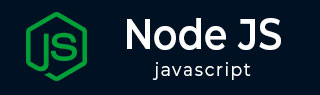
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - MongoDB Delete
The mongodb driver for Node.js includes two methods in the Collection class. The deleteOne() method deletes one document, whereas the deleteMany() method is used to delete more than on documents at a time. Both the methods need a filter argument.
collection.deleteOne(filter);
Note that if there are more than one documents that satisfy the given filter condition, only the first document is deleted.
deleteOne()
In the following example. The deleteOne() method removes a document from products collection, whose name field matches TV.
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://localhost:27017/"; const client = new MongoClient(uri); try { await client.connect(); await deldocs(client, "mydb", "products"); } finally { await client.close(); } } main().catch(console.error); async function deldocs(client, dbname, colname){ var myqry = { Name: "TV" }; const result = await client.db(dbname).collection(colname).deleteOne(myqry); console.log("Document Deleted"); }
Use MongoCompass (or MongoShell) to verify if the expected document has been removed after the above code is executed.
deleteMany()
The deleteMany() method also use the filter argument. However, it causes all the document satisfying the specified condition will be removed.
In the code above, change the deldocs() function as follows. It results in all the documents with price>10000 to be removed.
async function deldocs(client, dbname, colname){ var myqry = {"price":{$gt:10000}}; const result = await client.db(dbname).collection(colname).deleteMany(myqry); console.log("Documents Deleted"); }
Drop Collection
You can remove a collection from the database with drop() method.
Example
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://localhost:27017/"; const client = new MongoClient(uri); try { await client.connect(); await dropcol(client, "mydb", "products"); } finally { await client.close(); } } main().catch(console.error); async function dropcol(client, dbname, colname){ const result = await client.db(dbname).collection(colname).drop(); console.log("Collection dropped "); }
There is also a dropCollection() method available with the db object.
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://localhost:27017/"; const client = new MongoClient(uri); try { await client.connect(); await dropcol(client, "mydb", "orders"); } finally { await client.close(); } } main().catch(console.error); async function dropcol(client, dbname, colname){ const result = await client.db(dbname).dropCollection(colname); console.log("Collection dropped"); }