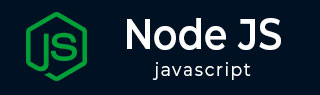
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Debugger
For smaller Node.js code, you can use console.log() or console.debug() functions to intermittently display messages or values of variables to manually debug the code. However, if the Node.js code becomes bigger and more complex, the manual method is not helpful. Instead, we can use the inbuilt debugger in Node.js runtime.
Consider a simple Node.js code as given below (main.js) −
let marks = [34, 45, 98, 26, 51]; let total = 0; for (let i = 0; i <= marks.length; i++) { total += marks[i]; } var no = marks.length; console.log(total/no);
We expect that the above program calculates the average marks obtained by 5 students. However, the result is not what we expect, when we run it as follows −
PS D:\nodejs> node main.js NaN
In JavaScript, NaN means Not a Number. To trace this error, let’s use the Node.js debugger. Instead, run the program with the following command −
PS D:\nodejs> node inspect main.js < Debugger listening on ws://127.0.0.1:9229/ca4aece6-308a-4979-8a32-734fe8ebbd0b < For help, see: https://nodejs.org/en/docs/inspector < connecting to 127.0.0.1:9229 ... ok < Debugger attached. < Break on start in a.js:1 > 1 let marks = [34, 45, 98, 26, 51]; 2 3 let total = 0; debug>
The built-in debugger starts at port 9229 of the localhost. The debugger breaks at the first line in the code.
We step through code by telling the debugger to go to the next line that the program will execute. Node.js allows the following commands to use a debugger −
Sr.No | Commands & Description |
---|---|
1 | c or cont Continue execution to the next breakpoint or to the end of the program. |
2 | n or next Move to the next line of code. |
3 | s or step Step into a function. By default, we only step through code in the block or scope we’re debugging. |
4 | o Step out of a function. After stepping into a function, the debugger goes back to the main file when the function returns. |
5 | pause Pause the running code. |
Press ‘n’ or ‘next’ successively to traverse the code. In this loop, two variables are changed every iteration—total and i. Let’s set up watchers for both of those variables.
debug> watch('total') debug> watch('i')
When you press ‘n’ in front of debug prompt, you get to see the instantaneous values of the two variables under watch.
break in main.js:6 Watchers: 0: total = 0 1: i = 0 4 5 > 6 for (let i = 0; i <= marks.length; i++) { 7 total += marks[i]; 8 }
Every time you press next, the changing values or total and I are displayed in debugger.
At the last iteration, the status of debugger is −
Watchers: 0: total = 254 1: i = 5 4 5 > 6 for (let i = 0; i <= marks.length; i++) { 7 total += marks[i]; 8 }
Next time, the value of total becomes NaN as the loop is trying to go beyond the array.
Watchers: 0: total = NaN 1: i = 6 4 5 > 6 for (let i = 0; i <= marks.length; i++) { 7 total += marks[i]; 8 }
Hence, we can come to know that the error is in the formation of for loop. The built-in debugger helps in identifying the error.
You can also enable debugging Node.js code inside VS Code. Open the command palette by pressing ctrl+shift+p and choose Attach Node.JS process. Then start debugging the program from the Run menu. It allows you to Step Over, Step Into and Step Out operations in the code to trace the flow of the program. You can also set watches on one or more variables to keep track of the values while the program is being executed step by step.