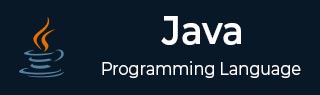
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine(JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - Thread Priority
Priority of a Thread in Java
Every Java thread has a priority that helps the operating system determine the order in which threads are scheduled. You can get and set the priority of a Thread. Thread class provides methods and constants for working with the priorities of a Thread.
Threads with higher priority are more important to a program and should be allocated processor time before lower-priority threads. However, thread priorities cannot guarantee the order in which threads execute and are very much platform dependent.
Built-in Property Constants of Thread Class
Java thread priorities are in the range between MIN_PRIORITY (a constant of 1) and MAX_PRIORITY (a constant of 10). By default, every thread is given priority NORM_PRIORITY (a constant of 5).
MIN_PRIORITY: Specifies the minimum priority that a thread can have.
NORM_PRIORITY: Specifies the default priority that a thread is assigned.
MAX_PRIORITY: Specifies the maximum priority that a thread can have.
Thread Priority Setter and Getter Methods
Thread.getPriority() Method: This method is used to get the priority of a thread.
Thread.setPriority() Method: This method is used to set the priority of a thread, it accepts the priority value and updates an existing priority with the given priority.
Example of Thread Priority in Java
In this example, we're showing a simple one thread program where we're not declaring any thread and checking the thread name and priority in the program execution.
package com.tutorialspoint; public class TestThread { public void printName() { System.out.println("Thread Name: " + Thread.currentThread().getName()); System.out.println("Thread Priority: " +Thread.currentThread().getPriority()); } public static void main(String args[]) { TestThread thread = new TestThread(); thread.printName(); } }
Output
Thread Name: main Thread Priority: 5
More Examples of Thread Priority
Example 1
In this example, we've created a ThreadDemo class which extends Thread class. We've created three threads. Each thread is assigned a priority. In run() method, we're printing the priorities and in output, it is reflecting in threads execution.
package com.tutorialspoint; class ThreadDemo extends Thread { ThreadDemo( ) { } public void run() { System.out.println("Thread Name: " + Thread.currentThread().getName() + ", Thread Priority: " +Thread.currentThread().getPriority()); for(int i = 4; i > 0; i--) { System.out.println("Thread: " + Thread.currentThread().getName() + ", " + i); } try { Thread.sleep(50); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public void start () { super.start(); } } public class TestThread { public static void main(String args[]) { ThreadDemo thread1 = new ThreadDemo(); ThreadDemo thread2 = new ThreadDemo(); ThreadDemo thread3 = new ThreadDemo(); thread1.setPriority(Thread.MAX_PRIORITY); thread2.setPriority(Thread.MIN_PRIORITY); thread3.setPriority(Thread.NORM_PRIORITY); thread1.start(); thread2.start(); thread3.start(); } }
Output
Thread Name: Thread-2, Thread Priority: 5 Thread Name: Thread-1, Thread Priority: 1 Thread Name: Thread-0, Thread Priority: 10 Thread: Thread-1, 4 Thread: Thread-2, 4 Thread: Thread-1, 3 Thread: Thread-0, 4 Thread: Thread-1, 2 Thread: Thread-2, 3 Thread: Thread-0, 3 Thread: Thread-0, 2 Thread: Thread-0, 1 Thread: Thread-2, 2 Thread: Thread-2, 1 Thread: Thread-1, 1
Example 2
In this example, we've created a ThreadDemo class which extends Thread class. We've created three threads. As we're not setting any priority, each thread has a normal priority. In run() method, we're printing the priorities and in output, threads are executing in any order.
package com.tutorialspoint; class ThreadDemo extends Thread { ThreadDemo( ) { } public void run() { System.out.println("Thread Name: " + Thread.currentThread().getName() + ", Thread Priority: " +Thread.currentThread().getPriority()); for(int i = 4; i > 0; i--) { System.out.println("Thread: " + Thread.currentThread().getName() + ", " + i); } try { Thread.sleep(50); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public void start () { super.start(); } } public class TestThread { public static void main(String args[]) { ThreadDemo thread1 = new ThreadDemo(); ThreadDemo thread2 = new ThreadDemo(); ThreadDemo thread3 = new ThreadDemo(); thread1.start(); thread2.start(); thread3.start(); } }
Output
Thread Name: Thread-1, Thread Priority: 5 Thread Name: Thread-2, Thread Priority: 5 Thread Name: Thread-0, Thread Priority: 5 Thread: Thread-2, 4 Thread: Thread-1, 4 Thread: Thread-1, 3 Thread: Thread-2, 3 Thread: Thread-0, 4 Thread: Thread-2, 2 Thread: Thread-1, 2 Thread: Thread-2, 1 Thread: Thread-0, 3 Thread: Thread-1, 1 Thread: Thread-0, 2 Thread: Thread-0, 1