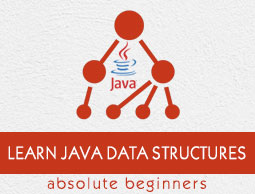
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Retrieving values in a hash table
You can retrieve the value of a particular key using the get() method. If you pass the key of a particular element as a parameter of this method, it returns the value of the specified key (as an object). If the hash table doesn’t contain any element under the specified key it returns null.
You can retrieve the values in a hash table using this method.
Example
import java.util.Hashtable; public class RetrievingElements { public static void main(String args[]) { Hashtable hashTable = new Hashtable(); hashTable.put("Ram", 94.6); hashTable.put("Rahim", 92); hashTable.put("Robert", 85); hashTable.put("Roja", 93); hashTable.put("Raja", 75); Object ob = hashTable.get("Ram"); System.out.println("Value of the specified key :"+ ob); } }
Output
Value of the specified key :94.6
Advertisements
To Continue Learning Please Login