
- AJAX Tutorial
- AJAX - Home
- AJAX - What is AJAX?
- AJAX - History
- AJAX - Dynamic Versus Static Sites
- AJAX - Technologies
- AJAX - Action
- AJAX - XMLHttpRequest
- AJAX - Sending Request
- AJAX - Types of requests
- AJAX - Handling Responses
- AJAX - Handling Binary Data
- AJAX - Submitting Forms
- AJAX - File Uploading
- AJAX - FormData Object
- AJAX - Send POST Requests
- AJAX - Send PUT Requests
- AJAX - Send JSON Data
- AJAX - Send Data Objects
- AJAX - Monitoring Progress
- AJAX - Status Codes
- AJAX - Applications
- AJAX - Browser Compatibility
- AJAX - Examples
- AJAX - Browser Support
- AJAX - XMLHttpRequest
- AJAX - Database Operations
- AJAX - Security
- AJAX - Issues
- Fetch API Basics
- Fetch API - Basics
- Fetch API Vs XMLHttpRequest
- Fetch API - Browser Compatibility
- Fetch API - Headers
- Fetch API - Request
- Fetch API - Response
- Fetch API - Body Data
- Fetch API - Credentials
- Fetch API - Send GET Requests
- Fetch API - Send POST Requests
- Fetch API - Send PUT Requests
- Fetch API - Send JSON Data
- Fetch API - Send Data Objects
- Fetch API - Custom Request Object
- Fetch API - Uploading Files
- Fetch API - Handling Binary Data
- Fetch API - Status Codes
- Stream API Basics
- Stream API - Basics
- Stream API - Readable Streams
- Stream API - Writeable Streams
- Stream API - Transform Streams
- Stream API - Request Object
- Stream API - Response Body
- Stream API - Error Handling
- AJAX Useful Resources
- AJAX - Quick Guide
- AJAX - Useful Resources
- AJAX - Discussion
AJAX - Handling Binary Data
Binary data is data that is in the binary format not in the text format. It includes images, audio, videos, and other file that are not in plain text. We can send and receive binary data in AJAX using an XMLHttpRequest object. While working with binary data in AJAX it is important to set a proper content type and response type headers. Hence for setting the header, we use the "Content-Type" header, here we set the proper MIME type to send binary data and set the "responseType" property to "arraybuffer" or "blob" which indicates that binary data is received.
Sending Binary Data
To send binary data we use send() method of XMLHttpRequest which can easily transmit binary data using ArrayBuffer, Blob or File object.
Example
In the following program, we create a program which will receive binary data from the server. So when we click on the button getBinaryData() function trigger. It uses an XMLHttpRequest object to get the data using the GET method from the given URL. In this function, we set the responseType property to arraybuffer which tells the browser that we have to only accept binary data in the response. When the request is completed an onload() function is called and inside this function, we check the status of the request if the response is successful, then the response is accessed as arraybuffer. Then convert arraybuffer into Uint8array using Unit8Array() function. It accesses the individual bytes of the binary data. After that, we will display the data on the HTML page.
<!DOCTYPE html> <html> <body> <script> function getBinaryData() { // Creating XMLHttpRequest object var myhttp = new XMLHttpRequest(); // Getting binary data myhttp.open("GET", "https://jsonplaceholder.typicode.com/posts", true); // Set responseType to arraybuffer. myhttp.responseType = "arraybuffer"; // Creating call back function myhttp.onload = (event) => { // IF the request is successful if (myhttp.status === 200){ var arraybuffer = myhttp.response; // Convert the arraybuffer into array var data = new Uint8Array(arraybuffer); // Display the binary data document.getElementById("example").innerHTML = data; console.log("Binary data Received"); }else{ console.log("Found error"); } }; // Sending the request to the server myhttp.send(); } </script> <div id="example"> <p>AJAX Example</p> <button type="button" onclick="getBinaryData()">Click Here</button> </div> </body> </html>
Output
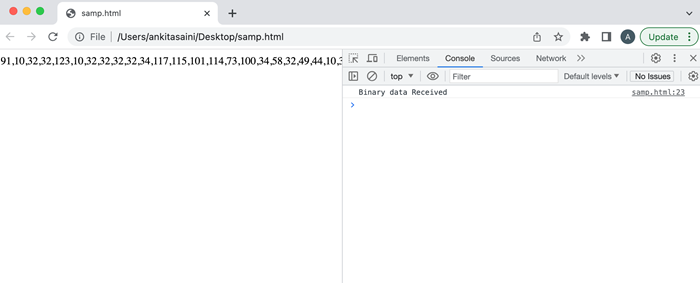
Conclusion
So this is how we can handle binary data. To handle binary data we need to convert binary data to an appropriate data format. We can also send binary data in the file, string, ArrayBuffer, and Blob. Now in the next article, we will learn how to submit forms using AJAX.
To Continue Learning Please Login