
- Android Basics
- Android - Home
- Android - Overview
- Android - Environment Setup
- Android - Architecture
- Android - Application Components
- Android - Hello World Example
- Android - Resources
- Android - Activities
- Android - Services
- Android - Broadcast Receivers
- Android - Content Providers
- Android - Fragments
- Android - Intents/Filters
- Android - User Interface
- Android - UI Layouts
- Android - UI Controls
- Android - Event Handling
- Android - Styles and Themes
- Android - Custom Components
- Android Advanced Concepts
- Android - Drag and Drop
- Android - Notifications
- Location Based Services
- Android - Sending Email
- Android - Sending SMS
- Android - Phone Calls
- Publishing Android Application
- Android Useful Examples
- Android - Alert Dialoges
- Android - Animations
- Android - Audio Capture
- Android - AudioManager
- Android - Auto Complete
- Android - Best Practices
- Android - Bluetooth
- Android - Camera
- Android - Clipboard
- Android - Custom Fonts
- Android - Data Backup
- Android - Developer Tools
- Android - Emulator
- Android - Facebook Integration
- Android - Gestures
- Android - Google Maps
- Android - Image Effects
- Android - ImageSwitcher
- Android - Internal Storage
- Android - JetPlayer
- Android - JSON Parser
- Android - Linkedin Integration
- Android - Loading Spinner
- Android - Localization
- Android - Login Screen
- Android - MediaPlayer
- Android - Multitouch
- Android - Navigation
- Android - Network Connection
- Android - NFC Guide
- Android - PHP/MySQL
- Android - Progress Circle
- Android - ProgressBar
- Android - Push Notification
- Android - RenderScript
- Android - RSS Reader
- Android - Screen Cast
- Android - SDK Manager
- Android - Sensors
- Android - Session Management
- Android - Shared Preferences
- Android - SIP Protocol
- Android - Spelling Checker
- Android - SQLite Database
- Android - Support Library
- Android - Testing
- Android - Text to Speech
- Android - TextureView
- Android - Twitter Integration
- Android - UI Design
- Android - UI Patterns
- Android - UI Testing
- Android - WebView Layout
- Android - Wi-Fi
- Android - Widgets
- Android - XML Parsers
- Android Useful Resources
- Android - Questions and Answers
- Android - Useful Resources
- Android - Discussion
Android - Navigation
In this chapter, we will see that how you can provide navigation forward and backward between an application. We will first look at how to provide up navigation in an application.
Providing Up Navigation
The up navigation will allow our application to move to previous activity from the next activity. It can be done like this.
To implement Up navigation, the first step is to declare which activity is the appropriate parent for each activity. You can do it by specifying parentActivityName attribute in an activity. Its syntax is given below −
android:parentActivityName = "com.example.test.MainActivity"
After that you need to call setDisplayHomeAsUpEnabled method of getActionBar() in the onCreate method of the activity. This will enable the back button in the top action bar.
getActionBar().setDisplayHomeAsUpEnabled(true);
The last thing you need to do is to override onOptionsItemSelected method. when the user presses it, your activity receives a call to onOptionsItemSelected(). The ID for the action is android.R.id.home.Its syntax is given below −
public boolean onOptionsItemSelected(MenuItem item) { switch (item.getItemId()) { case android.R.id.home: NavUtils.navigateUpFromSameTask(this); return true; } }
Handling device back button
Since you have enabled your back button to navigate within your application, you might want to put the application close function in the device back button.
It can be done by overriding onBackPressed and then calling moveTaskToBack and finish method. Its syntax is given below −
@Override public void onBackPressed() { moveTaskToBack(true); MainActivity2.this.finish(); }
Apart from this setDisplayHomeAsUpEnabled method, there are other methods available in ActionBar API class. They are listed below −
Sr.No | Method & description |
---|---|
1 |
addTab(ActionBar.Tab tab, boolean setSelected) This method adds a tab for use in tabbed navigation mode |
2 |
getSelectedTab() This method returns the currently selected tab if in tabbed navigation mode and there is at least one tab present |
3 |
hide() This method hide the ActionBar if it is currently showing |
4 |
removeAllTabs() This method remove all tabs from the action bar and deselect the current tab |
5 |
selectTab(ActionBar.Tab tab) This method select the specified tab |
Example
The below example demonstrates the use of Navigation. It crates a basic application that allows you to navigate within your application.
To experiment with this example, you need to run this on an actual device or in an emulator.
Steps | Description |
---|---|
1 | You will use Android studio to create an Android application under a package com.example.sairamkrishna.myapplication. |
2 | Modify src/MainActivity.java file to add Activity code. |
3 | Create a new activity with the name of second_main.java and edit it to add activity code. |
4 | Modify layout XML file res/layout/activity_main.xml add any GUI component if required. |
5 | Modify layout XML file res/layout/second.xml add any GUI component if required. |
6 | Modify AndroidManifest.xml to add necessary code. |
7 | Run the application and choose a running android device and install the application on it and verify the results. |
Here is the content of src/MainActivity.java.
package com.example.sairamkrishna.myapplication; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; public class MainActivity extends Activity { Button b1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); b1 = (Button) findViewById(R.id.button); b1.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent in=new Intent(MainActivity.this,second_main.class); startActivity(in); } }); } }
Here is the content of src/second_main.java.
package com.example.sairamkrishna.myapplication; import android.app.Activity; import android.os.Bundle; import android.webkit.WebView; import android.webkit.WebViewClient; /** * Created by Sairamkrishna on 4/6/2015. */ public class second_main extends Activity { WebView wv; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main_activity2); wv = (WebView) findViewById(R.id.webView); wv.setWebViewClient(new MyBrowser()); wv.getSettings().setLoadsImagesAutomatically(true); wv.getSettings().setJavaScriptEnabled(true); wv.loadUrl("https://www.tutorialspoint.com"); } private class MyBrowser extends WebViewClient { @Override public boolean shouldOverrideUrlLoading(WebView view, String url) { view.loadUrl(url); return true; } } }
Here is the content of activity_main.xml.
In the below code abcindicates the logo of tutorialspoint.com
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity" android:transitionGroup="true"> <TextView android:text="Navigation example" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/textview" android:textSize="35dp" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Tutorials point" android:id="@+id/textView" android:layout_below="@+id/textview" android:layout_centerHorizontal="true" android:textColor="#ff7aff24" android:textSize="35dp" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/imageView" android:src="@drawable/abc" android:layout_below="@+id/textView" android:layout_centerHorizontal="true" android:theme="@style/Base.TextAppearance.AppCompat" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="first page" android:id="@+id/button" android:layout_below="@+id/imageView" android:layout_alignRight="@+id/textView" android:layout_alignEnd="@+id/textView" android:layout_marginTop="61dp" android:layout_alignLeft="@+id/imageView" android:layout_alignStart="@+id/imageView" /> </RelativeLayout>
Here is the content of activity_main_activity2.xml.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent" android:weightSum="1"> <WebView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/webView" android:layout_gravity="center_horizontal" android:layout_weight="1.03" /> </LinearLayout>
Here is the content of Strings.xml.
<resources> <string name="app_name">My Application</string> </resources>
Here is the content of AndroidManifest.xml.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.sairamkrishna.myapplication" > <uses-permission android:name="android.permission.INTERNET"></uses-permission> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".second_main"></activity> </application> </manifest>
Let's try to run your application. I assume you had created your AVD while doing environment setup. To run the app from Android studio, open one of your project's activity files and click Run icon from the toolbar. Android studio installs the app on your AVD and starts it and if everything is fine with your setup and application, it will display following Emulator window−
Now just press on button and the following screen will be shown to you.

Second activity contains webview, it has redirected to tutorialspoint.com as shown below
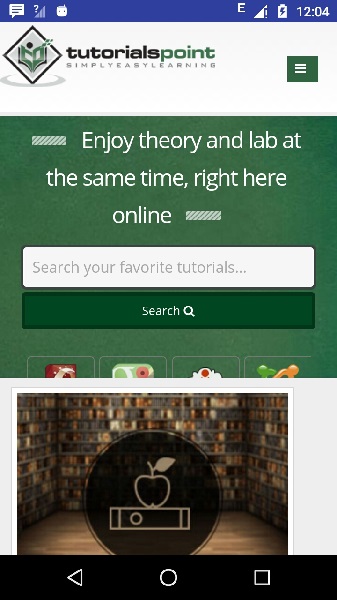