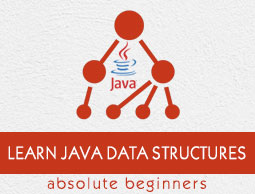
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Loop through a Dictionary
The Dictionary class provides a method named keys() which returns an enumeration object holding the all keys in the hash table.
Using this method get the keys and retrieve the value of each key using the get() method.
The hasMoreElements() method of the Enumeration (interface) returns true if the enumeration object has more elements. You can use this method to run the loop.
Example
import java.util.Enumeration; import java.util.Hashtable; import java.util.Dictionary; public class Loopthrough { public static void main(String args[]) { String str; Dictionary dic = new Hashtable(); dic.put("Ram", 94.6); dic.put("Rahim", 92); dic.put("Robert", 85); dic.put("Roja", 93); dic.put("Raja", 75); Enumeration keys = dic.keys(); System.out.println("Contents of the hash table are :"); while(keys.hasMoreElements()) { str = (String) keys.nextElement(); System.out.println(str + ": " + dic.get(str)); } } }
Output
Contents of the hash table are : Rahim: 92 Roja: 93 Raja: 75 Ram: 94.6 Robert: 85
Advertisements
To Continue Learning Please Login