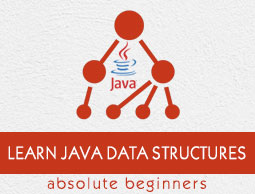
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Remove elements from a linked list
The remove() method of the LinkedList class accepts an element as a parameter and removes it from the current linked list.
You can use this method to remove elements from a linked list.
Example
import java.util.LinkedList; public class RemovingElements { public static void main(String[] args) { LinkedList linkedList = new LinkedList(); linkedList.add("Mangoes"); linkedList.add("Grapes"); linkedList.add("Bananas"); linkedList.add("Oranges"); linkedList.add("Pineapples"); System.out.println("Contents of the linked list :"+linkedList); linkedList.remove("Grapes"); System.out.println("Contents of the linked list after removing the specified element :"+linkedList); } }
Output
Contents of the linked list :[Mangoes, Grapes, Bananas, Oranges, Pineapples] Contents of the linked list after removing the specified element :[Mangoes, Bananas, Oranges, Pineapples]
Advertisements
To Continue Learning Please Login