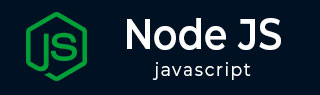
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Send an Email
Sending Email is one the desired features in any Node.js application, especially an Express app. There are various third party modules that facilitate this on the NPM repository. A Node.js app can be integrated with email facility with email APIs such as Mailgun, MailTrap, Mailjet etc.
In this chapter, we shall learn how to send email with a Node.js application with the help of nodemailer module and MilTrap API.
Nodemailer
Nodemailer is a module that enables email sending for Node.js applications. You can send emails with plain text as well as HTML content. It also supports adding attachments, secured TLS delivery, built-in SMTP support, OAUTH2 authentication among many other features.
To get started, install the nodemailer module.
npm install nodemailer
To test our email-enabled Node.js application, we shall use Mailtrap service, that provides a dummy SMTP server. You can use the real SMTP server URL when the application goes to the production stage. Create a Mailtrap account and obtain the API credentials from the Integrations dropdown on the dashboard, select Nodemailer.
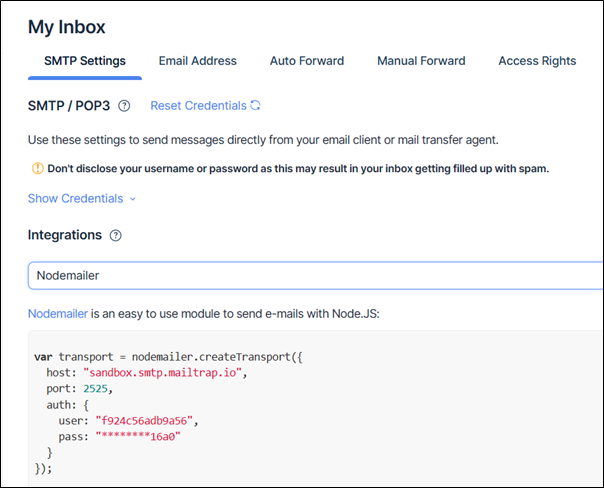
Save the above code along with the rest of the code given below −
const nodemailer = require('nodemailer'); var transport = nodemailer.createTransport({ host: "sandbox.smtp.mailtrap.io", port: 2525, auth: { user: "f924c******a56", pass: "******1b5516a0" } }); message = { from: "from-example@email.com", to: "to-example@email.com", subject: "Subject", text: "Hello SMTP Email" } transport.sendMail(message, function(err, info) { if (err) { console.log(err); } else { console.log(info); } });
Nodemailer's createTransport function specifies which method you want to use for sending email. It takes the connection data and credentials as an argument. In this case, since SMTP is the preferred transport, you will need to define an SMTP host, port, and credential password for accessing a host SMTP server.
The createTrsnsport() function returns a transport object. It's sendMail() method uses the credentials and message details and sends the message to the desired recipient. Run the above code as follows −
PS D:\nodejs\emailapp> node main.js { accepted: [ 'to-example@email.com' ], rejected: [], ehlo: [ 'SIZE 5242880', 'PIPELINING', 'ENHANCEDSTATUSCODES', '8BITMIME', 'DSN', 'AUTH PLAIN LOGIN CRAM-MD5' ], envelopeTime: 749, messageTime: 529, messageSize: 291, response: '250 2.0.0 Ok: queued', envelope: { from: 'from-example@email.com', to: [ 'to-example@email.com' ] }, messageId: '<1d29decd-7903-f5e4-3578-15b1e88f239a@email.com>' }
The dummy SMTP server provided by MailTrap provides you an inbox, wherein the email transaction can be verified.
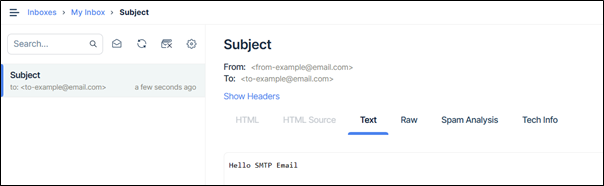
You can also use the popular Gmail service to send email through your Node.js code. Note that you may have to either enable less secure apps setting of the gmail account or use the authentication token if the account is using OAuth2 authentication.
var transport = nodemailer.createTransport({ service: 'gmail', auth: { user: 'youremail@gmail.com', pass: 'yourpassword' } });
Mailgun
You can also incorporate email facility in your Node.js application by using Mailgun API keys. To add this facility, install following modules −
npm i mailgun.js form-data
Sign up for a free Mailgun account by visiting the URL signup.mailgun.com and obtain public and private API keys.
Then, we initialize the Mailgun client instance and passing in the MAILGUN_API_KEY. Then we defined a function sendMail to handle the sending of emails using the mailgun-js library.
const formData = require("form-data"); const Mailgun = require("mailgun.js"); const mailgun = new Mailgun(formData); const mg = mailgun.client({ username: "api", key: process.env.MAILGUN_API_KEY, }); exports.sendMail = (req, res) => { const { toEmail, fromEmail, subject, message } = req.body; mg.messages.create(process.env.MAILGUN_DOMAIN, { from: fromEmail, to: [toEmail], subject: subject, text: message, }); };