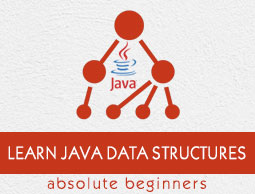
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Java Data Structures - Creating Arrays
In Java array is a data structure/container, which stores a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
Element − Each item stored in an array is called an element.
Index − Each location of an element in an array has a numerical index, which is used to identify the element.
Creating Arrays
To create an array, you need to declare the particular array, by specifying its type, and the variable to reference it. Then, allot memory to the declared array using the new operator (specify the size of the array in the square braces ‘[ ]’).
Syntax
dataType[] arrayRefVar; arrayRefVar = new dataType[arraySize]; (or) dataType[] arrayRefVar = new dataType[arraySize];
Alternatively, you can create an array by directly specifying the elements separated by commas, within the flower braces “{ }”.
dataType[] arrayRefVar = {value0, value1, ..., valuek};
The array elements are accessed through the index. Array indices are 0-based; that is, they start from 0 to arrayRefVar.length-1.
Example
Following statement declares an array variable of integer type, myArray, and allots memory to store of 10 elements of integer type and assigns its reference to myArray.
int[] myList = new int[10];
Populating the array
The above statement Just creates an empty array. You need populate this array by assigning values to each position using the index −
myList [0] = 1; myList [1] = 10; myList [2] = 20; . . . .
Example
Following is an Java example to create an integer. In this example we are trying to create an integer array of size 10, populate it, display the contents of it using loops.
public class CreatingArray { public static void main(String args[]) { int[] myArray = new int[10]; myArray[0] = 1; myArray[1] = 10; myArray[2] = 20; myArray[3] = 30; myArray[4] = 40; myArray[5] = 50; myArray[6] = 60; myArray[7] = 70; myArray[8] = 80; myArray[9] = 90; System.out.println("Contents of the array ::"); for(int i = 0; i<myArray.length; i++) { System.out.println("Element at the index "+i+" ::"+myArray[i]); } } }
Output
Contents of the array :: Element at the index 0 ::1 Element at the index 1 ::10 Element at the index 2 ::20 Element at the index 3 ::30 Element at the index 4 ::40 Element at the index 5 ::50 Element at the index 6 ::60 Element at the index 7 ::70 Element at the index 8 ::80 Element at the index 9 ::90
Example
Following is another Java example which creates and populates an array by taking inputs from user.
import java.util.Scanner; public class CreatingArray { public static void main(String args[]) { // Instantiating the Scanner class Scanner sc = new Scanner(System.in); // Taking the size from user System.out.println("Enter the size of the array ::"); int size = sc.nextInt(); // creating an array of given size int[] myArray = new int[size]; // Populating the array for(int i = 0 ;i<size; i++) { System.out.println("Enter the element at index "+i+" :"); myArray[i] = sc.nextInt(); } // Displaying the contents of the array System.out.println("Contents of the array ::"); for(int i = 0; i<myArray.length; i++) { System.out.println("Element at the index "+i+" ::"+myArray[i]); } } }
Output
Enter the size of the array :: 5 Enter the element at index 0 : 25 Enter the element at index 1 : 65 Enter the element at index 2 : 78 Enter the element at index 3 : 66 Enter the element at index 4 : 54 Contents of the array :: Element at the index 0 ::25 Element at the index 1 ::65 Element at the index 2 ::78 Element at the index 3 ::66 Element at the index 4 ::54
To Continue Learning Please Login