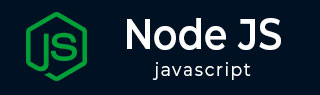
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - MongoDB Get Started
In this chapter, we shall learn to use MongoDB as a backend with Node.js application. A Node.js application can be interfaced with MongoDB through the NPM module called mongodb itself. MongoDB is a document-oriented NOSQL database. It is an open-source, distributed and horizontally scalable schema-free database architecture. Since MongoDB internally uses JSON-like format (called BSON) for data storage and transfer, it is a natural companion of Node.js, itself a JavaScript runtime, used for server-side processing.
Installation
MongoDB server software is available in two forms: Community edition and Enterprise edition. The MongoDB community edition is available for Windows, Linux as well as MacOS operating systems at https://www.mongodb.com/try/download/community
Windows
Download the latest version of MongoDB installer (https://fastdl.mongodb.org/windows/mongodb-windows-x86_64-7.0.4-signed.msi) and start the installation wizard by double-clicking the file.
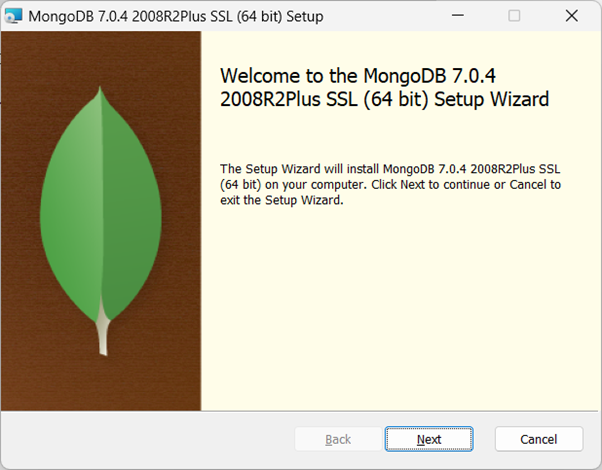
Choose Custom installation option to specify a convenient installation folder.
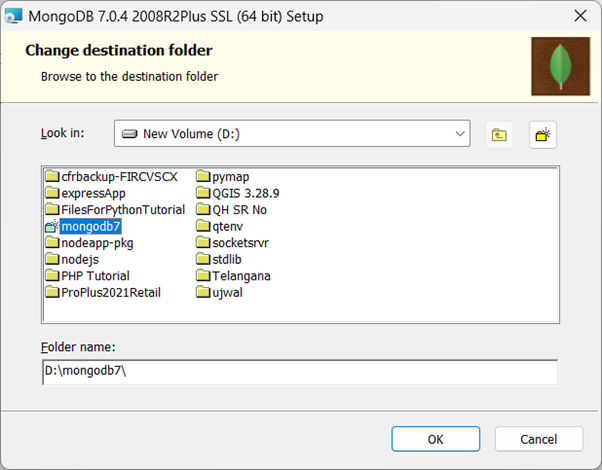
Uncheck the "Install MongoD as service" option, and accept the default data and log directory options.
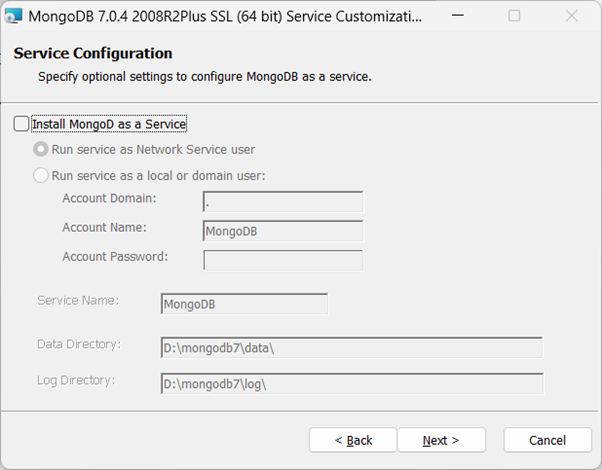
Go through the rest of steps in the installation wizard.
Create a directory "d:\data\db" and specify it as dbpath in the following command-line to start the MongoDB server −
"D:\mongodb7\mongod.exe" --dbpath="d:\data\db"
The installer also guides you to install MongoDB Compass, a GUI client for interacting with the MongoDB server. MongoDB server starts listening to the incoming connection requests at the 27017 port by default. Start the Compass app, and connect to the server using the default connection string as 'mongodb://localhost:27017'.
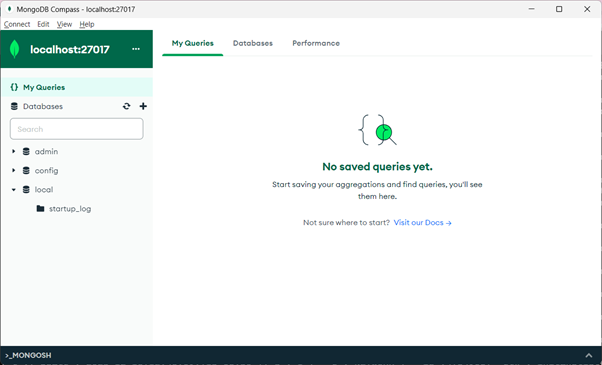
Ubuntu
To install MongoDB server on Ubuntu Linux, issue the following command to reload the local package database −
sudo apt-get update
You can now install either the latest stable version of MongoDB or a specific version of MongoDB.
To install the latest stable version, issue the following
sudo apt-get install -y mongodb-org
mongodb driver
Now we need to install the mongodb driver module from the NPM repository, so that a Node.js application could interact with MongoDB.
Initialize a new Node.js application in a new folder with the following command −
D:\nodejs\mongoproj>npm init -y Wrote to D:\nodejs\mongoproj\package.json: { "name": "mongoproj", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "keywords": [], "author": "", "license": "ISC" }
Install the mongodb driver module from NPM repository with the following command −
D:\nodejs\mongoproj>npm install mongodb
Connecting to MongoDB
Now we can establish connection with MongoDB server. First, import the MongoClient class from the mongodb module with the require() statement. Call its connect() method by passing the MongoDB server URL.
const { MongoClient } = require('mongodb'); // Connection URL const url = 'mongodb://localhost:27017'; const client = new MongoClient(url); // Database Name const dbName = 'myProject'; async function main() { // Use connect method to connect to the server await client.connect(); console.log('Connected successfully to server'); const db = client.db(dbName); const collection = db.collection('documents'); // the following code examples can be pasted here... return 'done.'; } main() .then(console.log) .catch(console.error) .finally(() => client.close());
Assuming that the above script is saved as app.js, run the application from command prompt −
PS D:\nodejs\mongoproj> node app.js Connected successfully to server done.