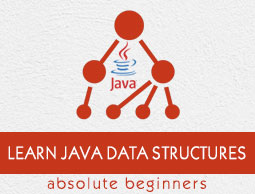
- Java Data Structures Tutorial
- Java Data Structures Resources
- Java Data Structures - Quick Guide
- Java Data Structures - Resources
- Java Data Structures - Discussion
Verifying if the BitSet is empty
A bit set is considered as empty when all the values in it are false. The BitSet class provides the isEmpty() method. This method returns a boolean value, which is false when the current BitSet is empty and true when it is not empty.
You can verify whether a particular BitSet is empty using the isEmpty() method.
Example
import java.util.BitSet; public class isEmpty { public static void main(String args[]) { BitSet bitSet = new BitSet(10); for (int i = 1; i<25; i++) { if(i%2==0) { bitSet.set(i); } } if (bitSet.isEmpty()) { System.out.println("This BitSet is empty"); } else { System.out.println("This BitSet is not empty"); System.out.println("The contents of it are : "+bitSet); } bitSet.clear(); if (bitSet.isEmpty()) { System.out.println("This BitSet is empty"); } else { System.out.println("This BitSet is not empty"); System.out.println("The contents of it are : "+bitSet); } } }
Output
This BitSet is not empty The contents of it are : {2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 22, 24} This BitSet is empty
Advertisements
To Continue Learning Please Login