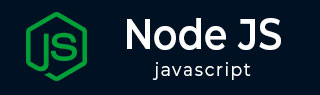
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - MongoDB Limit
The Limit() method in MongoDB has a similar effect as in Limit clause in SQL. It restricts the number of documents returned by find() query to a specified number. The limit() method takes a single integer as argument. It the argument is omitted, it implies that no limit has been applied.
To understand how the limit() function works, we shall use the orders collection in our database. Out of the available documents, those with price greater than or equal to 10 can be retrieved by the following code −
Example
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://localhost:27017/"; const client = new MongoClient(uri); try { await client.connect(); await limitdocs(client, "mydb", "orders"); } finally { await client.close(); } } main().catch(console.error); async function limitdocs(client, dbname, colname){ var myqry = {numPurchased:{$gte:10}}; const result = await client.db(dbname).collection(colname).find({"numPurchased":{$gte:10}}).toArray(); console.log(JSON.stringify(result)); }
Output
[{"_id":"658d7f3b30a92c8e5018a43a","orderId":201,"custid":301,"prodId":100,"numPurchased":20},{"_id":"658d7f3b30a92c8e5018a43b","orderId":202,"custid":302,"prodId":101,"numPurchased":10},{"_id":"658d7f3b30a92c8e5018a43d","orderId":204,"custid":303,"prodId":103,"numPurchased":15},{"_id":"658d7f3b30a92c8e5018a43e","orderId":205,"custid":303,"prodId":103,"numPurchased":20},{"_id":"658d7f3b30a92c8e5018a441","orderId":208,"custid":301,"prodId":100,"numPurchased":10},{"_id":"658d7f3b30a92c8e5018a442","orderId":209,"custid":303,"prodId":103,"numPurchased":30}]
Now we will apply a limit to the result returned by find() query to 1. The syntax of limit() method is as follows −
collection.find(query).limit(number);
Change the limitdocs() function to the following code, to obtain number of documents limited to the specified number.
async function limitdocs(client, dbname, colname){ var myqry = {numPurchased:{$gte:10}}; const result = await client.db(dbname).collection(colname).find({"numPurchased":{$gte:10}}).limit(1).toArray(); console.log(JSON.stringify(result)); }
Output
[{"_id":"658d7f3b30a92c8e5018a43a","orderId":201,"custid":301,"prodId":100,"numPurchased":20}]
The find() query fetches the documents tht atisfy the filter condition, starting from its first occurrence. If you want to skip certain number of documents, use the skip() clause.
collection.find(query).limit(x).skip(y)
Out of the documents returned by find(), only x documents will populate the resultset, out of which first y documents will be removed.
The following limitdocs() function return the second document in orders collection that has price>=10
async function limitdocs(client, dbname, colname){ var myqry = {numPurchased:{$gte:10}}; const result = await client.db(dbname).collection(colname).find({"numPurchased":{$gte:10}}).limit(1).skip(1).toArray(); console.log(JSON.stringify(result)); }
Output
[{"_id":"658d7f3b30a92c8e5018a43b","orderId":202,"custid":302,"prodId":101,"numPurchased":10}]