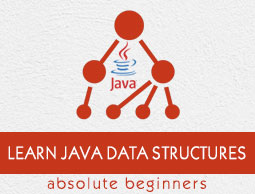
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Java Data Structures - Linear Search
Linear search is a very simple search algorithm. In this type of search, a sequential search is made over all items one by one. Every item is checked and if a match is found then that particular item is returned, otherwise the search continues till the end of the data collection.
Algorithm
Linear Search. ( Array A, Value x)
Step 1: Get the array and the element to search. Step 2: Compare value of the each element in the array to the required value. Step 3: In case of match print element found.
Example
public class LinearSearch { public static void main(String args[]) { int array[] = {10, 20, 25, 63, 96, 57}; int size = array.length; int value = 63; for (int i=0 ;i< size-1; i++) { if(array[i]==value) { System.out.println("Index of the required element is :"+ i); } } } }
Output
Index of the required element is :3
Advertisements
To Continue Learning Please Login