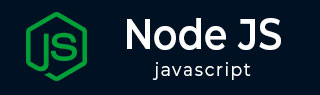
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - os.cpus() Method
The Node.js os.cpus() method will return an array of objects which contains detailed information about every logical CPU core of the current computer.
In each entry, there are a few properties. There's a property named model, which is a string that indicates the model of the system's CPU. Another property named speed is a numeric property that is measured in megahertz. The times property from the output is an object with the following properties −
model − This attribute is a string that specifies the model of the current CPU core.
speed − This attribute is a number that specifies the speed of the CPU core in MHz (megahertz).
times − An object that contains the following properties.
user − This attribute gives a number that specifies the time that the CPU spent in user mode in milliseconds.
nice − This attribute gives a number that specifies the time that the CPU spent in the nice mode in milliseconds.
sys − This attribute gives a number that specifies the time that the CPU spent in sys mode in milliseconds.
idle − This attribute gives a number that specifies the time that the CPU spent in idle mode in milliseconds.
irq − This attribute gives a number that specifies the time that the CPU spent in irq mode in milliseconds.
Syntax
Following is the syntax of the Node.js os.cpus() method −
os.cpus()
Parameters
This method does not accept any parameters.
Return value
This method will return an array of objects containing data about the logical cores of the current computer.
Note − We can get the 'nice' values only in POSIX. Whereas in the windows operating system, the 'nice' value of every processor will always be 0.
Example
In the following example, we are trying to log the Node.js os.cpus() method to the console.
const os = require('os'); const {cpus} = os; console.log(os.cpus());
Output
[ { model: 'AMD EPYC 7502P 32-Core Processor', speed: 1500, times: { user: 189226200, nice: 0, sys: 80776200, idle: 8565692600, irq: 0 } }, { model: 'AMD EPYC 7502P 32-Core Processor', speed: 1500, times: { user: 224461400, nice: 9000, sys: 97874200, idle: 8571598100, irq: 0 } }, . . . . . . . . { model: 'AMD EPYC 7502P 32-Core Processor', speed: 1500, times: { user: 114360400, nice: 0, sys: 80239500, idle: 8711811800, irq: 0 } }, { model: 'AMD EPYC 7502P 32-Core Processor', speed: 1500, times: { user: 112385500, nice: 0, sys: 51727200, idle: 8742557600, irq: 0 } } ]
Note − To get the accurate result, better execute the above code in local.
If we compile and run the above program, the os.cpus() will return an array of objects which will contain the information about each logical CPU core.
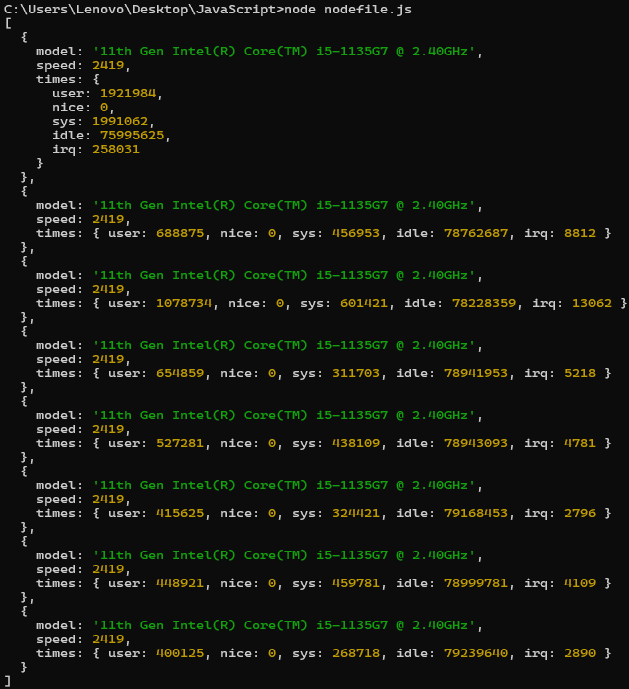
Example
In the example below, we are trying to label each logical CPU core with numbers by using foreach() method. Then we are printing a statement with the total number of logical CPU cores in the current computer.
const os = require('os'); const {cpus} = os; let CPU = os.cpus(); let LogicalCores=0; CPU.forEach(element => { LogicalCores++; console.log("Logical CPU core " + LogicalCores + " - "); console.log(element); }); console.log("Total number of Logical CPU cores are: " + LogicalCores);
Output
Logical CPU core 1 - { model: 'AMD EPYC 7502P 32-Core Processor', speed: 1500, times: { user: 2242000, nice: 0, sys: 1316600, idle: 281004400, irq: 0 } } Logical CPU core 2 - { model: 'AMD EPYC 7502P 32-Core Processor', speed: 1500, times:{ user: 5663000, nice: 0, sys: 1501800, idle: 278434700, irq: 0 } } . . . . . . . Logical CPU core 63 - { model: 'AMD EPYC 7502P 32-Core Processor', speed: 1500, times: { user: 2922500, nice: 0, sys: 1063400, idle: 281854900, irq: 0 } } Logical CPU core 64 - { model: 'AMD EPYC 7502P 32-Core Processor', speed: 1500, times: { user: 596600, nice: 0, sys: 813400, idle: 284439400, irq: 0 } } Total number of Logical CPU cores are: 64
Note − To get the accurate result, better execute the above code in local.
If we compile and run the above program, we get all the logical CPU cores of our current computer. We can see that in the figure below.
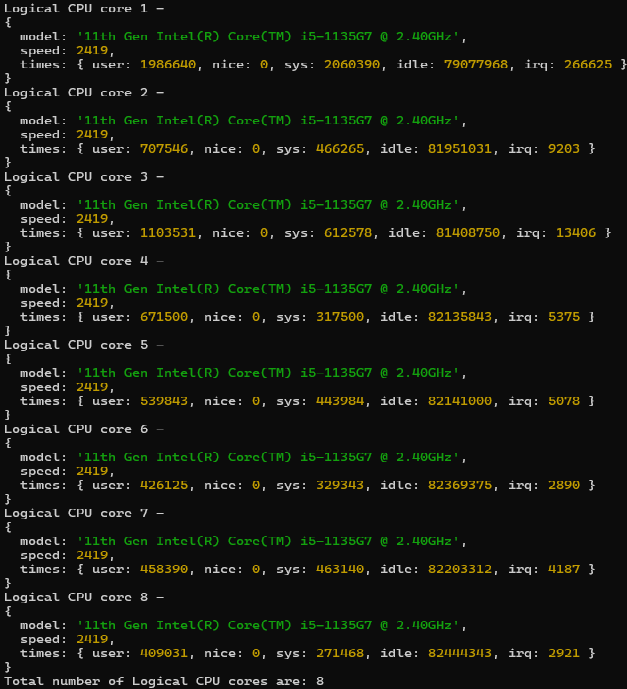