
- Android Basics
- Android - Home
- Android - Overview
- Android - Environment Setup
- Android - Architecture
- Android - Application Components
- Android - Hello World Example
- Android - Resources
- Android - Activities
- Android - Services
- Android - Broadcast Receivers
- Android - Content Providers
- Android - Fragments
- Android - Intents/Filters
- Android - User Interface
- Android - UI Layouts
- Android - UI Controls
- Android - Event Handling
- Android - Styles and Themes
- Android - Custom Components
- Android Advanced Concepts
- Android - Drag and Drop
- Android - Notifications
- Location Based Services
- Android - Sending Email
- Android - Sending SMS
- Android - Phone Calls
- Publishing Android Application
- Android Useful Examples
- Android - Alert Dialoges
- Android - Animations
- Android - Audio Capture
- Android - AudioManager
- Android - Auto Complete
- Android - Best Practices
- Android - Bluetooth
- Android - Camera
- Android - Clipboard
- Android - Custom Fonts
- Android - Data Backup
- Android - Developer Tools
- Android - Emulator
- Android - Facebook Integration
- Android - Gestures
- Android - Google Maps
- Android - Image Effects
- Android - ImageSwitcher
- Android - Internal Storage
- Android - JetPlayer
- Android - JSON Parser
- Android - Linkedin Integration
- Android - Loading Spinner
- Android - Localization
- Android - Login Screen
- Android - MediaPlayer
- Android - Multitouch
- Android - Navigation
- Android - Network Connection
- Android - NFC Guide
- Android - PHP/MySQL
- Android - Progress Circle
- Android - ProgressBar
- Android - Push Notification
- Android - RenderScript
- Android - RSS Reader
- Android - Screen Cast
- Android - SDK Manager
- Android - Sensors
- Android - Session Management
- Android - Shared Preferences
- Android - SIP Protocol
- Android - Spelling Checker
- Android - SQLite Database
- Android - Support Library
- Android - Testing
- Android - Text to Speech
- Android - TextureView
- Android - Twitter Integration
- Android - UI Design
- Android - UI Patterns
- Android - UI Testing
- Android - WebView Layout
- Android - Wi-Fi
- Android - Widgets
- Android - XML Parsers
- Android Useful Resources
- Android - Questions and Answers
- Android - Useful Resources
- Android - Discussion
Android - XML Parser
XML stands for Extensible Mark-up Language.XML is a very popular format and commonly used for sharing data on the internet. This chapter explains how to parse the XML file and extract necessary information from it.
Android provides three types of XML parsers which are DOM,SAX and XMLPullParser. Among all of them android recommend XMLPullParser because it is efficient and easy to use. So we are going to use XMLPullParser for parsing XML.
The first step is to identify the fields in the XML data in which you are interested in. For example. In the XML given below we interested in getting temperature only.
<?xml version="1.0"?> <current> <city id="2643743" name="London"> <coord lon="-0.12574" lat="51.50853"/> <country>GB</country> <sun rise="2013-10-08T06:13:56" set="2013-10-08T17:21:45"/> </city> <temperature value="289.54" min="289.15" max="290.15" unit="kelvin"/> <humidity value="77" unit="%"/> <pressure value="1025" unit="hPa"/> </current>
XML - Elements
An xml file consist of many components. Here is the table defining the components of an XML file and their description.
Sr.No | Component & description |
---|---|
1 |
Prolog An XML file starts with a prolog. The first line that contains the information about a file is prolog |
2 |
Events An XML file has many events. Event could be like this. Document starts , Document ends, Tag start , Tag end and Text e.t.c |
3 |
Text Apart from tags and events, and xml file also contains simple text. Such as GB is a text in the country tag. |
4 |
Attributes Attributes are the additional properties of a tag such as value e.t.c |
XML - Parsing
In the next step, we will create XMLPullParser object , but in order to create that we will first create XmlPullParserFactory object and then call its newPullParser() method to create XMLPullParser. Its syntax is given below −
private XmlPullParserFactory xmlFactoryObject = XmlPullParserFactory.newInstance(); private XmlPullParser myparser = xmlFactoryObject.newPullParser();
The next step involves specifying the file for XmlPullParser that contains XML. It could be a file or could be a Stream. In our case it is a stream.Its syntax is given below −
myparser.setInput(stream, null);
The last step is to parse the XML. An XML file consist of events, Name, Text, AttributesValue e.t.c. So XMLPullParser has a separate function for parsing each of the component of XML file. Its syntax is given below −
int event = myParser.getEventType(); while (event != XmlPullParser.END_DOCUMENT) { String name=myParser.getName(); switch (event){ case XmlPullParser.START_TAG: break; case XmlPullParser.END_TAG: if(name.equals("temperature")){ temperature = myParser.getAttributeValue(null,"value"); } break; } event = myParser.next(); }
The method getEventType returns the type of event that happens. e.g: Document start , tag start e.t.c. The method getName returns the name of the tag and since we are only interested in temperature , so we just check in conditional statement that if we got a temperature tag , we call the method getAttributeValue to return us the value of temperature tag.
Apart from the these methods, there are other methods provided by this class for better parsing XML files. These methods are listed below −
Sr.No | Method & description |
---|---|
1 |
getAttributeCount() This method just Returns the number of attributes of the current start tag |
2 |
getAttributeName(int index) This method returns the name of the attribute specified by the index value |
3 |
getColumnNumber() This method returns the Returns the current column number, starting from 0. |
4 |
getDepth() This method returns Returns the current depth of the element. |
5 |
getLineNumber() Returns the current line number, starting from 1. |
6 |
getNamespace() This method returns the name space URI of the current element. |
7 |
getPrefix() This method returns the prefix of the current element |
8 |
getName() This method returns the name of the tag |
9 |
getText() This method returns the text for that particular element |
10 |
isWhitespace() This method checks whether the current TEXT event contains only whitespace characters. |
Example
Here is an example demonstrating the use of XML DOM Parser. It creates a basic application that allows you to parse XML.
To experiment with this example, you can run this on an actual device or in an emulator.
Steps | Description |
---|---|
1 | You will use Android studio to create an Android application under a package com.example.sairamkrishna.myapplication. |
2 | Modify src/MainActivity.java file to add necessary code. |
3 | Modify the res/layout/activity_main to add respective XML components |
4 | Create a new XML file under Assets Folder/file.xml |
5 | Modify AndroidManifest.xml to add necessary internet permission |
6 | Run the application and choose a running android device and install the application on it and verify the results |
Following is the content of the modified main activity file MainActivity.java.
package com.example.sairamkrishna.myapplication; import java.io.InputStream; import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import org.w3c.dom.Document; import org.w3c.dom.Element; import org.w3c.dom.Node; import org.w3c.dom.NodeList; import android.app.Activity; import android.os.Bundle; import android.widget.TextView; public class MainActivity extends Activity { TextView tv1; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); tv1=(TextView)findViewById(R.id.textView1); try { InputStream is = getAssets().open("file.xml"); DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance(); DocumentBuilder dBuilder = dbFactory.newDocumentBuilder(); Document doc = dBuilder.parse(is); Element element=doc.getDocumentElement(); element.normalize(); NodeList nList = doc.getElementsByTagName("employee"); for (int i=0; i<nList.getLength(); i++) { Node node = nList.item(i); if (node.getNodeType() == Node.ELEMENT_NODE) { Element element2 = (Element) node; tv1.setText(tv1.getText()+"\nName : " + getValue("name", element2)+"\n"); tv1.setText(tv1.getText()+"Surname : " + getValue("surname", element2)+"\n"); tv1.setText(tv1.getText()+"-----------------------"); } } } catch (Exception e) {e.printStackTrace();} } private static String getValue(String tag, Element element) { NodeList nodeList = element.getElementsByTagName(tag).item(0).getChildNodes(); Node node = nodeList.item(0); return node.getNodeValue(); } }
Following is the content of Assets/file.xml.
<?xml version="1.0"?> <records> <employee> <name>Sairamkrishna</name> <surname>Mammahe</surname> <salary>50000</salary> </employee> <employee> <name>Gopal </name> <surname>Varma</surname> <salary>60000</salary> </employee> <employee> <name>Raja</name> <surname>Hr</surname> <salary>70000</salary> </employee> </records>
Following is the modified content of the xml res/layout/activity_main.xml.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".MainActivity"> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </RelativeLayout>
Following is the content of AndroidManifest.xml file.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.sairamkrishna.myapplication" > <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Let's try to run our application we just modified. I assume you had created your AVD while doing environment setup. To run the app from Android studio, open one of your project's activity files and click Run icon from the toolbar. Android studio installs the app on your AVD and starts it and if everything is fine with your setup and application, it will display following Emulator window −
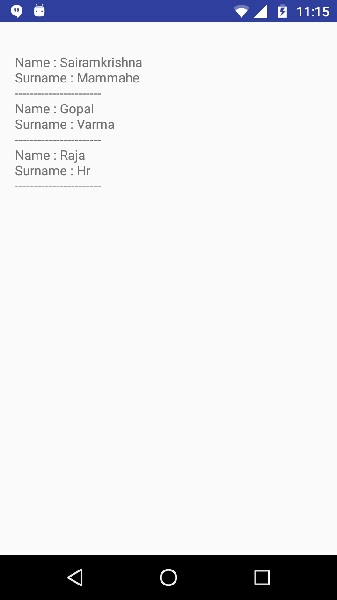