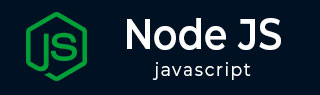
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - MongoDB Create Database
MongoDB is an open-source, cross-platform document-oriented database environment. MongoDB uses JSON-like, schema-free documents. More than one documents form a collection, and one or more collections may be present in a MongoDB database. In this chapter, we whall learn how to create a MongoDB database with a Node.js application.
The MongoDB database management software is available in the following three editions −
MongoDB Community − The source-available, free-to-use, and self-managed version of MongoDB, available for local installation, available for Windows, Linux, and macOS.
MongoDB Enterprise − A commercial, subscription-based, self-managed version of MongoDB, with a lot of advanced features than the community edition.
MongoDB Atlas − An on-demand, fully managed service for MongoDB deployments in the cloud. It runs on AWS, Microsoft Azure, and Google Cloud Platform.
Connection strings
The mongodb driver for Node.js is imported into the code with the require() function. The object of MongoClient class represents a database connection. You need to pass a connection string to its constructor.
const { MongoClient } = require('mongodb'); const client = new MongoClient(ConnectionString);
The MongoDB connection string must be one of the following formats −
Standard Connection String Format − This format is used to connect to a self-hosted MongoDB standalone deployment, replica set, or sharded cluster. The standard URI connection scheme has the form −
mongodb://[username:password@]host1[:port1][,...hostN[:portN]][/[defaultauthdb][?options]]
The default connection string is −
mongodb://localhost:27017/
SRV Connection Format − A connection string with a hostname that corresponds to a DNS SRV record. MongoDB Atlas uses SRV connection format. MongoDB supports a DNS-constructed seed list. It allows more flexibility of deployment and the ability to change the servers in rotation without reconfiguring clients. The SRV URI connection scheme has the following form −
mongodb+srv://[username:password@]host[/[defaultauthdb][?options]]
Here is an example of SRV connection string −
const uri = "mongodb+srv://user:pwd@cluster0.zhmrg1h.mongodb.net/?retryWrites=true&w=majority";
In the following example, we are using the standard connection string to fetch the list of databases.
Example: List of databases
The connect() method of MongoClient object returns a Promise. The call to connect() method is asynchronous. Next a function listdatabases() is called.
const { MongoClient } = require('mongodb'); async function main() { const uri = "mongodb://localhost:27017/"; const client = new MongoClient(uri); try { // Connect to the MongoDB cluster await client.connect(); // Make the appropriate DB calls await listDatabases(client); } catch (e) { console.error(e); } finally { // Close the connection to the MongoDB cluster await client.close(); } } main().catch(console.error); async function listDatabases(client) { databasesList = await client.db().admin().listDatabases(); console.log("Databases:"); databasesList.databases.forEach(db => console.log(` - ${db.name}`)); };
Output
Databases: - admin - config - local - myProject - mydb
Create a new database
To create a new database on the server, you need to call the db() method of MongoClient class.
var dbobj = client.db(database_name, options);
The db() method returns a db instance that represents the database on the server. In the following example, we have demonstrated how to create a new database named as mydatabase in MongoDB.
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://localhost:27017/mydb"; const client = new MongoClient(uri); try { // Connect to the MongoDB cluster await client.connect(); await createdb(client, "mydatabase"); } finally { // Close the connection to the MongoDB cluster await client.close(); } } main().catch(console.error); async function createdb(client, dbname){ const dbobj = await client.db(dbname); console.log("Database created"); console.log(dbobj); }
However, note that the database is not physically created on the server till at least one collection is created and there should be at least one document in it.