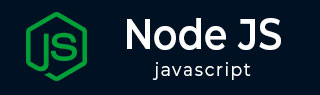
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - os.setPriority() Method
The Node.js os.setPriority() method will allow setting the scheduling priority of a process specified by the process ID (pid).
The priority is a whole number which specifies the scheduling process priority we want to set. The input priority must be in between −20 (high priority) and to 19 (low priority).
Because POSIX systems priority levels differ from those of Windows systems, Node.js provided us with constants that have different levels of priorities for processes and they are in os.constants.priority constants. Elevated privileges are required to set the process priority to the PRIORITY_HIGHEST on Windows. In case the user that's running the program doesn't have the privileges, then it will be reduced to PRIORITY_HIGH
Syntax
Following is the syntax of the Node.js os.setPriority() method −
os.setPriority([pid, ] priority)
Parameters
pid − (optional) This is the process ID, a whole number that identifies the running process for which the priority should be set. The default value is 0. This is an optional parameter.
priority − (required) The second argument is the priority, a whole integer that represents the scheduling of the process priority that we wish to set. The range goes from −20 for the highest priority to 19, which is the lowest. This is a required parameter.
Return value
This method doesn't return anything instead, it set the scheduling priority of the specified by pid.
Below are the priority constants that are provided by Node.js −
PRIORITY_LOW − This is the lowest process scheduling priority. On Windows, this corresponds to IDLE PRIORITY CLASS, and on all other platforms, it has a nice value of 19.
PRIORITY_BELOW_NORMAL − On windows, this corresponds to BELOW_NORMAL_PRIORITY_CLASS, and on all other platforms, it has a nice value of 10.
PRIORITY_NORMAL − This is the default process scheduling priority and this corresponds to NORMAL_PRIORITY_CLASS on windows. 0 is a nice value on all other platforms.
PRIORITY_ABOVE_NORMAL − This corresponds to ABOVE_NORMAL_PRIORITY_CLASS on windows and all other platforms, it has a nice value of -7.
PRIORITY_HIGH − On windows, this corresponds to HIGH_PRIORITY_CLASS, and on all other platforms, it has a nice value of -14.
PRIORITY_HIGHEST − This is the highest process scheduling priority and this corresponds to REALTIME_PRIORITY_CLASS on windows. −20 is a nice value on all other platforms.
Example
In the following example, we are trying to set the priority for the current process as 0.
const os = require('os'); os.setPriority(0); console.log(os.getPriority());
Output
/home/cg/root/63a002c52763b/main.js:3 os.setPriority(0); ^ TypeError: os.setPriority is not a function at Object.<anonymous> (/home/cg/root/63a002c52763b/main.js:3:4) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Note − To get the accurate result, better execute the above code in local.
After executing the above program, the setPriority() method sets the priority as 0 to the current process. As setPriority() doesn't return anything, we logged the getPriority() method and it prints the priority of the current process.
0
Example
In the example below, we are trying to set the priority for the current process as 19.
const os = require('os'); os.setPriority(19); console.log(os.getPriority());
Error
home/cg/root/63a002c52763b/main.js:3 os.setPriority(19); ^ TypeError: os.setPriority is not a function at Object.<anonymous> (/home/cg/root/63a002c52763b/main.js:3:4) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Note − To get the accurate result, better execute the above code in local.
After executing the above program, 19 sets as a scheduling priority to the pid. Then the. getPriority() method prints the scheduling priority of the current process.
19
Example
Note − In case the user that's running the program doesn't have the privileges, then it will be reduced to PRIORITY_HIGH.
In the following example, we are setting the priority for the current process as -20 by using setPriority() method.
const os = require('os'); os.setPriority(-20); console.log(os.getPriority());
Error
/home/cg/root/63a002c52763b/main.js:3 os.setPriority(-20); ^ TypeError: os.setPriority is not a function at Object.<anonymous> (/home/cg/root/63a002c52763b/main.js:3:4) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Note − To get the accurate result, better execute the above code in local.
After executing the above program, the user that's running the above program doesn't have the privileges, and it slowly reduced to PRIORITY_HIGH (-14).
-14
To Continue Learning Please Login