
- Android Basics
- Android - Home
- Android - Overview
- Android - Environment Setup
- Android - Architecture
- Android - Application Components
- Android - Hello World Example
- Android - Resources
- Android - Activities
- Android - Services
- Android - Broadcast Receivers
- Android - Content Providers
- Android - Fragments
- Android - Intents/Filters
- Android - User Interface
- Android - UI Layouts
- Android - UI Controls
- Android - Event Handling
- Android - Styles and Themes
- Android - Custom Components
- Android Advanced Concepts
- Android - Drag and Drop
- Android - Notifications
- Location Based Services
- Android - Sending Email
- Android - Sending SMS
- Android - Phone Calls
- Publishing Android Application
- Android Useful Examples
- Android - Alert Dialoges
- Android - Animations
- Android - Audio Capture
- Android - AudioManager
- Android - Auto Complete
- Android - Best Practices
- Android - Bluetooth
- Android - Camera
- Android - Clipboard
- Android - Custom Fonts
- Android - Data Backup
- Android - Developer Tools
- Android - Emulator
- Android - Facebook Integration
- Android - Gestures
- Android - Google Maps
- Android - Image Effects
- Android - ImageSwitcher
- Android - Internal Storage
- Android - JetPlayer
- Android - JSON Parser
- Android - Linkedin Integration
- Android - Loading Spinner
- Android - Localization
- Android - Login Screen
- Android - MediaPlayer
- Android - Multitouch
- Android - Navigation
- Android - Network Connection
- Android - NFC Guide
- Android - PHP/MySQL
- Android - Progress Circle
- Android - ProgressBar
- Android - Push Notification
- Android - RenderScript
- Android - RSS Reader
- Android - Screen Cast
- Android - SDK Manager
- Android - Sensors
- Android - Session Management
- Android - Shared Preferences
- Android - SIP Protocol
- Android - Spelling Checker
- Android - SQLite Database
- Android - Support Library
- Android - Testing
- Android - Text to Speech
- Android - TextureView
- Android - Twitter Integration
- Android - UI Design
- Android - UI Patterns
- Android - UI Testing
- Android - WebView Layout
- Android - Wi-Fi
- Android - Widgets
- Android - XML Parsers
- Android Useful Resources
- Android - Questions and Answers
- Android - Useful Resources
- Android - Discussion
Android - Fragment Transition
What is a Transition?
Activity and Fragment transitions in Lollipop are built on top of a relatively new feature in Android called Transitions. Introduced in KitKat, the transition framework provides a convenient API for animating between different UI states in an application. The framework is built around two key concepts: scenes and transitions. A scene defines a given state of an application's UI, whereas a transition defines the animated change between two scenes.
When a scene changes, a Transition has two main responsibilities −
- Capture the state of each view in both the start and end scenes.
- Create an Animator based on the differences that will animate the views from one scene to the other.
Example
This example will explain you how to create your custom animation with fragment transition . So let's follow the following steps to similar to what we followed while creating Hello World Example −
Step | Description |
---|---|
1 | You will use Android Studio to create an Android application and name it as fragmentcustomanimations under a package com.example.fragmentcustomanimations, with blank Activity. |
2 | Modify the activity_main.xml, which has placed at res/layout/activity_main.xml to add a Text View |
3 | Create a layout called fragment_stack.xml.xml under the directory res/layout to define your fragment tag and button tag |
4 | Create a folder, which is placed at res/ and name it as animation and add fragment_slide_right_enter.xml fragment_slide_left_exit.xml, ,fragment_slide_right_exit.xml and fragment_slide_left_enter.xml |
5 | In MainActivity.java, need to add fragment stack, fragment manager, and onCreateView() |
6 | Run the application to launch Android emulator and verify the result of the changes done in the application. |
following will be the content of res.layout/activity_main.xml it contained TextView
<?xml version="1.0" encoding="utf-8"?> <TextView xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/text" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center_vertical|center_horizontal" android:text="@string/hello_world" android:textAppearance="?android:attr/textAppearanceMedium" />
Following will be the content of res/animation/fragment_stack.xml file. it contained frame layout and button
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <fragment android:id="@+id/fragment1" android:name="com.pavan.listfragmentdemo.MyListFragment" android:layout_width="match_parent" android:layout_height="match_parent" /> </LinearLayout>
Following will be the content of res/animation/fragment_slide_left_enter.xml file. it contained set method and object animator
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <objectAnimator android:interpolator="@android:interpolator/decelerate_quint" android:valueFrom="100dp" android:valueTo="0dp" android:valueType="floatType" android:propertyName="translationX" android:duration="@android:integer/config_mediumAnimTime" /> <objectAnimator android:interpolator="@android:interpolator/decelerate_quint" android:valueFrom="0.0" android:valueTo="1.0" android:valueType="floatType" android:propertyName="alpha" android:duration="@android:integer/config_mediumAnimTime" /> </set>
following will be the content of res/animation/fragment_slide_left_exit.xml file.it contained set and object animator tags.
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <objectAnimator android:interpolator="@android:interpolator/decelerate_quint" android:valueFrom="0dp" android:valueTo="-100dp" android:valueType="floatType" android:propertyName="translationX" android:duration="@android:integer/config_mediumAnimTime" /> <objectAnimator android:interpolator="@android:interpolator/decelerate_quint" android:valueFrom="1.0" android:valueTo="0.0" android:valueType="floatType" android:propertyName="alpha" android:duration="@android:integer/config_mediumAnimTime" /> </set>
Following code will be the content of res/animation/fragment_slide_right_enter.xmlfile.it contained set and object animator tags
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <objectAnimator android:interpolator="@android:interpolator/decelerate_quint" android:valueFrom="-100dp" android:valueTo="0dp" android:valueType="floatType" android:propertyName="translationX" android:duration="@android:integer/config_mediumAnimTime" /> <objectAnimator android:interpolator="@android:interpolator/decelerate_quint" android:valueFrom="0.0" android:valueTo="1.0" android:valueType="floatType" android:propertyName="alpha" android:duration="@android:integer/config_mediumAnimTime" /> </set>
following code will be the content of res/animation/fragment_slide_right_exit.xmlfile, it contained set and object animator tags
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <objectAnimator android:interpolator="@android:interpolator/decelerate_quint" android:valueFrom="0dp" android:valueTo="100dp" android:valueType="floatType" android:propertyName="translationX" android:duration="@android:integer/config_mediumAnimTime" /> <objectAnimator android:interpolator="@android:interpolator/decelerate_quint" android:valueFrom="1.0" android:valueTo="0.0" android:valueType="floatType" android:propertyName="alpha" android:duration="@android:integer/config_mediumAnimTime" /> </set>
following code will be the content of src/main/java/MainActivity.java file. it contained button listener, stack fragment and onCreateView
package com.example.fragmentcustomanimations; import android.app.Activity; import android.app.Fragment; import android.app.FragmentTransaction; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.View.OnClickListener; import android.view.ViewGroup; import android.widget.Button; import android.widget.TextView; /** * Demonstrates the use of custom animations in a FragmentTransaction when * pushing and popping a stack. */ public class FragmentCustomAnimations extends Activity { int mStackLevel = 1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.fragment_stack); // Watch for button clicks. Button button = (Button)findViewById(R.id.new_fragment); button.setOnClickListener(new OnClickListener() { public void onClick(View v) { addFragmentToStack(); } }); if (savedInstanceState == null) { // Do first time initialization -- add initial fragment. Fragment newFragment = CountingFragment.newInstance(mStackLevel); FragmentTransaction ft = getFragmentManager().beginTransaction(); ft.add(R.id.simple_fragment, newFragment).commit(); } else { mStackLevel = savedInstanceState.getInt("level"); } } @Override public void onSaveInstanceState(Bundle outState) { super.onSaveInstanceState(outState); outState.putInt("level", mStackLevel); } void addFragmentToStack() { mStackLevel++; // Instantiate a new fragment. Fragment newFragment = CountingFragment.newInstance(mStackLevel); // Add the fragment to the activity, pushing this transaction // on to the back stack. FragmentTransaction ft = getFragmentManager().beginTransaction(); ft.setCustomAnimations(R.animator.fragment_slide_left_enter, R.animator.fragment_slide_left_exit, R.animator.fragment_slide_right_enter, R.animator.fragment_slide_right_exit); ft.replace(R.id.simple_fragment, newFragment); ft.addToBackStack(null); ft.commit(); } public static class CountingFragment extends Fragment { int mNum; /** * Create a new instance of CountingFragment, providing "num" * as an argument. */ static CountingFragment newInstance(int num) { CountingFragment f = new CountingFragment(); // Supply num input as an argument. Bundle args = new Bundle(); args.putInt("num", num); f.setArguments(args); return f; } /** * When creating, retrieve this instance's number from its arguments. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); mNum = getArguments() != null ? getArguments().getInt("num") : 1; } /** * The Fragment's UI is just a simple text view showing its * instance number. */ @Override public View onCreateView(LayoutInflater inflater, ViewGroup container,Bundle savedInstanceState) { View v = inflater.inflate(R.layout.hello_world, container, false); View tv = v.findViewById(R.id.text); ((TextView)tv).setText("Fragment #" + mNum); tv.setBackgroundDrawable(getResources(). getDrawable(android.R.drawable.gallery_thumb)); return v; } } }
following will be the content of AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.fragmentcustomanimations" android:versionCode="1" android:versionName="1.0" > <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.example.fragmentcustomanimations.MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Running the Application
Let's try to run our Fragment Transitions application we just created. I assume you had created your AVD while doing environment set-up. To run the app from Android Studio, open one of your project's activity files and click Run icon from the toolbar. Android installs the app on your AVD and starts it and if everything is fine with your setup and application, it will display following Emulator window:
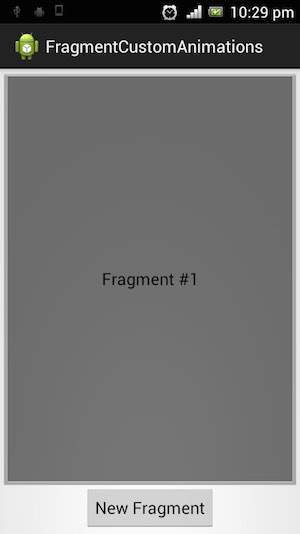
If click on new fragment, it going to be changed the first fragment to second fragment as shown below
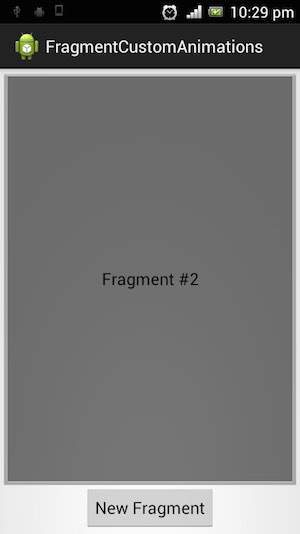