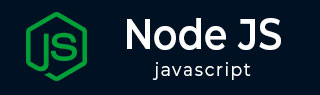
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - MongoDB Update
The Update operation refers to modifying the value of one or more fields in a document in a MongoDB collection. The mongodb driver for Node.js defines updateOne() and updateMany() methods. The updateOne() method is used to modify a single document, whereas the updateMany() method performs updating on more than one documents.
The updateOne() method has the following syntax −
collection.updateOne(query, values);
The query parameter helps in finding the desired document to be updated. The values parameter contains the key:value pairs with which the document is to be modified. The updateMany() method follows the same syntax, except that the query returns multiple documents from the collection.
updateOne()
The following example changes price of a document with ProductID=3. The $set operator assigns a new value to the price field.
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://localhost:27017/"; const client = new MongoClient(uri); try { await client.connect(); await sortdocs(client, "mydb", "products"); } finally { // Close the connection to the MongoDB cluster await client.close(); } } main().catch(console.error); async function sortdocs(client, dbname, colname){ var qry = { ProductID: 3 }; var vals = { $set: { Name: "Router", price: 2750 } }; const result = await client.db(dbname).collection(colname).updateOne(qry, vals); console.log("Document updated"); }
To verify that the document has been updated, use the Mongosh shell and enter the commands as below −
> use mydb < switched to db mydb > db.products.find({"ProductID":3}) { _id: ObjectId("6580964f20f979d2e9a72ae9"), ProductID: 3, Name: 'Router', price: 2750 }
updateMany()
Assuming that the products collection has the following documents that have the name ending with 'er'.
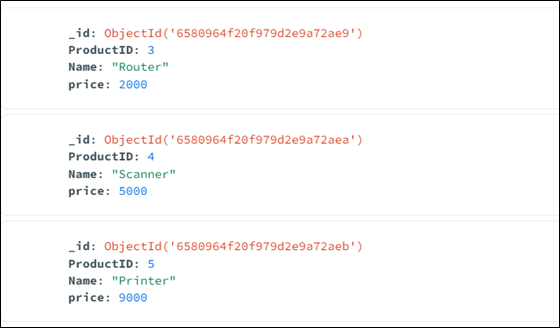
The following sortdocs() function increments the price of all the above products by Rs. 125. We have used the $inc operator.
Example
async function sortdocs(client, dbname, colname){ var qry = {Name: /er$/}; var vals = { $inc: { price: 125 } }; const result = await client.db(dbname).collection(colname).updateMany(qry, vals); console.log("Documents updated"); }
Output
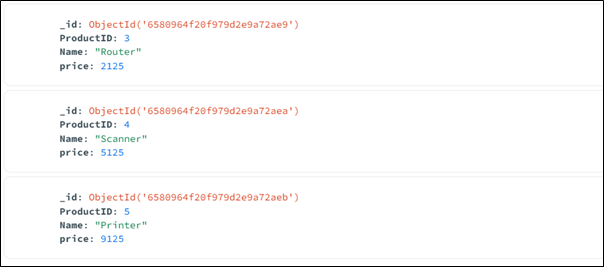