
- Android Basics
- Android - Home
- Android - Overview
- Android - Environment Setup
- Android - Architecture
- Android - Application Components
- Android - Hello World Example
- Android - Resources
- Android - Activities
- Android - Services
- Android - Broadcast Receivers
- Android - Content Providers
- Android - Fragments
- Android - Intents/Filters
- Android - User Interface
- Android - UI Layouts
- Android - UI Controls
- Android - Event Handling
- Android - Styles and Themes
- Android - Custom Components
- Android Advanced Concepts
- Android - Drag and Drop
- Android - Notifications
- Location Based Services
- Android - Sending Email
- Android - Sending SMS
- Android - Phone Calls
- Publishing Android Application
- Android Useful Examples
- Android - Alert Dialoges
- Android - Animations
- Android - Audio Capture
- Android - AudioManager
- Android - Auto Complete
- Android - Best Practices
- Android - Bluetooth
- Android - Camera
- Android - Clipboard
- Android - Custom Fonts
- Android - Data Backup
- Android - Developer Tools
- Android - Emulator
- Android - Facebook Integration
- Android - Gestures
- Android - Google Maps
- Android - Image Effects
- Android - ImageSwitcher
- Android - Internal Storage
- Android - JetPlayer
- Android - JSON Parser
- Android - Linkedin Integration
- Android - Loading Spinner
- Android - Localization
- Android - Login Screen
- Android - MediaPlayer
- Android - Multitouch
- Android - Navigation
- Android - Network Connection
- Android - NFC Guide
- Android - PHP/MySQL
- Android - Progress Circle
- Android - ProgressBar
- Android - Push Notification
- Android - RenderScript
- Android - RSS Reader
- Android - Screen Cast
- Android - SDK Manager
- Android - Sensors
- Android - Session Management
- Android - Shared Preferences
- Android - SIP Protocol
- Android - Spelling Checker
- Android - SQLite Database
- Android - Support Library
- Android - Testing
- Android - Text to Speech
- Android - TextureView
- Android - Twitter Integration
- Android - UI Design
- Android - UI Patterns
- Android - UI Testing
- Android - WebView Layout
- Android - Wi-Fi
- Android - Widgets
- Android - XML Parsers
- Android Useful Resources
- Android - Questions and Answers
- Android - Useful Resources
- Android - Discussion
Android - Custom Fonts
In android, you can define your own custom fonts for the strings in your application. You just need to download the required font from the internet, and then place it in assets/fonts folder.
After putting fonts in the assets folder under fonts folder, you can access it in your java code through Typeface class. First , get the reference of the text view in the code. Its syntax is given below −
TextView tx = (TextView)findViewById(R.id.textview1);
The next thing you need to do is to call static method of Typeface class createFromAsset() to get your custom font from assets. Its syntax is given below −
Typeface custom_font = Typeface.createFromAsset(getAssets(), "fonts/font name.ttf");
The last thing you need to do is to set this custom font object to your TextView Typeface property. You need to call setTypeface() method to do that. Its syntax is given below −
tx.setTypeface(custom_font);
Apart from these Methods, there are other methods defined in the Typeface class , that you can use to handle Fonts more effectively.
Sr.No | Method & description |
---|---|
1 |
create(String familyName, int style) Create a Typeface object given a family name, and option style information |
2 |
create(Typeface family, int style) Create a Typeface object that best matches the specified existing Typeface and the specified Style |
3 |
createFromFile(String path) Create a new Typeface from the specified font file |
4 |
defaultFromStyle(int style) Returns one of the default Typeface objects, based on the specified style |
5 |
getStyle() Returns the Typeface's intrinsic style attributes |
Example
Here is an example demonstrating the use of Typeface to handle CustomFont. It creates a basic application that displays a custom font that you specified in the fonts file.
To experiment with this example, you can run this on an actual device or in an emulator.
Steps | Description |
---|---|
1 | You will use Android studio IDE to create an Android application under a package com.example.sairamkrishna.myapplication. |
2 | Download a font from internet and put it under assets/fonts folder. |
3 | Modify src/MainActivity.java file to add necessary code. |
4 | Modify the res/layout/activity_main to add respective XML components |
5 | Run the application and choose a running android device and install the application on it and verify the results |
Before entering to code part add fonts in assests folder from windows explorer.
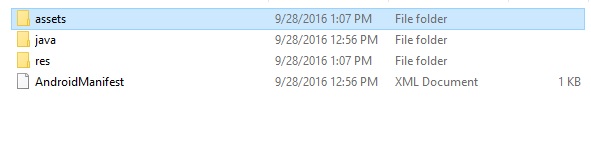
Following is the content of the modified main activity file MainActivity.java.
package com.example.sairamkrishna.myapplication; import android.graphics.Typeface; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.widget.TextView; public class MainActivity extends ActionBarActivity { TextView tv1,tv2; protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); tv1=(TextView)findViewById(R.id.textView3); tv2=(TextView)findViewById(R.id.textView4); Typeface face= Typeface.createFromAsset(getAssets(), "font/font.ttf"); tv1.setTypeface(face); Typeface face1= Typeface.createFromAsset(getAssets(), "font/font1.ttf"); tv2.setTypeface(face1); } }
Following is the modified content of the xml activity_main.xml.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Typeface" android:id="@+id/textView" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:textSize="30dp" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Tutorials Point" android:id="@+id/textView2" android:layout_below="@+id/textView" android:layout_centerHorizontal="true" android:textSize="35dp" android:textColor="#ff16ff01" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Tutorials Point" android:id="@+id/textView3" android:layout_centerVertical="true" android:textSize="45dp" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Tutorials Point" android:id="@+id/textView4" android:layout_below="@+id/textView3" android:layout_alignLeft="@+id/textView3" android:layout_alignStart="@+id/textView3" android:layout_marginTop="73dp" android:textSize="45dp" /> </RelativeLayout>
Following is the content of the res/values/string.xml.
<resources> <string name="app_name">My Application</string> </resources>
Following is the content of AndroidManifest.xml file.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.sairamkrishna.myapplication" > <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Let's try to run our Custom Font application we just modified. I assume you had created your AVD while doing environment setup. To run the app from Android studio, open one of your project's activity files and click Run icon from the toolbar.Android studio installs the app on your AVD and starts it and if everything is fine with your setup and application, it will display following Emulator window −
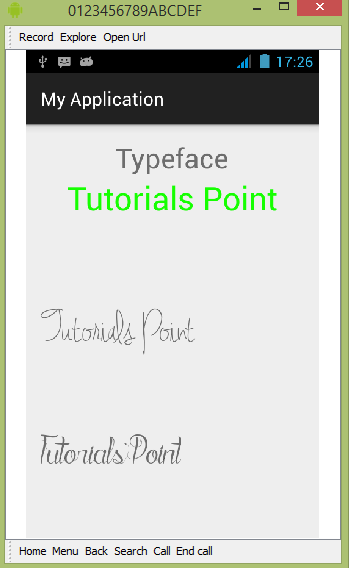
As you can see that the text that appeared on the AVD has not a default android font, rather it has the custom font that you specified in the fonts folder.
Note − You need to take care of the size and the character supported by the font , when using custom fonts.