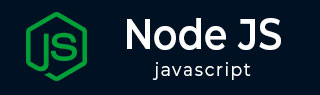
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - MySQL Where
In MySQL, the WHERE clause can be used in SELECT, DELETE and UPDATE queries. The WHERE clause allows you to specify a search condition for the rows returned by a query. When using mysql module with a Node.js application, the query() method of connection object executes a query string. To apply filter on a SELECT or UPDATE or DELETE queries, the WHERE clause is used. In this chapter, various examples of usage of WHERE clause in a Node.js application are explained.
The following shows the syntax of the WHERE clause in a SELECT statement −
SELECT select_list FROM table_name WHERE search_condition;
The search_condition is a combination of one or more expressions using the logical operator AND, OR and NOT.
Various operators used in WHERE clause are −
Sr.No | Operator & Description |
---|---|
1 | = Equal |
2 | > Greater than |
3 | < Less than |
4 | >= Greater than or equal |
5 | <= Less than or equal |
6 | != Not equal |
7 | BETWEEN Between a certain range |
8 | LIKE Search for a pattern |
9 | IN To specify multiple possible values for a column |
In MySQL, a predicate is a Boolean expression that evaluates to TRUE, FALSE, or UNKNOWN. The SELECT statement will include any row that satisfies the search_condition in the result set.
Logical operators
In the following Node.js application code, the SELECT query string passed to the query() method uses WHERE clause to fetch the employee records whose salary exceeds Rs. 25000.
Example
var mysql = require('mysql'); var con = mysql.createConnection({ host: "localhost", user: "root", password: "mypassword", database: "mydb" }); var qry =`SELECT name,salary FROM employee WHERE salary>25000;`; con.connect(function (err) { if (err) throw err; console.log("Connected!"); con.query(qry, function (err, results) { if (err) throw err; console.log(results); }); con.end(); });
Output
[ RowDataPacket { name: 'Anil', salary: 30000 }, RowDataPacket { name: 'Meena', salary: 27000 } ]
The filter condition in the WHERE clause may be a compound logical expression, where the individual logical expressions that use the comparison operators (>, <, >=.<=, ==, =) are combined with either AND, OR or NOT operators.
Let us change the SELECT query in the query() method to apply the condition on salary field such that it is more than 25000 and less than 30000.
Example
var mysql = require('mysql'); var con = mysql.createConnection({ host: "localhost", user: "root", password: "mypassword", database: "mydb" }); var qry =`SELECT name,salary FROM employee WHERE salary>25000 and salary>30000;`; con.connect(function (err) { if (err) throw err; console.log("Connected!"); con.query(qry, function (err, results) { if (err) throw err; console.log(results); }); con.end(); });
Output
[ RowDataPacket { name: 'Meena', salary: 27000 } ]
BETWEEN
The BETWEEN checks if a certain field value falls between the given range. The following query string fetched employees whose salary lies between 27000 and 30000. In the above code, change the qry variable to the following expression, keep rest of the code same.
Example
var qry =`SELECT name,salary FROM employee WHERE salary BETWEEN 27000 and 30000;`;
Output
[ RowDataPacket { name: 'Anil', salary: 30000 }, RowDataPacket { name: 'Meena', salary: 27000 } ]
LIKE
The LIKE operator evaluates to TRUE if a value matches a specified pattern. To form a pattern, you use the % and _ wildcards. The % wildcard matches any string of zero or more characters while the _ wildcard matches any single character.
Change the query string variable to the following.
var qry =`SELECT name,salary FROM employee WHERE name LIKE '%n%';`;
The resultset will include names containing the alphabet 'n' anywhere in the name.
[ RowDataPacket { name: 'Anil', salary: 30000 }, RowDataPacket { name: 'Meena', salary: 27000 } ]
IN
MySQL also support IN operator along with WHERE clause. The IN operator returns TRUE if a value matches any value in a list.
value IN (value1, value2,...)
Change the query string in the code to following.
var qry =`SELECT name,salary FROM employee WHERE name IN ('Anil', 'Tina', 'Ravi');`;
This will return the resultset wherein the rows with names are found in the given list.
[ RowDataPacket { name: 'Ravi', salary: 25000 }, RowDataPacket { name: 'Anil', salary: 30000 } ]