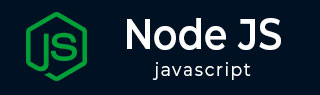
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - MongoDB Sort
The result of find() query on a collection in a MongoDB database can be arranged in ascending or descending order of a certain field in the Document. The mongodb driver for Node.js has sort() method defined in the Collection object.
To sort the results of a MongoDB query in Node.js, you can use the sort() method. This method allows you to order the returned documents by the values of one or more fields in a certain direction. To sort returned documents by a field in ascending (lowest first) order, use a value of 1. To sort in descending (greatest first) order instead, use -1.
The syntax for ascending order is as follows −
result = col.find(query).sort(field:1);
The syntax for descending sort is as follows −
result = col.find(query).sort(field:-1);
Ascending sort
The following example displays the documents in the products collection in the ascending order of price.
Example
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://localhost:27017/"; const client = new MongoClient(uri); try { await client.connect(); await sortdocs(client, "mydb", "products"); } finally { await client.close(); } } main().catch(console.error); async function sortdocs(client, dbname, colname){ var mysort = { price: 1 }; const result = await client.db(dbname).collection(colname).find({}).sort(mysort).toArray(); result.forEach(element => { console.log(element); }); }
Output
{ _id: new ObjectId('6580964f20f979d2e9a72ae9'), ProductID: 3, Name: 'Router', price: 2000 } { _id: new ObjectId('6580964f20f979d2e9a72aea'), ProductID: 4, Name: 'Scanner', price: 5000 } { _id: new ObjectId('6580964f20f979d2e9a72aeb'), ProductID: 5, Name: 'Printer', price: 9000 } { _id: new ObjectId('65809214693bd4622484dce3'), ProductID: 1, Name: 'Laptop', Price: 25000 } { _id: new ObjectId('6580964f20f979d2e9a72ae8'), ProductID: 2, Name: 'TV', price: 40000 }
Descending sort
To produce list of documents from products collection in the descending order of name field, change the sortdocs() function to the following −
Example
async function sortdocs(client, dbname, colname){ var mysort = { Name: -1 }; const result = await client.db(dbname).collection(colname).find({}).sort(mysort).toArray(); result.forEach(element => { console.log(element); }); });
Output
{ _id: new ObjectId('6580964f20f979d2e9a72ae8'), ProductID: 2, Name: 'TV', price: 40000 } { _id: new ObjectId('6580964f20f979d2e9a72aea'), ProductID: 4, Name: 'Scanner', price: 5000 } { _id: new ObjectId('6580964f20f979d2e9a72ae9'), ProductID: 3, Name: 'Router', price: 2000 } { _id: new ObjectId('6580964f20f979d2e9a72aeb'), ProductID: 5, Name: 'Printer', price: 9000 } { _id: new ObjectId('65809214693bd4622484dce3'), ProductID: 1, Name: 'Laptop', Price: 25000 }