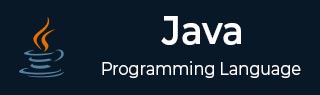
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine(JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - Encapsulation
Java Encapsulation
Encapsulation is one of the four fundamental OOP concepts. The other three are inheritance, polymorphism, and abstraction.
Encapsulation in Java is a mechanism of wrapping the data (variables) and code acting on the data (methods) together as a single unit. In encapsulation, the variables of a class will be hidden from other classes, and can be accessed only through the methods of their current class. Therefore, it is also known as data hiding.
Achieving Encapsulation in Java
To achieve encapsulation in Java −
Declare the variables of a class as private.
Provide public setter and getter methods to modify and view the variables values.
Java Encapsulation Example
Following is an example that demonstrates how to achieve Encapsulation in Java −
/* File name : EncapTest.java */ public class EncapTest { private String name; private String idNum; private int age; public int getAge() { return age; } public String getName() { return name; } public String getIdNum() { return idNum; } public void setAge( int newAge) { age = newAge; } public void setName(String newName) { name = newName; } public void setIdNum( String newId) { idNum = newId; } }
The public setXXX() and getXXX() methods are the access points of the instance variables of the EncapTest class. Normally, these methods are referred as getters and setters. Therefore, any class that wants to access the variables should access them through these getters and setters.
The variables of the EncapTest class can be accessed using the following program −
/* File name : RunEncap.java */ public class RunEncap { public static void main(String args[]) { EncapTest encap = new EncapTest(); encap.setName("James"); encap.setAge(20); encap.setIdNum("12343ms"); System.out.print("Name : " + encap.getName() + " Age : " + encap.getAge()); } } public class EncapTest { private String name; private String idNum; private int age; public int getAge() { return age; } public String getName() { return name; } public String getIdNum() { return idNum; } public void setAge( int newAge) { age = newAge; } public void setName(String newName) { name = newName; } public void setIdNum( String newId) { idNum = newId; } }
Output
Name : James Age : 20
Benefits of Encapsulation
The fields of a class can be made read-only or write-only.
A class can have total control over what is stored in its fields.
Java Encapsulation: Read-Only Class
A read-only class can have only getter methods to get the values of the attributes, there should not be any setter method.
Example: Creating Read-Only Class
In this example, we defined a class Person with two getter methods getName() and getAge(). These methods can be used to get the values of attributes declared as private in the class.
// Class "Person" class Person { private String name = "Robert"; private int age = 21; // Getter methods public String getName() { return this.name; } public int getAge() { return this.age; } } public class Main { public static void main(String args[]) { // Object to Person class Person per = new Person(); // Getting and printing the values System.out.println("Name of the person is: " + per.getName()); System.out.println("Age of the person is: " + per.getAge()); } }
Output
Name of the person is: Robert Age of the person is: 21
Java Encapsulation: Write-Only Class
A write-only class can have only setter methods to set the values of the attributes, there should not be any getter method.
Example: Creating Write-Only Class
In this example, we defined a class Person with two setter methods setName() and setAge(). These methods can be used to set the values of attributes declared as private in the class.
// Class "Person" class Person { private String name; private int age; // Setter Methods public void setName(String name) { this.name = name; } public void setAge(int age) { this.age = age; } } public class Main { public static void main(String args[]) { // Object to Person class Person per = new Person(); // Setting the values per.setName("Robert"); per.setAge(21); } }
Java Encapsulation: More Examples
Example 1: Person Class (Fully Encapsulated)
This example creates a fully encapsulated class named "Person". This class has private class attributes, setter, and getter methods.
// Class "Person" class Person { private String name; private int age; // Setter Methods public void setName(String name) { this.name = name; } public void setAge(int age) { this.age = age; } // Getter methods public String getName() { return this.name; } public int getAge() { return this.age; } } // The Main class to test encapsulated class "Person" public class Main { public static void main(String args[]) { // Objects to Person class Person per1 = new Person(); Person per2 = new Person(); // Setting the values per1.setName("Robert"); per1.setAge(21); per2.setName("Riyan"); per2.setAge(22); // Printing the values System.out.println("Person 1: Name : " + per1.getName() + " Age : " + per1.getAge()); System.out.println("Person 2: Name : " + per2.getName() + " Age : " + per2.getAge()); } }
Output
Person 1: Name : Robert Age : 21 Person 2: Name : Riyan Age : 22
Example 2: Employee Class (Fully Encapsulated)
This example creates a fully encapsulated class named "Employee". This class has private class attributes, setter, and getter methods.
// Class "Employee" class Employee { private String emp_name; private String emp_id; private double net_salary; // Constructor public Employee(String emp_name, String emp_id, double net_salary) { this.emp_name = emp_name; this.emp_id = emp_id; this.net_salary = net_salary; } // Getter methods public String getEmpName() { return emp_name; } public String getEmpId() { return emp_id; } public double getSalary() { return net_salary; } // Setter methods public void setEmpName(String emp_name) { this.emp_name = emp_name; } public void setEmpId(String emp_id) { this.emp_id = emp_id; } public void setSalary(double net_salary) { this.net_salary = net_salary; } } // The Main class to test encapsulated class "Employee" public class Main { public static void main(String args[]) { // Objects to Employee class // First object - setting values using constructor Employee emp = new Employee("Robert", "EMP001", 75450.00); // Printing data System.out.println("Employee (Intial Values):"); System.out.println(emp.getEmpId() + " , " + emp.getEmpName() + " , " + emp.getSalary()); // Updating values using setter methods emp.setEmpName("Riyan"); emp.setEmpId("EMP002"); emp.setSalary(90500.00); // Printing data System.out.println("Employee (Updated Values):"); System.out.println(emp.getEmpId() + " , " + emp.getEmpName() + " , " + emp.getSalary()); } }
Output
Employee (Intial Values): EMP001 , Robert , 75450.0 Employee (Updated Values): EMP002 , Riyan , 90500.0