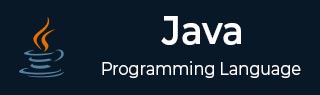
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine(JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - Main Thread
Main Thread in Java
Whenever we run a Java program, main thread is created automatically. This thread is responsible for execution of java program. Java runtime searches for main method to execute and create a main thread based on it. If we're creating multiple threads then all child threads will be spawned from main thread. This main thread is the first thread to be created and is generally the last thread and it is used to perform shut down tasks.
How to Control Main Thread?
The main thread is created by the JVM automatically when a program starts. But you can control a Main thread by using different Thread methods and techniques.
The following are some of the methods for controlling the Main thread.
Example of Java Main Thread
In this example, we're showing a simple one thread program where we're not declaring any thread and checking the thread name in the program execution.
package com.tutorialspoint; public class TestThread { public void printName() { System.out.println("Thread Name: " + Thread.currentThread().getName()); System.out.println("Thread Priority: " +Thread.currentThread().getPriority()); } public static void main(String args[]) { TestThread thread = new TestThread(); thread.printName(); } }
Output
Thread Name: main Thread Priority: 5
More Example of Main Thread
Example
In this example, we've created a ThreadDemo class which extends Thread class. We're not passing any name to the Thread and it will print the default names assigned to the threads by the system. In main method, we've created two threads. In output, you can check, current thread name is printed as main while threads are created using constructor() method call.
package com.tutorialspoint; class ThreadDemo extends Thread { ThreadDemo( ) { System.out.println("Thread: " + Thread.currentThread().getName() + ", " + "State: New"); } public void run() { System.out.println("Thread: " + Thread.currentThread().getName() + ", " + "State: Running"); for(int i = 4; i > 0; i--) { System.out.println("Thread: " + Thread.currentThread().getName() + ", " + i); } System.out.println("Thread: " + Thread.currentThread().getName() + ", " + "State: Dead"); } public void start () { System.out.println("Thread: " + Thread.currentThread().getName() + ", " + "State: Start"); super.start(); } } public class TestThread { public static void main(String args[]) { ThreadDemo thread1 = new ThreadDemo(); ThreadDemo thread2 = new ThreadDemo(); thread1.start(); thread2.start(); } }
Output
Thread: main, State: New Thread: main, State: New Thread: main, State: Start Thread: main, State: Start Thread: Thread-0, State: Running Thread: Thread-0, 4 Thread: Thread-0, 3 Thread: Thread-1, State: Running Thread: Thread-1, 4 Thread: Thread-0, 2 Thread: Thread-1, 3 Thread: Thread-0, 1 Thread: Thread-1, 2 Thread: Thread-0, State: Dead Thread: Thread-1, 1 Thread: Thread-1, State: Dead
Example 2
In this example, we've created a ThreadDemo class which extends Thread class. We're not passing any name to the Thread and it will print the default names assigned to the threads by the system. In main method, we've created two threads. In output, you can check, current thread name is printed as main while threads are created using constructor() method call. In the end of main method, we're printing the state of main thread.
package com.tutorialspoint; class ThreadDemo extends Thread { ThreadDemo( ) { System.out.println("Thread: " + Thread.currentThread().getName() + ", " + "State: New"); } public void run() { System.out.println("Thread: " + Thread.currentThread().getName() + ", " + "State: Running"); for(int i = 4; i > 0; i--) { System.out.println("Thread: " + Thread.currentThread().getName() + ", " + i); } System.out.println("Thread: " + Thread.currentThread().getName() + ", " + "State: Dead"); } public void start () { System.out.println("Thread: " + Thread.currentThread().getName() + ", " + "State: Start"); super.start(); } } public class TestThread { public static void main(String args[]) { ThreadDemo thread1 = new ThreadDemo(); ThreadDemo thread2 = new ThreadDemo(); thread1.start(); thread2.start(); System.out.println("Thread: " + Thread.currentThread().getName() + ", " + "State: Dead"); } }
Output
Thread: main, State: New Thread: main, State: New Thread: main, State: Start Thread: main, State: Start Thread: Thread-0, State: Running Thread: main, State: Dead Thread: Thread-1, State: Running Thread: Thread-0, 4 Thread: Thread-1, 4 Thread: Thread-1, 3 Thread: Thread-1, 2 Thread: Thread-1, 1 Thread: Thread-1, State: Dead Thread: Thread-0, 3 Thread: Thread-0, 2 Thread: Thread-0, 1 Thread: Thread-0, State: Dead
In this output, you can check that main thread was finished in earlier stages but threads were still running and finished their execution.