any given time, we can only access the top element of a stack.
This feature makes it LIFO data structure. LIFO stands for Last-in-first-out. Here, the element which is placed (inserted or added) last, is accessed first. In stack terminology, insertion operation is called PUSH operation and removal operation is called POP operation.
Stack Representation
The following diagram depicts a stack and its operations −
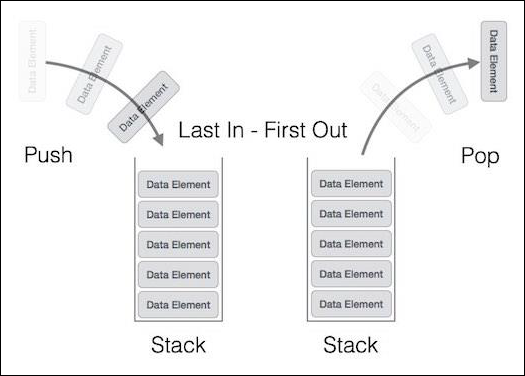
A stack can be implemented by means of Array, Structure, Pointer, and Linked List. Stack can either be a fixed size one or it may have a sense of dynamic resizing. Here, we are going to implement stack using arrays, which makes it a fixed size stack implementation.
The Stack Class
Stack is a subclass of Vector that implements a standard last-in, first-out stack.
Stack only defines the default constructor, which creates an empty stack. Stack includes all the methods defined by Vector, and adds several of its own.
Stack( )
Apart from the methods inherited from its parent class Vector, Stack defines the following methods −
Sr.No. | Method and Description |
---|---|
1 | boolean empty() Tests if this stack is empty. Returns true if the stack is empty, and returns false if the stack contains elements. |
2 | Object peek( ) Returns the element on the top of the stack, but does not remove it. |
3 | Object pop( ) Returns the element on the top of the stack, removing it in the process. |
4 | Object push(Object element) Pushes the element onto the stack. Element is also returned. |
5 | int search(Object element) Searches for element in the stack. If found, its offset from the top of the stack is returned. Otherwise, .1 is returned. |
Example
The following program illustrates several of the methods supported by this collection −
import java.util.*; public class StackDemo { static void showpush(Stack st, int a) { st.push(new Integer(a)); System.out.println("push(" + a + ")"); System.out.println("stack: " + st); } static void showpop(Stack st) { System.out.print("pop -> "); Integer a = (Integer) st.pop(); System.out.println(a); System.out.println("stack: " + st); } public static void main(String args[]) { Stack st = new Stack(); System.out.println("stack: " + st); showpush(st, 42); showpush(st, 66); showpush(st, 99); showpop(st); showpop(st); showpop(st); try { showpop(st); } catch (EmptyStackException e) { System.out.println("empty stack"); } } }
Output
stack: [ ] push(42) stack: [42] push(66) stack: [42, 66] push(99) stack: [42, 66, 99] pop -> 99 stack: [42, 66] pop -> 66 stack: [42] pop -> 42 stack: [ ] pop -> empty stack
To Continue Learning Please Login