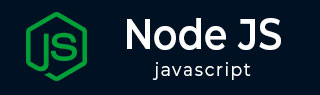
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - os.arch() Method
The os module of Node.js provides a bunch of operating system-related utility methods and properties.
The Node.js os.arch() method will return the CPU architecture of the computer for which the current Node.js is compiled.
There are a lot of CPU architectures like 'x32', 'x64', 'arm', 'arm64', 's390', 's390x', 'mipsel', 'ia32', 'mips', 'ppc' and 'ppc64'. To know the CPU architecture of our current computer, we need to compile the os.arch() method.
Syntax
Following is the syntax of the Node.js os.arch() method −
os.arch()
Parameters
This method doesn't accept any parameters.
Return value
This method returns the operating system CPU architecture for which the current node.js binary was compiled.
The possible values of OS (operating system) CPU architecture are −
'arm'
'arm64'
'ia32'
'mips'
'mipsel'
'ppc'
'ppc64'
's390'
's390x'
'x64'
Example
In the program below, we are trying to print the CPU architecture of the current system by using os.arch() method.
const os = require('os'); const {arch} = os; console.log(os.arch());
Output
If we compile and run the above program, the os.arch() method returns 'x64' as output because this method has returned the CPU architecture for the current computer.
x64
Example
In the example below,
We are performing a switch case to get the CPU architecture of the computer.
So the switch will check each case against the output string value of the os.arch() method until a match is found.
If nothing matches, the default condition will be printed.
const os = require('os'); let arch = os.arch(); switch(arch) { case 'arm': console.log("This is a 32-bit 'Advanced RISC Machine'."); break; case 'arm64': console.log("This is a 64-bit 'Advanced RISC Machine'."); break; case 'ia32': console.log("This is a 32-bit 'Intel Architecture'."); break; case 'mips': console.log("This is a 32-bit Microprocessor without Interlocked Pipelined Stages"); break; case 'mipsel': console.log("This is a 64-bit Microprocessor without Interlocked Pipelined Stages"); break; case 'ppc': console.log("This is a PowerPC Architecture."); break; case 'ppc64': console.log("This is a 64-bit PowerPC Architecture."); break; case 's390': console.log("This is a 31-bit IBM System/390, the third generation of the System/360 instruction set architecture"); break; case 's390x': console.log("This is a 64-bit IBM System/390, the third generation of the System/360 instruction set architecture."); break; case 'x64': console.log("This is a 64-bit extended system."); break; case 'x32': console.log("This is a 32-bit extended system."); break; }
Output
When we compile and run the above program, the output string value of the os.arch() method will be 'x64'. So the case 'x64 got matched and executed.
This is a 64-bit extended system.