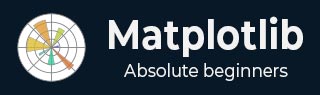
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Text
- Matplotlib - Images
- Matplotlib - Mathematical Expressions
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap -->
- Matplotlib Widgets
- Matplotlib - Cursor Widget
- Matplotlib - Annotated Cursor
- Matplotlib - Buttons Widget
- Matplotlib - Check Buttons
- Matplotlib - Lasso Selector
- Matplotlib - Menu Widget
- Matplotlib - Mouse Cursor
- Matplotlib - Multicursor
- Matplotlib - Polygon Selector
- Matplotlib - Radio Buttons
- Matplotlib - RangeSlider
- Matplotlib - Rectangle Selector
- Matplotlib - Ellipse Selector
- Matplotlib - Slider Widget
- Matplotlib - Span Selector
- Matplotlib - Textbox
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Image Thumbnail
Thumbnails, smaller and compressed versions of original images, serve as essential components in various applications, including image previews, web development, and optimizing the loading speed of web pages and applications with heavy images.
In this tutorial, we'll explore using Matplotlib to efficiently generate image thumbnails. Matplotlib takes the support of the Python Pillow library for image processing and allows us to easily generate thumbnails from existing images.
Image thumbnail in Matplotlib
Matplotlib offers the thumbnail() function within its image module to generate thumbnails with customizable parameters, allowing users to efficiently create scaled-down versions of images for various purposes.
Following is the syntax of the function −
Syntax
matplotlib.image.thumbnail(infile, thumbfile, scale=0.1, interpolation='bilinear', preview=False)
Here are the details of its parameters −
infile − The input image file. As you know that Matplotlib relies on Pillow for image reading, so it supports a wide range of file formats, including PNG, JPG, TIFF, and others.
thumbfile − The filename or file-like object where the thumbnail will be saved.
scale − The scale factor for the thumbnail. It determines the size reduction of the thumbnail relative to the original image. A smaller scale factor generates a smaller thumbnail.
interpolation − The interpolation scheme used in the resampling process. This parameter specifies the method used to estimate pixel values in the thumbnail.
preview − If set to True, the default backend (presumably a user interface backend) will be used, which may raise a figure if show() is called. If set to False, the figure is created using FigureCanvasBase, and the drawing backend is selected as Figure.savefig would normally do.
The function returns a Figure instance containing the thumbnail. This Figure object can be further manipulated or saved as needed.
Generating Thumbnails for a single image
Matplotlib supports various image formats like png, pdf, ps, eps svg, and more, making it flexible for different use cases.
Example
Here is an example that creates a thumbnail for a single .jpg image.
import matplotlib.pyplot as plt import matplotlib.image as mpimg input_image_path = "Images/Tajmahal.jpg" output_thumbnail_path = "Images/Tajmahal_thumbnail.jpg" # Load the original image img = mpimg.imread(input_image_path) # Create a thumbnail using Matplotlib thumb = mpimg.thumbnail(input_image_path, output_thumbnail_path, scale=0.15) print(f"Thumbnail generated for {input_image_path}. Saved to {output_thumbnail_path}")
Output
If you visit the folder where the images are saved you can observe both the original and output Thumbnail images as shown below −
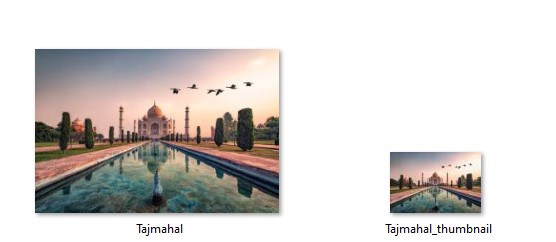
Generating Thumbnails for multiple Images
Consider a scenario where you have multiple images in a directory, and you want to generate thumbnails for each of them.
Example
Here is an example that creates thumbnails of multiple PNG images in a directory. Save the following script as generate_thumbnails.py file.
from argparse import ArgumentParser from pathlib import Path import sys import matplotlib.image as image parser = ArgumentParser(description="Generate thumbnails of PNG images in a directory.") parser.add_argument("imagedir", type=Path) args = parser.parse_args() if not args.imagedir.is_dir(): sys.exit(f"Could not find the input directory {args.imagedir}") outdir = Path("thumbs") outdir.mkdir(parents=True, exist_ok=True) for path in args.imagedir.glob("*.png"): outpath = outdir / path.name try: fig = image.thumbnail(path, outpath, scale=0.15) print(f"Saved thumbnail of {path} to {outpath}") except Exception as e: print(f"Error generating thumbnail for {path}: {e}")
Then run the script as follows in command prompt
python generate_thumbnails.py /path/to/all_images
Output
On executing the above program, will create a directory named "thumbs" and generate thumbnails for PNG images in your working directory. If it encounters an image with a different format, it will print an error message and continue processing other images.
Saved thumbnail of Images\3d_Star.png to thumbs\3d_Star.png Saved thumbnail of Images\balloons_noisy.png to thumbs\balloons_noisy.png Saved thumbnail of Images\binary image.png to thumbs\binary image.png Saved thumbnail of Images\black and white.png to thumbs\black and white.png Saved thumbnail of Images\Black1.png to thumbs\Black1.png Saved thumbnail of Images\Blank.png to thumbs\Blank.png Saved thumbnail of Images\Blank_img.png to thumbs\Blank_img.png Saved thumbnail of Images\Circle1.png to thumbs\Circle1.png Saved thumbnail of Images\ColorDots.png to thumbs\ColorDots.png Saved thumbnail of Images\colorful-shapes.png to thumbs\colorful-shapes.png Saved thumbnail of Images\dark_img1.png to thumbs\dark_img1.png Saved thumbnail of Images\dark_img2.png to thumbs\dark_img2.png Saved thumbnail of Images\decore.png to thumbs\decore.png Saved thumbnail of Images\deform.png to thumbs\deform.png Saved thumbnail of Images\Different shapes.png to thumbs\Different shapes.png Error generating thumbnail for Images\Different shapes_1.png: not a PNG file Saved thumbnail of Images\Ellipses.png to thumbs\Ellipses.png Error generating thumbnail for Images\Ellipses_and_circles.png: not a PNG file Saved thumbnail of Images\images (1).png to thumbs\images (1).png Saved thumbnail of Images\images (3).png to thumbs\images (3).png Saved thumbnail of Images\images.png to thumbs\images.png Saved thumbnail of Images\image___1.png to thumbs\image___1.png Saved thumbnail of Images\Lenna.png to thumbs\Lenna.png Saved thumbnail of Images\logo-footer-b.png to thumbs\logo-footer-b.png Saved thumbnail of Images\logo-w.png to thumbs\logo-w.png Saved thumbnail of Images\logo.png to thumbs\logo.png Saved thumbnail of Images\logo_Black.png to thumbs\logo_Black.png
To Continue Learning Please Login