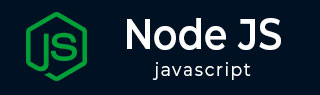
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - MongoDB Find
The MongoShell client is similar to the MySQL command-line tool. It is a tool for interaction with the MongoDB database. You can use MongoDB Language to perform CRUD operations. MongoDB Language is similar to SQL. The find() and findOne() methods available to the Collection object are the equivalent of SELECT query as in SQL. These methods are also defined in the mongodb module for use with Node.js application.
The find() method has one argument in the form of a query in JSON format.
db.collection.find({k:v});
The find() method returns a resultset consisting of all the documents from the collection that satisfy the given query. If the query argument is empty, it returns all the documents in the collection.
Read all documents
The following example retrieves all the documents in the products collection.
Example
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://localhost:27017/"; const client = new MongoClient(uri); try { // Connect to the MongoDB cluster await client.connect(); // Make the appropriate DB calls // Create a single new listing await listall(client, "mydb", "products"); } finally { // Close the connection to the MongoDB cluster await client.close(); } } main().catch(console.error); async function listall(client, dbname, colname){ const result = await client.db("mydb").collection("products").find({}).toArray(); console.log(JSON.stringify(result)); }
Output
[{"_id":"65809214693bd4622484dce3","ProductID":1,"Name":"Laptop","Price":25000},{"_id":"6580964f20f979d2e9a72ae7","ProductID":1,"Name":"Laptop","price":25000},{"_id":"6580964f20f979d2e9a72ae8","ProductID":2,"Name":"TV","price":40000},{"_id":"6580964f20f979d2e9a72ae9","ProductID":3,"Name":"Router","price":2000},{"_id":"6580964f20f979d2e9a72aea","ProductID":4,"Name":"Scanner","price":5000},{"_id":"6580964f20f979d2e9a72aeb","ProductID":5,"Name":"Printer","price":9000}]
You can also traverse the resultset with a forEach loop as follows −
var count=0; result.forEach(row => { count++; console.log(count, row['Name'], row['price']); });
Output
1 Desktop 20000 2 Laptop 25000 3 TV 40000 4 Router 2000 5 Scanner 5000 6 Printer 9000
findOne()
The findOne() method returns the first occurrence of the given query. The following code returns the document with name of the product as TV
async function listall(client, dbname, colname){ const result = await client.db(dbname).collection(colname).find({"Name":"TV"}).toArray(); console.log(JSON.stringify(result)); }
Output
[{"_id":"6580964f20f979d2e9a72ae8","ProductID":2,"Name":"TV","price":40000}]
If the query is empty, it returns the first document in the collection.
async function listall(client, dbname, colname){ const result = await client.db(dbname).collection(colname).findOne({}); console.log(JSON.stringify(result)); }
Output
{"_id":"65809214693bd4622484dce3","ProductID":1,"Name":"Laptop","Price":25000}